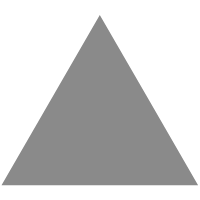
18
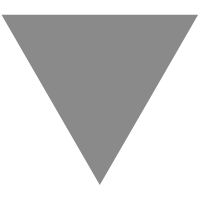
个人学习系列 - Spring Boot 集成 Swagger
source link: https://segmentfault.com/a/1190000024506469
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
恩,java后端开发的痛就是测试接口。以前都是启动项目用postman复制地址然后再添加参数,如果参数多的话真的是痛苦的经历,这里我要尝试一下Swagger了。。。
Swagger简介
Swagger 是一个规范和完整的框架,用于生成、描述、调用和可视化 RESTful 风格的 Web 服务。
1. 新建Spring Boot项目
1.1 pom.xml
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>com.battcn</groupId> <artifactId>swagger-spring-boot-starter</artifactId> <version>2.1.5-RELEASE</version> </dependency> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.3.0</version> </dependency>
1.2 application.yml
server: # 端口号 port: 8888 servlet: # 项目前缀 context-path: /swagger spring: swagger: enabled: true # 项目标题 title: spring-boot-swagger # 项目说明 description: 这是一个简单的 Swagger API 演示 # 版本号,自己定义 version: 1.0.0 contact: # 自己的信息 name: zhouzhaodong email: [email protected] url: http://www.zhouzhaodong.xyz # swagger扫描的基础包,默认:全扫描 # base-package: # 需要处理的基础URL规则,默认:/** # base-path: # 需要排除的URL规则,默认:空 # exclude-path: security: # 是否启用 swagger 登录验证 filter-plugin: true # 账号 username: zhouzhaodong # 密码 password: 123456 # 相关错误说明 global-response-messages: GET[0]: code: 400 message: Bad Request,这个错误一般情况下为请求参数不对 GET[1]: code: 404 message: NOT FOUND,这个错误一般情况下为请求路径不对 GET[2]: code: 500 message: ERROR,这个错误一般情况下为程序内部错误 POST[0]: code: 400 message: Bad Request,这个错误一般情况下为请求参数不对 POST[1]: code: 404 message: NOT FOUND,这个错误一般情况下为请求路径不对 POST[2]: code: 500 message: ERROR,这个错误一般情况下为程序内部错误
1.3 接口返回实体类
注解说明: @ApiModel: 用于响应实体类上,用于说明实体作用 参数: description="描述实体的作用" @ApiModelProperty: 用在属性上,描述实体类的属性 参数: value="用户名" 描述参数的意义 name="name" 参数的变量名 required=true 参数是否必选
/** * 接口返回实体类 * * @author zhouzhaodong */ @Data @Builder @NoArgsConstructor @AllArgsConstructor @ApiModel(value = "接口返回实体类", description = "Common Api Response") public class ResponseBody<T> implements Serializable { private static final long serialVersionUID = -8987146499044811408L; /** * 返回状态 */ @ApiModelProperty(value = "返回状态", required = true) private Integer code; /** * 返回信息 */ @ApiModelProperty(value = "返回信息", required = true) private String message; /** * 返回数据 */ @ApiModelProperty(value = "返回数据", required = true) private T data; }
1.4 新建Person实体类
注解说明: @ApiModel: 用于响应实体类上,用于说明实体作用 参数: description="描述实体的作用" @ApiModelProperty: 用在属性上,描述实体类的属性 参数: value="用户名" 描述参数的意义 name="name" 参数的变量名 required=true 参数是否必选
/** * 用户实体类 * * @author zhouzhaodong */ @Data @NoArgsConstructor @AllArgsConstructor @ApiModel(value = "用户实体", description = "User Entity") public class Person implements Serializable { private static final long serialVersionUID = 5057954049311281252L; /** * 主键id */ @ApiModelProperty(value = "主键id", required = true) private Integer id; /** * 用户名 */ @ApiModelProperty(value = "用户名", required = true) private String name; /** * 工作岗位 */ @ApiModelProperty(value = "工作岗位", required = true) private String job; }
1.5 新建controller
注解说明: @API: 放在请求的类上,与@Controller 并列,说明类的作用,如用户模块, 订单类等. 参数: tags="说明该类的作用,参数是个数组,可以填多个" value="该参数没什么意义,在UI界面上不显示,所以不用配置" description = "用户基本信息操作" @ApiOperation: 用在请求的方法上, 说明方法的作用。 参数: value="方法的用途和作用" notes="方法的注意事项和备注" tags:说明该方法的作用,参数是个数组,可以填多个。 格式:tags={"作用1","作用2"} @ApiImplicitParam:用于方法,表示单独的请求参数 参数: name="参数ming" value="参数说明" dataType="数据类型" paramType="query" 表示参数放在哪里 · header 请求参数的获取:@RequestHeader · query 请求参数的获取:@RequestParam · path (用于restful接口) 请求参数的获取:@PathVariable · body (不常用) · form (不常用) defaultValue="参数的默认值" required="true" 表示参数是否必须传 @ApiImplicitParams: 用在请求的方法上,包含多@ApiImplicitParam
/** * 控制器 * * @author zhouzhaodong */ @RestController @RequestMapping("/person") @Api(tags = "1.0.0-SNAPSHOT", description = "用户管理", value = "用户管理") @Slf4j public class PersonController { @GetMapping @ApiOperation(value = "条件查询", notes = "备注") @ApiImplicitParams({@ApiImplicitParam(name = "name", value = "用户名", dataType = DataType.STRING, paramType = ParamType.QUERY, defaultValue = "xxx")}) public ResponseBody<Person> getByPersonName(String name) { log.info("多个参数用 @ApiImplicitParams"); return ResponseBody.<Person>builder().code(200).message("操作成功").data(new Person(1, name, "JAVA")).build(); } @GetMapping("/{id}") @ApiOperation(value = "主键查询", notes = "备注") @ApiImplicitParams({@ApiImplicitParam(name = "id", value = "用户编号", dataType = DataType.INT, paramType = ParamType.PATH)}) public ResponseBody<Person> get(@PathVariable Integer id) { log.info("单个参数用 @ApiImplicitParam"); return ResponseBody.<Person>builder().code(200).message("操作成功").data(new Person(id, "u1", "p1")).build(); } @DeleteMapping("/{id}") @ApiOperation(value = "删除用户", notes = "备注") @ApiImplicitParam(name = "id", value = "用户编号", dataType = DataType.INT, paramType = ParamType.PATH) public void delete(@PathVariable Integer id) { log.info("单个参数用 ApiImplicitParam"); } @PostMapping @ApiOperation(value = "添加用户", notes = "备注") public Person post(@RequestBody Person person) { log.info("如果是 POST PUT 这种带 @RequestBody 的可以不用写 @ApiImplicitParam"); return person; } @PostMapping("/multipar") @ApiOperation(value = "添加用户", notes = "备注") public List<Person> multipar(@RequestBody List<Person> person) { log.info("如果是 POST PUT 这种带 @RequestBody 的可以不用写 @ApiImplicitParam"); return person; } @PostMapping("/array") @ApiOperation(value = "添加用户", notes = "备注") public Person[] array(@RequestBody Person[] person) { log.info("如果是 POST PUT 这种带 @RequestBody 的可以不用写 @ApiImplicitParam"); return person; } @PutMapping("/{id}") @ApiOperation(value = "修改用户", notes = "备注") public void put(@PathVariable Long id, @RequestBody Person person) { log.info("如果你不想写 @ApiImplicitParam 那么 swagger 也会使用默认的参数名作为描述信息 "); } }
2. 测试
2.1 打开 http://localhost :8888/swagger/swagger-ui.html
++注意:++
如果我们打开页面发现我们后台的汉字变成了乱码,需要设置IDEA的文件编码
2.2 登录
2.2.1所有接口
2.2.2 点击某个接口
2.2.3 点击在线调试
2.2.4 点击发送按钮
个人博客地址:
GitHub代码地址:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK