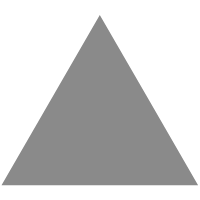
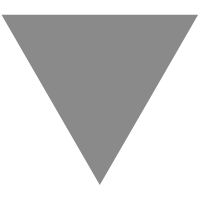
Genetic Algorithm Based Approach for Robotic Controllers
source link: https://towardsdatascience.com/genetic-algorithm-based-approach-for-robotic-controllers-3966a9b874fb?gi=ec2cb5e56846
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
implemented in Python
Jul 29 ·5min read
Today, we will solve a real-world problem is to design a robotic controller. There are many techniques that can be used to solve this problem. Some of them include genetic algorithm (GA), particle swarm optimization and neural network (NN).
What we need to do is to apply an algorithm to the robotic as a method of designing robotic controllers that enable the robot to perform complex tasks and behaviors.
Autonomous robot is a robot that can perform certain work independently without the human help.
One of the capabilities of robots is to move from one point to another which called is autonomous navigation. Imagine that we have built a robot that can move goods around a warehouse. In this article, we will implement this capability using the Python language. How the robot can see its local environment? Yes, we would install sensors, which allow the robot can look around and we have given it wheels so it can navigate based on input from its sensors. The biggest problem is how we can link the sensor data to motor actions so that the robot can navigate the warehouse.
In general, we frequently use neural networks to successfully map robotic sensors to outputs by using reinforcement learning algorithms to the learning process. But we will use another way today, it is to use genetic algorithms. Typically, a genetic algorithm will evaluate a large population of individuals to find the best individuals for the next generation by using a fitness function that compute the performance of and individual based on certain predefined rules.
However, we will be facing a new challenge, physically evaluating each robot controller is not feasible for a large population due to the difficulty in physically testing each robotic controller and the time it would take to do so. For this reason, we will use our knowledge of genetic algorithms to design and implement a robotic controller and apply it to a virtual robot in a virtual environment.
The target
Our robot can take four actions: move one step forward, turn left, turn righ and do nothing.
The robot also has six sensors in the figure below.
- three on the front
- one on the left
- one on the right
- one on the back
The maze is comprised of walls that the robot can’t cross and will have an outlined route. We are going to design a robotic controller that can use the robot sensors to navigate a robot successfully through a maze.
The Solution
The genetic algorithm pseudocode
The pseudo code for a basic genetic algorithm is as follows
generation = 0; population[generation] = initializePopulation(populationSize); evaluatePopulation(population[generation]); While isTerminationConditionMet() == false do parents = selectParents(population[generation]); population[generation+1] = crossover(parents); population[generation+1] = mutate(population[generation+1]); evaluatePopulation(population[generation]); generation++; End loop;
This pseudocode demonstrates the basic process of a genetic algorithm. Next steps, we will implement them in Python.
Mapping the sensor values to actions
As mentioned in the previous section, the robot will have four actions that can be represented in binary as follows:
- “00” — do nothing;
- “01” — move forward;
- “10” — turn left;
- and “11” — turn right.
We also have six different sensors. To simplify the representation we limit the measurements to binary encoding, that is, values less than a threshold indicate obstacle detection and larger than threshold indicate a clear path. 6 sensors are giving us 2⁶ = 64 possible combinations of sensor inputs. Since an action requires 2 bits, our controller requires 64*2 = 128 bits of storage to represent any possible input. Given that 128 bits are our requirement for representing instructions different combinations. But how should we organize the chromosome so we can encode and decode it?
We have a human readable list of inputs and outputs as follows:
- Sensor #1 (front): on
- Sensor #2 (front-left): off
- Sensor #3 (front-right): on
- Sensor #4 (left): off
- Sensor #5 (right): off
- Sensor #6 (back): off
We also have an instruction that is “turn left” with a value of 10 in binary. Our next is to take the six sensor values and encode those further.
000101 => 10
If we now convert the sensor values’ bit string to decimal, we get the following:
5 => 10
So, we can use the sensor’s decimal value as the position in the chromosome that represents a combination of sensor inputs as follows.
xx xx xx xx xx 10 xx xx xx xx (… 54 more pairs…)
As can be seen in the following figure, a combination of sensor values produces a binary output that describes how a typical chromosome can map the robot’s sensor values to actions.
This encoding scheme may seem obtuse at first and the chromosome is not human-readable but it has a couple of helpful properties.
- First, the chromosome can be manipulated as an array of bits which makes crossover, mutation, and other manipulations much easier.
- Secondly, every 128-bit value is a valid solution.
Tournament Selection
Implementation
Firstly, we need to create and initialize a maze to run the robot in ( world.py
file). The maze object we’ve created uses integers to represent different terrain types:
- 1 defines a wall;
- 2 is the starting position;
- 3 traces the best route through the maze;
- 4 is the goal position;
- and 0 is an empty position that the robot can travel over but isn’t on the route to the goal.
We write a constructor to create a new maze from a double int array and implement public methods to get the start position, score a route through the maze.
Individual
An individual is represented by a single chromosome composed of multiple genes.
Robot
Next, we need to create a Robot that can follow instructions and generate a route by executing those instructions.
Population
The population refers to a group of chromosomes.
Genetic Algorithm
We implement methods to calculate fitness, select individuals, crossover and do mutation.
Robotic Controller
Finally, we can write a class that actually executes the algorithm. Create another new file called main.py
as follows.
The output.
Easy, right?
References
[1] An Introduction to Genetic Algorithms
[2] https://github.com/housecricket/Genetic-Algorithm-Based-Approach-for-Robotic-Controllers
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK