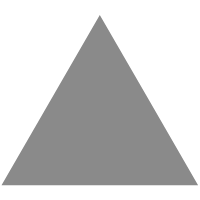
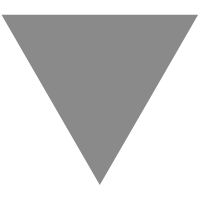
使用.Net Core实现的一个图形验证码
source link: http://www.cnblogs.com/jiangbingyang/p/13238190.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
SimpleCaptcha是一个使用简单,基于.Net Standard 2.0的图形验证码模块。它的灵感来源于Edi.Wang的这篇文章https://edi.wang/post/2018/10/13/generate-captcha-code-aspnet-core,我将其中生成验证码的代码抽取出来进行封装得到了这个模块。下面介绍一下使用方式。
基本使用方式
安装SimpleCaptcha
在Nuget中搜索安装SimpleCaptcha
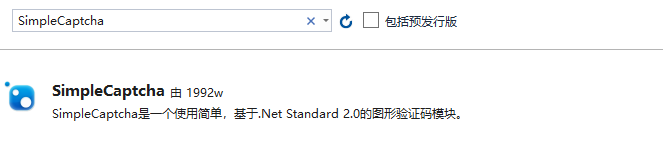
安装缓存模块
SimpleCaptcha依赖Microsoft.Extensions.Caching.Abstractions模块用来存储验证码,所以你需要在项目中根据自己的需要安装相应的实现包,例如这里我使用 Microsoft.Extensions.Caching.Memory
Startup
修改Startup.cs文件注入相应的服务:
services.AddMemoryCache() .AddSimpleCaptcha(builder => { builder.UseMemoryStore(); });
注入ICaptcha接口
在Controller中注入核心接口ICaptcha
private readonly ICaptcha _captcha; public HomeController(ICaptcha captcha) { _captcha = captcha; }
生成验证码
使用ICaptcha接口的 Generate
方法生成验证码
public IActionResult Captcha(string id) { var info = _captcha.Generate(id); var stream = new MemoryStream(info.CaptchaByteData); return File(stream, "image/png"); }
验证
使用ICaptcha接口的 Validate
方法对用户的提交进行验证
public IActionResult Validate(string id, string code) { var result = _captcha.Validate(id, code); return Json(new { success = result }); }
完整的例子可以在这里找到: https://github.com/1992w/SimpleCaptcha/tree/master/src/SimpleCaptcha.Demo
配置
SimpleCaptcha预留了一些默认的配置项,你可以根据需要自行修改。
设置验证码长度
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.CodeLength = 6; }); });
设置图片大小
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.ImageWidth = 100; options.ImageHeight = 36; }); });
设置区分大小写
默认情况下验证不区分大小写
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.IgnoreCase = false; }); });
设置验证码有效期
验证码默认的有效期为5分钟
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.ExpiryTime =TimeSpan.FromMinutes(10); }); });
设置字符集
SimpleCaptcha提供了 ICaptchaCodeGenerator
接口用来生成字符,默认的实现是从字符集 012346789ABCDEFGHIJKLMNOPQRSTUVWXYZ
中随机生成,你可以继承ICaptchaCodeGenerator接口实现自己的需求。
public class MyCaptchaCodeGenerator : ICaptchaCodeGenerator { public string Generate(int length) { throw new NotImplementedException(); } }
配置自己的生成器
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.CodeGenerator = new MyCaptchaCodeGenerator(); }); });
设置个性化的图片
如果默认生成的图片你觉得不符合你的要求,你可以实现 ICaptchaImageGenerator
接口进行修改
public class CaptchaImageGenerator : ICaptchaImageGenerator { public byte[] Generate(int width, int height, string captchaCode) { throw new NotImplementedException(); } }
services.AddSimpleCaptcha(builder => { builder.AddConfiguration(options => { options.ImageGenerator = new CaptchaImageGenerator(); }); });
源代码
所有源代码可以在这里获取: https://github.com/1992w/SimpleCaptcha
感谢
在这里感谢 Edi.Wang 分享。
最后
欢迎你对这个模块提出任何的建议和想法。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK