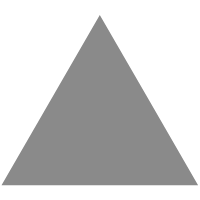
22
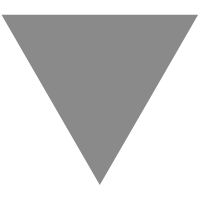
go发送smtp邮件
source link: https://segmentfault.com/a/1190000025146124
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
最近看了下go发送smtp邮件,于是总结一下
简单示例
- 先上一个最简单的代码 (网上搂的代码改了改)
package main import ( "fmt" "net/smtp" ) const ( // 邮件服务器地址 SMTP_MAIL_HOST = "smtp.qq.com" // 端口 SMTP_MAIL_PORT = "587" // 发送邮件用户账号 SMTP_MAIL_USER = "[email protected]" // 授权密码 SMTP_MAIL_PWD = "xxxx" // 发送邮件昵称 SMTP_MAIL_NICKNAME = "lewis" ) func main() { //声明err, subject,body类型,并为address,auth以及contentType赋值, //subeject是主题,body是邮件内容, address是收件人 var err error var subject, body string address := "[email protected]" auth := smtp.PlainAuth("", SMTP_MAIL_USER, SMTP_MAIL_PWD, SMTP_MAIL_HOST) contentType := "Content-Type: text/html; charset=UTF-8" //要发送的消息,可以直接写在[]bytes里,但是看着太乱,因此使用格式化 s := fmt.Sprintf("To:%s\r\nFrom:%s<%s>\r\nSubject:%s\r\n%s\r\n\r\n%s", address, SMTP_MAIL_NICKNAME, SMTP_MAIL_USER, subject, contentType, body) msg := []byte(s) //邮件服务地址格式是"host:port",因此把addr格式化为这个格式,直接赋值也行。 addr := fmt.Sprintf("%s:%s", SMTP_MAIL_HOST, SMTP_MAIL_PORT) //发送邮件 err = smtp.SendMail(addr, auth, SMTP_MAIL_USER, []string{address}, msg) if err != nil { fmt.Println(err) } else { fmt.Println("send email succeed") } }
收到邮件截图
简要说明
- const中SMTP_MAIL_PWD 是我申请开通qq邮箱的smtp服务时,腾讯发来的密码,并不是qq邮箱的密码。
- 可以看到邮件截图中显示(无主题),邮件也没内容,因为subeject和body我只声明了,但并未赋值,因此这俩为空。
- contentType设为text/html,当然也有其他格式。
- 其余的注释里应该说的比较清楚了
稍加改进
-
我们来加点常用的功能
- 收件人有多个
- 当然还得设置主题和邮件内容
- 发邮件功能单独封装为一个函数,然后main里调用
package main import ( "fmt" "net/smtp" ) const ( // 邮件服务器地址 SMTP_MAIL_HOST = "smtp.qq.com" // 端口 SMTP_MAIL_PORT = "587" // 发送邮件用户账号 SMTP_MAIL_USER = "[email protected]" // 授权密码 SMTP_MAIL_PWD = "jhguvicvhrnwgaeh" // 发送邮件昵称 SMTP_MAIL_NICKNAME = "lewis" ) func main() { address := []string{"[email protected]", "[email protected]"} subject := "test mail" body := `<br>hello!</br> <br>this is a test email, pls ignore it.</br>` SendMail(address, subject, body) } func SendMail(address []string, subject, body string) (err error) { // 认证, content-type设置 auth := smtp.PlainAuth("", SMTP_MAIL_USER, SMTP_MAIL_PWD, SMTP_MAIL_HOST) contentType := "Content-Type: text/html; charset=UTF-8" // 因为收件人,即address有多个,所以需要遍历,挨个发送 for _, to := range address { s := fmt.Sprintf("To:%s\r\nFrom:%s<%s>\r\nSubject:%s\r\n%s\r\n\r\n%s", to, SMTP_MAIL_NICKNAME, SMTP_MAIL_USER, subject, contentType, body) msg := []byte(s) addr := fmt.Sprintf("%s:%s", SMTP_MAIL_HOST, SMTP_MAIL_PORT) err = smtp.SendMail(addr, auth, SMTP_MAIL_USER, []string{to}, msg) if err != nil { return err } } return err }
收到邮件截图
简要说明
- 收件人是两个,都收到了
- subject有了
- body使用了br标签换行,直接在``内化换行试了下不行
- 另外如果发邮件报错:550 Mailbox not found or access denied,百度会发现是:您要发送的收件人短时间内收到大量邮件,为避免受到恶意攻击,暂时禁止向该收件人发信,但其实收件人的地址不存在时也会报这个错误。
OK,暂时先到这里。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK