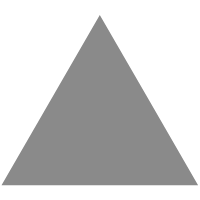
11
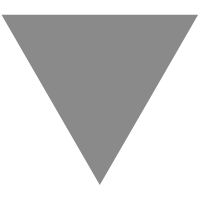
设计模式 - 数据访问对象模式
source link: http://violetzijing.is-programmer.com/posts/215462.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
数据访问对象模式(Data Access Object Pattern)或 DAO 模式用于把低级的数据访问 API 或操作从高级的业务服务中分离出来。以下是数据访问对象模式的参与者。
- 数据访问对象接口(Data Access Object Interface) - 该接口定义了在一个模型对象上要执行的标准操作。
- 数据访问对象实体类(Data Access Object concrete class) - 该类实现了上述的接口。该类负责从数据源获取数据,数据源可以是数据库,也可以是 xml,或者是其他的存储机制。
- 模型对象/数值对象(Model Object/Value Object) - 该对象是简单的 POJO,包含了 get/set 方法来存储通过使用 DAO 类检索到的数据。
package main import "fmt" type Student struct { Name string RollNo int } func (s *Student) GetName() string { return s.Name } func (s *Student) SetName(name string) { s.Name = name } func (s *Student) GetRollNo() int { return s.RollNo } func (s *Student) SetRollNo(no int) { s.RollNo = no } type StudentDAO interface { GetAllStudents() []*Student GetStudent(int) *Student UpdateStudent(*Student) } type StudentDAOImpl struct { Students []*Student } func NewStudentDAOImpl() *StudentDAOImpl { return &StudentDAOImpl{ Students: []*Student{ &Student{Name: "Robert", RollNo: 1}, &Student{Name: "John", RollNo: 2}, }, } } func (s *StudentDAOImpl) GetAllStudents() []*Student { return s.Students } func (s *StudentDAOImpl) GetStudent(no int) *Student { for _, s := range s.Students { if s.RollNo == no { return s } } return nil } func (s *StudentDAOImpl) UpdateStudent(student *Student) { for _, s := range s.Students { if s.RollNo == student.RollNo { s.SetName(student.Name) fmt.Println("Student: roll no", student.RollNo, "updated in the database") } } } func main() { var dao StudentDAO dao = NewStudentDAOImpl() for _, s := range dao.GetAllStudents() { fmt.Println("Student: ", s.Name, "with roll no", s.RollNo) } student := dao.GetStudent(dao.GetAllStudents()[0].RollNo) student.SetName("Michael") dao.UpdateStudent(student) fmt.Println(dao.GetStudent(student.RollNo)) }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK