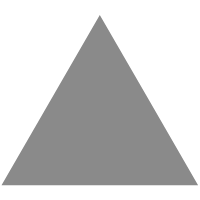
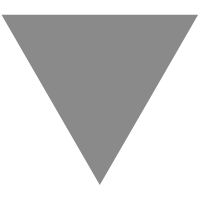
Standalone UUID generator in Javascript
source link: https://abhishekdutta.org/blog/standalone_uuid_generator_in_javascript.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Standalone UUID generator in Javascript
Home > Blog
Standalone UUID generator in Javascript
12 June 2020 (Updated: 15 June 2020)
Here is a simple and easy (no external dependencies) method to generate UUID in your Javascript applications.
// Author: Abhishek Dutta, 12 June 2020 // License: CC0 (https://creativecommons.org/choose/zero/) function uuid() { var temp_url = URL.createObjectURL(new Blob()); var uuid = temp_url.toString(); URL.revokeObjectURL(temp_url); return uuid.substr(uuid.lastIndexOf('/') + 1); // remove prefix (e.g. blob:null/, blob:www.test.com/, ...) }
The URL.createObjectURL()
static method available in Javascript creates a unique URL to represent an object passed to it as a parameter. The uuid()
method uses this property of URL.createObjectURL()
to generate unique URL -- which is a random UUID in all the browsers that I have tested so far -- on empty objects. Here are some example UUID generated by this method:
for(var i=0; i<10; ++i) { console.log(uuid()); } f6ca05c0-fad5-46fc-a237-a8e930e7cb49 6a88664e-51e1-48c3-a85e-7bf00467e9e6 e6050f4c-e86d-4081-9376-099bfbef2c30 bde3da3c-b318-4498-8a03-9a773afa84bd ba0fda03-f806-4c2f-b6f5-1e74a299e603 62b2edc3-b09f-4bf9-8dbf-c4d599479a29 e70c0609-22ad-4493-abcc-0e3445291397 920255b2-1838-497d-bc33-56550842b378 45559c64-971c-4236-9cfc-706048b60e70 4bc4bbb9-1e90-432b-99e8-277b40af92cd
Note: The intended purpose of URL.createObjectURL()
is not to generate random UUID. So, the above method of generating UUID may cause side effects that I am not yet aware of. If you can think of any issues, please let me know.
Updates:
- Michael J. R. reported via email that
URL.createObjectURL()
in Internet Explorer 11 returns a blob URL in the following formatblob:f6976204-3976-4208-aadc-c2c4260883f7
and therefore suggested the following update to theuuid()
method:return uuid.split(/[:\/]/g).pop()
. - one800higgins : this works because generating a UUID for the blob URL is part of the spec.
- DrDuPont: Here's the file API working draft from 2010. The outputted URI was slated to be a UUID from the beginning.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK