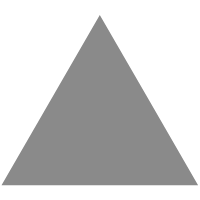
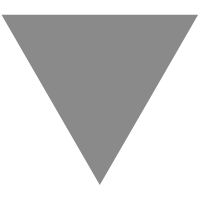
Advanced Function Concepts in Python
source link: https://mc.ai/advanced-function-concepts-in-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The usage of functions in your program improves the simplicity of code and made the debugging simple. Python gives wonderful control over creating and utilizing functions in your programs. In the previous article, I have clearly explained the process of creating a function. In this,we will learn about some advanced functional programming concepts in python. Once you mastered these things you can write better programs than before. Let me try to explain everything in simple manner as much as possible. On successful completion of this reading, you can create functions in one line of code, you can create iterators from the given sequences.
Lambda Expressions
Lambda is a pure anonymous function in python which allows us to write a function in a single line of code. It reduces the effort of programmer in writing multiple lines of code to create user defined functions. Before going to write the actual code we must understand the concept behind the lambda function. Look at the explanation about lambda in Wikipedia .
Lambda calculus(also written as λ- calculus ) is a formal system in mathematical logic for expressing computation based on function abstraction and application using variable binding and substitution. It is a universal model of computation that can be used to simulate any Turing machine.
Lambda calculus was introduced by the mathematician Alonzo Church to investigate about the foundational mathematical calculus. This calculus helps the mathematicians to understand the mechanism between basic arithmetic and logical operations. The symbolic representation of the lambda is λ Greek alphabet.
In python the function is denoted with the keyword lambda
. Look at the syntax of the lambda function.
Syntax: lambda arguments : expression
Let us create a function to calculate the volume of given cube.
def volume(side): return side*side*sideside = int(input("Enter Side of Cube")) Volume_of_cube = volume(side) print( Volume_of_cube )
Look at the following code which contains lambda
expression to create the function.
side = int(input("Enter Side of Cube")) Volume_of_cube = lambda side : side * side * sideprint( Volume_of_cube (side) )
The both codes gives the same output for similar kind of inputs as follows.
Enter Side of Cube6 216
Why Anonymous?
In the previous program we have called the lambda function with the help of the name Volume_of_cube
. But actually this name is not a function name. The previous program is just one of the way to use lambda function. In python the function created using the lambda
does not have any function name. In the program the variable name itself acted as function name. To understand clearly you can replace the entire lambda expression instead of the variable name.
side = int(input("Enter Side of Cube"))
print( (lambda side : side * side * side) (side) )
I hope you can understand the concept. That is why the lambda expression is called as anonymous function. There are certain conditions in creating lambda function in Python. More than one argument can be passed in the lambda expression but only one expression can be created in it. Some more examples are given below using lambda functions.
add = lambda a,b,c : a + b + c print( add(5,4,6) )multiply = lambda x,y : x * y print( multiply(3,7) )power = lambda m,n : m ** n print( power(6,2) )
Output
Map function in Python
Map is a function used to trace the sequencing objects. The map function reduces great effort of applying some functions for each elements of collections such as list and dictionaries. Let us try to get a list of interest amount of each employee for an particular sum of money using normal way.
Syntax: map[]
def interest(amount, rate, year): return amount * rate * year / 100amount = [10000, 12000, 15000] rate = 5 year = 3for x in amount: y = interest(x, rate, year) print(y)
Output
1500.0 1800.0 2250.0
The same can be done easily with the help of map function in Python. The following code is created using the map function.
def interest(amount): rate = 5 year = 3 return amount * rate * year / 100amount = [10000, 12000, 15000]interest_list = map(interest,amount) print( interest_list )
Output
<map object at 0x7fd6e45d90b8>
Get confused with the output? The map function always return a map object. We have type cast the object using list keyword.
def interest(amount): rate = 5 year = 3 return amount * rate * year / 100amount = [10000, 12000, 15000]interest_list = list( map(interest,amount) ) print( interest_list )
Output
[1500.0, 1800.0, 2250.0]
Map function is not only take the user defined function. We can use the built in function also.
name = ["alex", "felix", "antony"] cap = list( map(str.capitalize, name)) print(cap)
Output
['Alex', 'Felix', 'Antony']
Filter Function in Python
In some times you have to filter some elements in a list based on some conditions. Let us consider a list of ages of ten persons. We have to filter the persons based on their age. If a person’s age is greater than or equal to 24 then they can be eligible to be in the list. In a normal way, we can use something like the following.
list_of_age = [10, 24, 27, 33, 30, 18, 17, 21, 26, 25]filtered_list = []for x in list_of_age: if(x>=24): filtered_list.append(x)print( filtered_list )
Using filter function this can be very easy. The filter function returns an object that contains the elements that are true for certain conditions.
Syntax:filter(function, iterables)
def eligibility(age): if(age>=24): return True list_of_age = [10, 24, 27, 33, 30, 18, 17, 21, 26, 25]age = filter(eligibility, list_of_age)print(list(age))
The output for the previous programs are given below.
[24, 27, 33, 30, 26, 25]
Reduce function in Python
Reduce is a built in function in a module called functools. It reduces the size of iterable objects by applying function in each step. The syntax of reduce()
function is given below.
Syntax:reduce(function, iterables, start)
Let us consider a list of five numbers [1, 2, 3, 4, 5] from which you need to find the sum of the numbers. The reduce function first takes the numbers (1,2) then pass them through the defined function. Then the return value from the first one and the third value will be passed through the function. Let the name of the function be add()
then the steps involved in finding the sum will be the following.
add(1, 2) add(add(1, 2) , 3) add(add(add(1, 2) , 3), 4) add(add(add(add(1, 2) , 3), 4),5)
The actual code for the previous explanation is given below.
from functools import reduce def add(a,b): return a+b list = [1, 2, 3, 4, 5] sum = reduce(add, list) print(list(sum))
Output
Combining Lambda with Map, Filter and Reduce
The higher order functions can replace the function object with the lambda expressions. The usage of lambda expression helps us to implement the discussed concept easily with a couple of lines of code.
Lambda with Map
The following code will help you to know how to implement the map function with lambda. Let us try to create a list of power of a list.
list_of_numbers = [10, 6, 5, 17, 15] order = 2 power = map(lambda x : x ** order, list_of_numbers) print(list(power))
Output
[100, 36, 25, 289, 225]
Lambda with Filter
The conditional statements can be used with lambda expressions. Using the return value from the lambda function we can use filter the list.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even = list(filter(lambda x: x%2==0, numbers)) print(even)
Output
[2, 4, 6, 8, 10]
I hope this article helped you to understand some interesting concepts in functional programming. Practice more using the concepts explained in this article.
Happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK