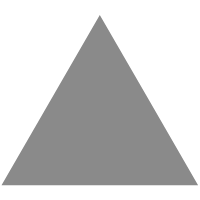
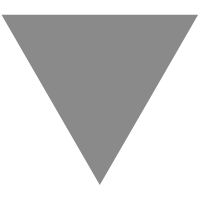
Vue.js 学习笔记之五:编译 vue 组件
source link: http://www.cnblogs.com/owlman/p/13835784.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
正如上一篇笔记中所说,直接使用 ES6 标准提供的模块规范来编写 Vue 组件在很多情况下可能并不是最佳实践。主要原因有两个,首先是市面上还有许多并没有对 ES6 标准提供完全支持的 Web 浏览器,这样做可能会导致某些用户无法使用应用程序。其次,即使将来所有的 Web 浏览器都完全支持了 ES6 标准规范,直接在 JavaScript 原生的字符串对象中编写 HTML 模版的做法也会让我们的编程工具无法对其进行高亮显示与语法检查,这不仅会让编程体验大打折扣,也会增加编码的出错概率。为了解决这个问题,Vue 社区专门定义了一种编写 Vue 组件的文件格式,例如对于 component_2
中的 sayHello.js
模块文件,我们可以将其重写为一个名为 sayHello.vue
的 Vue 组件文件,具体内容如 下:
<template> <div class="box"> <h1>你好, {{ you }}!</h1> <input type="text" v-model="you" /> </div> </template> <script> const sayHello = { name: 'sayHello', props : ['who'], data : function() { return { you: this.who } } }; export default sayHello; </script> <style scoped> .box { height: 150px; width: 250px; background-color: #ccc; } </style>
如你所见,这个专用文件实际上是一个 XML 格式的文件,它主要由三个标签组成:首先是 <template>
标签,用于定义组件的 HTML 模版,其作用就相当于之前在 sayHello.js
中定义的 tpl
字符串对象,区别在于该标签中的内容会被自动关联到组件对象的 template
模版属性上。接下来是 <script>
标签,用于定义组件对象本身,这部分代码与之前 sayHello.js
文件中的内容基本相同,只是无需再手动定义组件的 template
值了。最后是 <style>
标签,用于定义组件的 CSS 样式,当然了,这部分是可以省略的,如果没有样式可以定义就不必写。
在了解了专用于定义 vue 组件的文件格式之后,我们接下来要面对的一个问题是,JavaScript 解释器本身并不认识这种格式的文件,所以接下来的工作是要用 babel 和 webpack 这些工具将其编译成普通的 JavaScript 代码文件,现在就通过第三个实验记录一下这部分的工作,其主要步骤如下:
-
在
code/00_test
目录中再创建一个名为component_3
的实验目录,并在该目录下执行npm init -y
命令将其初始化成一个 Node.js 项目。 -
在
component_3
实验目录执行npm install --save vue
命令将 Vue.js 框架安装到该实验项目中。 -
在
component_3
实验目录执行npm install --save-dev <组件名>
命令分别安装以下组件:- webpack、webpack-cli: 用于构建项目的专用工具。
- babel、babel-core、babel-loader:用于将文件内容转译成普通的 JavaScript 代码。
- html-webpack-plugin:用于处理 HTML 文档的 webpack 插件。
- vue-loader、vue-template-compiler:用于处理 vue 组件文件格式的 webpack 插件。
- css-loader、vue-style-loader:用于处理 CSS 样式的 webpack 插件。
请注意,以上组件的版本必须与当前使用的 Node.js 运行环境的版本相匹配,否则在后续工作中会遇到各种意想不到的麻烦。
-
在
component_3
实验目录下创建一个名为src
的目录,用于存放将要被编译的源代码。 -
将之前创建的
sayHello.vue
保存在src
原代码目录中,并在该目录下创建以下文件:-
index.htm
文件:<!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>学习 vue 组件实验(3):以专用文件格式注册组件</title> </head> <body> <div id="app"> <say-hello :who="who"></say-hello> </div> </body> </html>
-
main.js
文件:import Vue from 'vue'; import sayHello from './sayHello.vue'; new Vue({ el: '#app', components: { 'say-hello': sayHello }, data: { who:'vue' } });
-
-
在
component_3
实验目录下创建一个名为webpack.config.js
的 webpack 配置文件,并在其中输入如下代码:const path = require('path'); const VueLoaderPlugin = require('vue-loader/lib/plugin'); const HtmlWebpackPlugin = require('html-webpack-plugin'); const config = { entry: { main: path.join(__dirname,'src/main.js') }, output: { path: path.resolve(__dirname,'./public/'), filename:'js/[name]-bundle.js' }, plugins:[ new VueLoaderPlugin(), new HtmlWebpackPlugin({ template: path.join(__dirname, 'src/index.htm') }) ], module: { rules: [ { test: /\.vue$/, loader: 'vue-loader' }, { test: /\.js$/, loader: 'babel-loader' }, { test: /\.css/, use: [ 'vue-style-loader', 'css-loader' ] } ] }, resolve: { alias: { 'vue$': 'vue/dist/vue.esm.js' } } }; module.exports = config;
-
在
component_3
实验目录下将package.json
文件中的script
项修改如下:"scripts": { "test": "echo \"Error: no test specified\" && exit 1", "build": "rm -rf ./public/* && node_modules/.bin/webpack" }
-
在
component_3
实验目录下创建一个名为public
的目录,用于存放编译结果。 -
在
component_3
实验目录下执行npm run build
命令,就可以在public
目录下看到编译结果了。 -
用浏览器访问
public
目录下的index.html
文件,就可以看到最后的结果了,如下图所示:
当然了,webpack 的配置工作是一个非常复杂和繁琐的过程,你在这里看到的只是沧海一粟,更复杂的内容请参考 webpack 的官方文档 。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK