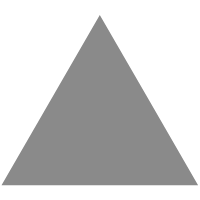
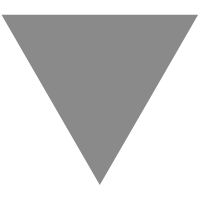
GitHub - michaelbull/kotlin-coroutines-jdbc: A library for interacting with bloc...
source link: https://github.com/michaelbull/kotlin-coroutines-jdbc
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
kotlin-coroutines-jdbc
A library for interacting with blocking JDBC drivers using Kotlin Coroutines.
Use of this library allows you to offload blocking JDBC calls to a dedicated
CoroutineDispatcher
(e.g.
Dispatchers.IO
), thus suspending your coroutine and freeing
your thread for other work while waiting.
Installation
repositories { mavenCentral() } dependencies { implementation("com.michael-bull.kotlin-coroutines-jdbc:kotlin-coroutines-jdbc:1.0.0") }
Introduction
The primary higher-order function exposed by the library is the
transaction
function.
suspend inline fun <T> transaction(crossinline block: suspend () -> T): T
Calling this function with a specific suspending block will run the block in
the context of a CoroutineTransaction
.
Calls to transaction
can be nested inside another, with each child re-using
the first CoroutineTransaction
. Only the outermost call will either
commit
or rollback
the
transaction.
Starting a fresh transaction will add a
CoroutineTransaction
to the current
CoroutineContext
. Transactions cannot be re-used after
completion and attempting to do so will result in a runtime failure.
A transaction will establish a new Connection
if an open one
does not already exist in the active CoroutineContext
.
If the transaction does establish a new Connection
, it will
attempt to close
it upon completion.
An active CoroutineConnection
is accessible from the
current CoroutineContext
. The connection from the context
can be used to prepare statements.
Example
import com.github.michaelbull.jdbc.context.CoroutineDataSource import com.github.michaelbull.jdbc.context.connection import com.github.michaelbull.jdbc.transaction import kotlinx.coroutines.CoroutineScope import kotlinx.coroutines.Dispatchers import kotlinx.coroutines.launch import javax.sql.DataSource import kotlin.coroutines.coroutineContext class Example(dataSource: DataSource) { private val scope = CoroutineScope(Dispatchers.IO + CoroutineDataSource(dataSource)) private val customers = CustomerRepository() fun query() { scope.launchTransaction() } private fun CoroutineScope.launchTransaction() = launch { val customers = addThenFindAllCustomers() customers.forEach(::println) } private suspend fun addThenFindAllCustomers(): List<String> { return transaction { customers.add("John Doe") customers.findAll() } } } class CustomerRepository { suspend fun add(name: String) { coroutineContext.connection.prepareStatement("INSERT INTO customers VALUES (?)").use { stmt -> stmt.setString(1, name) stmt.executeUpdate() } } suspend fun findAll(): List<String> { val customers = mutableListOf<String>() coroutineContext.connection.prepareStatement("SELECT name FROM customers").use { stmt -> stmt.executeQuery().use { rs -> while (rs.next()) { customers += rs.getString("name") } } } return customers } }
Further Reading
Contributing
Bug reports and pull requests are welcome on GitHub.
License
This project is available under the terms of the ISC license. See the
LICENSE
file for the copyright information and licensing terms.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK