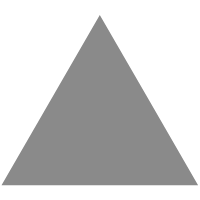
35
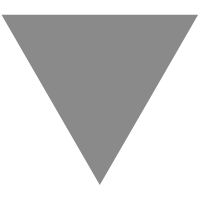
Spring Boot 整合 H2
source link: http://www.zhouzhaodong.xyz/archives/springboot整合h2
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
每当有项目需要访问数据库的时候总是很苦恼,因为无论哪个数据库都比较庞大,运行起来也比较耗内存,有没有简单可以随项目启动的数据库吗?有,H2来了。。。
1. 搭建Spirng Boot项目
如果启动出现异常,就将中文注释去掉再启动即可。
1.1 pom.xml
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
1.2 application.yml
server: port: 8888 # 端口号 spring: jpa: show-sql: true # 启用SQL语句的日志记录 hibernate: ddl-auto: update # 设置ddl模式 datasource: driver-class-name: org.h2.Driver # url: jdbc:h2:mem:h2 # 配置h2数据库连接地址(这里使用的是内存,下次启动数据就不存在了) url: jdbc:h2:file:./db/h2;AUTO_SERVER=TRUE # 配置h2数据库连接地址(这里使用的是本地存储方式,下次启动数据还存在) username: root # 配置数据库用户名 password: 123456 # 配置数据库密码 h2: console: path: /h2 # 进行该配置,你就可以通过YOUR_URL/h2访问h2 web consloe。YOUR_URL是你程序的访问URl enabled: true # 配置程序开启时就会启动h2 web consloe settings: web-allow-others: true # 配置h2的远程访问
1.3 新建User实体类
/** * 实体类 * @author zhouzhaodong */ @Entity @Table(name="user") public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Integer id; @Column private String name; @Column private String phone; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } }
1.4 新建UserDao类
/** * dao * @author zhouzhaodong */ public interface UserDao extends JpaRepository<User, Integer> { }
1.5 新建UserController控制层
/** * 控制层 * @author zhouzhaodong */ @RestController public class UserController { @Resource UserDao userDao; @RequestMapping("/findById") public Object findById(Integer id){ return userDao.findById(id); } @RequestMapping("/insert") public void insert(User user){ userDao.save(user); } @RequestMapping("/delete") public void delete(Integer id){ userDao.deleteById(id); } }
2. 测试
2.1 启动项目
会发现db持久化在项目内部了:

2.2 打开localhost:8888/h2
2.2.1 登录界面
2.2.2 填写完毕后点击连接:
2.2.3 新建User表
create table if not exists USER( ID INT NOT NULL PRIMARY KEY AUTO_INCREMENT, NAME VARCHAR(100), PHONE VARCHAR(100) );
2.3 访问接口进行数据操作
2.3.1 新增数据
2.3.2 查询数据
2.3.3 删除数据
GitHub代码地址:
https://github.com/zhouzhaodong/springboot/tree/master/spring-boot-h2
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK