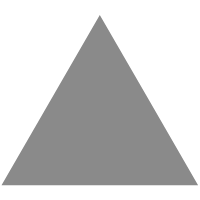
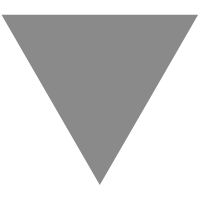
设计模式 - Flyweight Pattern
source link: http://violetzijing.is-programmer.com/posts/215444.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
意图: 运用共享技术有效地支持大量细粒度的对象。
主要解决: 在有大量对象时,有可能会造成内存溢出,我们把其中共同的部分抽象出来,如果有相同的业务请求,直接返回在内存中已有的对象,避免重新创建。
何时使用: 1、系统中有大量对象。 2、这些对象消耗大量内存。 3、这些对象的状态大部分可以外部化。 4、这些对象可以按照内蕴状态分为很多组,当把外蕴对象从对象中剔除出来时,每一组对象都可以用一个对象来代替。 5、系统不依赖于这些对象身份,这些对象是不可分辨的。
如何解决: 用唯一标识码判断,如果在内存中有,则返回这个唯一标识码所标识的对象。
关键代码: 用 HashMap 存储这些对象。
应用实例: 1、JAVA 中的 String,如果有则返回,如果没有则创建一个字符串保存在字符串缓存池里面。 2、数据库的数据池。
优点: 大大减少对象的创建,降低系统的内存,使效率提高。
缺点: 提高了系统的复杂度,需要分离出外部状态和内部状态,而且外部状态具有固有化的性质,不应该随着内部状态的变化而变化,否则会造成系统的混乱。
使用场景: 1、系统有大量相似对象。 2、需要缓冲池的场景。
package main import "fmt" type Shape interface { Draw() } type Circle struct { Color string X, Y, Radius int } func (c *Circle) SetX(x int) { c.X = x } func (c *Circle) SetY(y int) { c.Y = y } func (c *Circle) SetRadius(radius int) { c.Radius = radius } func (c *Circle) Draw() { fmt.Println("Draw circle, color: ", c.Color, ", x, y, radius: ", c.X, c.Y, c.Radius) } type ShapeFactory struct { Hash map[string]Shape } func (s *ShapeFactory) GetCircle(color string) *Circle { if v, ok := s.Hash[color]; ok { return v.(*Circle) } circle := &Circle{Color: color} s.Hash[color] = circle fmt.Println("Creating circle: ", circle) return circle } func main() { colors := []string{"red", "green", "blue", "white", "black"} factory := &ShapeFactory{Hash: map[string]Shape{}} for i := 0; i < 5; i++ { factory.GetCircle(colors[i]) } for _, v := range factory.Hash { fmt.Println("v: ", v) } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK