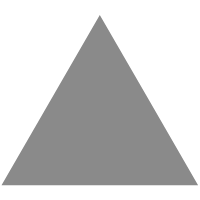
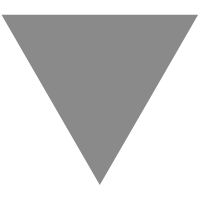
springboot~使用自定义的aspect
source link: http://www.cnblogs.com/lori/p/13437986.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
对某个类型中的方法进行拦截,然后加入固定的业务逻辑,这是AOP面向切面编程可以做的事,在springboot里实现aop的方法也有很多, spring-boot-starter-aop
或者 aspectjweaver
都是可以实现的,不过我们在实现之前,先来看一下aop里的几个概念。
概念
-
切面(Aspect):是指横切多个对象的关注点的一个模块化,事务管理就是J2EE应用中横切关注点的很好示例。在Spring AOP中,切面通过常规类(基本模式方法)或者通过使用了注解@Aspect的常规类来实现。
-
连接点(Joint point):是指在程序执行期间的一个点,比如某个方法的执行或者是某个异常的处理。在Spring AOP中,一个连接点往往代表的是一个方法执行。
-
通知(Advice):是指切面在某个特殊连接点上执行的动作。通知有不同类型,包括"around","before"和"after"通知。许多AOP框架包括Spring,将通知建模成一个拦截器,并且围绕连接点维持一个拦截器链。
-
切入点(Pointcut):是指匹配连接点的一个断言。通知是和一个切入点表达式关联的,并且在任何被切入点匹配的连接点上运行(举例,使用特定的名字执行某个方法)。AOP的核心就是切入点表达式匹配连接点的思想。Spring默认使用AspectJ切入点表达式语
-
引入(Introduction):代表了对一个类型额外的方法或者属性的声明。Spring AOP允许引入新接口到任何被通知对象(以及一个对应实现)。比如,可以使用一个引入去使一个bean实现IsModified接口,从而简化缓存机制。(在AspectJ社区中,一个引入也称为一个inter-type declaration类型间声明)
-
目标对象(Target object):是指被一个或多个切面通知的那个对象。也指被通知对象("advised object"),由于Spring AOP是通过运行时代理事项的,这个目标对象往往是一个代理对象。
-
AOP 代理(AOP proxy):是指通过AOP框架创建的对象,用来实现切面合约的(执行通知方法等等)。在Spring框架中,一个AOP代理是一个JDK动态代理或者是一个CGLIB代理。
-
织入(Weaving):将切面和其他应用类型或者对象连接起来,创骗一个被通知对象。这些可以在编译时(如使用AspectJ编译器)、加载时或者运行时完成。Spring AOP,比如其他纯Java AOP框架一般是在运行时完成织入。
实现
1 引用依赖包
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> </dependency>
2 添加切面和拦截的行为
@Aspect @Component @Slf4j public class TestAspect { /** * 对TestService类下面的所有方法拦截. */ @Pointcut("execution(* com.lind.start.test.aop.TestService.*(..))") public void pointcut() { } //前置通知 @Before("pointcut()") public void beforeMethod(JoinPoint joinPoint) { if (joinPoint.getArgs().length == 1 && joinPoint.getArgs()[0] instanceof User) { User user = (User) joinPoint.getArgs()[0]; user.setUsername("aop赋值"); log.info("调用了前置通知" + user.toString()); } } //@After: 后置通知 @After("pointcut()") public void afterMethod(JoinPoint joinPoint) { log.info("调用了后置通知"); } //@AfterRunning: 返回通知 result为返回内容 @AfterReturning(value = "pointcut()", returning = "result") public void afterReturningMethod(JoinPoint joinPoint, Object result) { log.info("调用了返回通知"); } //@Around:环绕通知 @Around("pointcut()") public Object Around(ProceedingJoinPoint pjp) throws Throwable { log.info("around执行方法之前"); Object object = pjp.proceed(); log.info("around执行方法之后--返回值:" + object); return object; } }
3 调用及结果
@SpringBootTest @RunWith(SpringRunner.class) public class AopTest { @Autowired TestService testService; @Test public void test() { testService.print(new User()); } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK