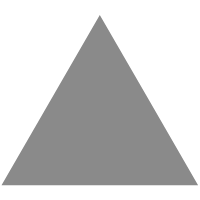
39
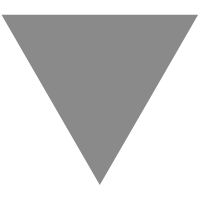
C++11新特性-std::tuple
source link: http://www.banbeichadexiaojiubei.com/index.php/2020/10/05/c11新特性-stdtuple/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
1. 引入头文件
#include <tuple>
2. std::tuple初始化
std::tuple<int, std::string, float> t1(10, "Test", 3.14);
这里要注意,不是所有的C++ 11编译器都支持copy-list-initialization的方式。如下代码所示。
std::tuple<int, int> foo_tuple() { return {1, -1}; // Error until N4387 return std::tuple<int, int>{1, -1}; // Always works return std::make_tuple(1, -1); // Always works }
3. 打印std::tuple
打印std::tuple可以将它的元素逐个打印出来,不过非常繁琐,我们可以通过如下通用的打印函数,帮助我们一次性的将tuple的所有要素打印出来。
#include <iostream> #include <tuple> #include <string> // helper function to print a tuple of any size template<class Tuple, std::size_t N> struct TuplePrinter { static void print(const Tuple& t) { TuplePrinter<Tuple, N-1>::print(t); std::cout << ", " << std::get<N-1>(t); } }; template<class Tuple> struct TuplePrinter<Tuple, 1> { static void print(const Tuple& t) { std::cout << std::get<0>(t); } }; template<typename... Args, std::enable_if_t<sizeof...(Args) == 0, int> = 0> void print(const std::tuple<Args...>& t) { std::cout << "()\n"; } template<typename... Args, std::enable_if_t<sizeof...(Args) != 0, int> = 0> void print(const std::tuple<Args...>& t) { std::cout << "("; TuplePrinter<decltype(t), sizeof...(Args)>::print(t); std::cout << ")\n"; } // end helper function int main() { std::tuple<int, std::string, float> t1(10, "Test", 3.14); print(t1); }
输出:
(10, Test, 3.14)
4、合并多个std::tuple
std::tuple_cat函数可以将多个std::tuple合并为一个tuple。
int main() { std::tuple<int, std::string, float> t1(10, "Test", 3.14); int n = 7; auto t2 = std::tuple_cat(t1, std::make_tuple("Foo", "bar"), t1, std::tie(n)); n = 42; print(t2); }
输出:
(10, Test, 3.14, Foo, bar, 10, Test, 3.14, 42)
5. std::tuple的解包(unpack)
std::tie能够将std::tuple包含的要素解包(unpack)成单个的对象。
#include <iostream> #include <tuple> #include <string> int main() { auto info = std::make_tuple(3.8, 'A', "Lisa Simpson"); double score = 0.0; char grade; std::string name; std::tie(score, grade, name) = info; std::cout << "score:" << score << ", grade:" << grade << ", name:" << name << std::endl; return 0; }
输出:
score:3.8, grade:A, name:Lisa Simpson
std::tie还支持std::pair对象的解包(unpack)。
#include <iostream> #include <tuple> #include <string> #include <utility> int main() { auto info = std::make_pair(3.8, "Lisa Simpson"); double score = 0.0; std::string name; std::tie(score, name) = info; std::cout << "score:" << score << ", name:" << name << std::endl; return 0; }
输出:
score:3.8, name:Lisa Simpson
当我们不关注tuple中的某个元素时,可以使用std::ignore忽略该元素。
#include <iostream> #include <tuple> #include <string> #include <utility> int main() { auto info = std::make_pair(3.8, "Lisa Simpson"); double score = 0.0; std::string name; std::tie(score, std::ignore) = info; std::cout << "score:" << score << ", name:" << name << std::endl; return 0; }
输出:
score:3.8, name:
参考材料
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK