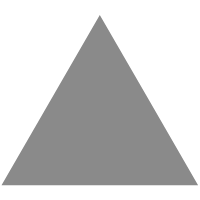
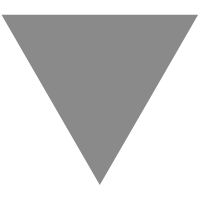
TypeScript 语法糖
source link: https://mp.weixin.qq.com/s/oBV06AZ2CDBft7MPSZz7ZQ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TypeScript 增加了代码的可读性和可维护性 。
前言
其实TS,已经用过一段时间,但是没有具体的整理过,虽然有官方文档,但是还是不如自己写一遍来的记忆深刻。
字符串的新特性
多行字符串
通过``来实现多行字符串。
const
str
=`You have pushed
the button this
many times:
`;
字符串模版
const
name=
'
haha
';
const
str
=`My name is ${name}
`;
自动拆分字符串
function
test(template, name, age) {
console.log(template);
console.log(name);
console.log(age);
}
const
Myname =
'haha'
;
const
getaAge = function () {
return 18;
}
test
`hello,my name is
${
Myname
}
,I'm
${
getaAge()
}
`
;
template会被解析成通过表达式分割的字符串模版数组,后面的参数是相应变量的值。
参数类型
声明变量中指定类型;
声明函数时指定类型;
函数参数也可以指定类型;
const
Myname =
'haha'
;
const
getaAge = function () {
return 18;
}
let
myname
: string
=
'haha'
;
let
age
:
number
= 18;
let
flag
: boolean
= true;
let
proType
: any
//any 可以是任何类型 = 22;
function
test(name
:string
)
: void
{
//表明test方法不需要任何返回值
}
class
Persion{
name
: string
;
age
: number
;
}
let
P1 =
new
Persion();
//会有语法提示 P1.age = 18;
指定参数默认值
设定参数默认值的参数要写在必填参数后面;
同样可选参数是不能声明在必填参数的后面;
function
test(a
:string
,b?
:number
,c
:boolean
=true) {
console.log(a);
console.log(b);
console.log(c);
}
test('haha',17)
Rest and Spread 操作符
用来声明任意数量的方法参数;
利用ES6的扩展运算符;
function
test(...args) {
args.forEach((arg) => {
console.log(arg)
})
}
test('haha', 17)
function
test1(a, b, c) {
console.log(a, b, c);
}
let
arry = [1, 2];
//1,2,undefined test1(...arry);
Destructuring析构表达式
通过表达式将数组和对象拆解成任意数量的变量;
function
getProps() {
return {
myname: 'haha',
ageObj: {
Tom: 12,
Jerry:19
}
}
}
let
{
myname: proname,
ageObj: { Tom }
} = getProps();
//haha console.log(proname);
//12 console.log(Tom);
let
arry = [1, 2, 3, 4, 5];
let
[one, two, ...others] = arry;
//1 console.log(one);
//2 console.log(two);
//[3,4,5] console.log(others);
类
在类的内部,有三种访问控制符:
public 控制的属性,可以在类的内部和外部访问到,是默认的访问控制符;
private 控制的属性,只可以在类的内部被访问;
protected 控制的属性,可以在类的内部和子类的被访问;
class
Persion{
constructor
(name
:string
) {
console.log(`parent ${name}`)
this
.name = name;
}
public
name;
protected
//可以在类的内部和子类被访问到 age;
eat() {
console.log(`eat ${
this
.name}`);
}
}
class
P1
extends
Persion {
constructor
(name
:string
,code
:string
) {
super
(name);
this
.code = code;
}
code;
work() {
super.eat();
console.log(
this
.code)
this
.doWork()
}
private
//私有方法,不会被外部调用 doWork() {
console.log("I'm do work");
}
}
const
persion1 =
new
P1('Tom', "2329323");
persion1.work();
类的继承有extend和super关键字来控制。
泛型(generic)
参数化类型,一般用来限制集合的内容。
class
Persion{
...
}
let
list: Array<Persion> = [];
//用类似指定数组只能放一类型元素,不能放其他类型
接口(Interface)
用来建立某种代码约定,使得其他开发者在调用某个方法或创建新的类时必须遵循接口所定义的代码约定。
Interface 关键字是声明一个接口;
implements 关键字是声明一个类去实现一个接口的方法;
interface
Ipersion{
name: string,
age:number
}
class
Persion{
constructor(public config:Ipersion) {
}
}
l
et
P1 =
new
Persion({
name: 'Tom',
age:12
//通过接口声明一些属性; });
interface
//声明一些方法 Animal {
eat()
}
class
sheep
implements
Animal {
eat() {
console.log('eat grass')
}
}
class
Tiger implements Animal {
eat() {
console.log('eat meat')
}
}
模块(Module)
模块可以帮助开发者将代码分割为可重用的单元。开发者可以自己决定将模块中的哪些资源(类、方法、变量)暴露出去供外部使用,哪些资源只在模块内使用。
export 用来控制模块对外暴露什么东西;
import 用来引入其他模块暴露东西;
注解(annotation)
注解为程序的元素(类、方法、变量)加上更直观更明了的说明。这些说明信息与程序的业务逻辑无关,而是供指定的工具或框架使用的。
import
{ Component }
from
'@angular/core'
;
@Component
({
selector:
'app-root'
,
templateUrl:
'./app.component.html'
,
styleUrls:[
'./app.component.css'
]
})
export
class
AppComponent {
title =
'app works'
;
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK