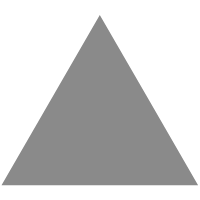
26
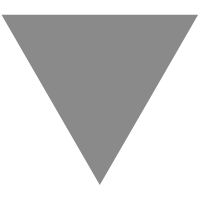
Java 查漏补缺:函数式接口
source link: http://einverne.github.io/post/2020/06/java-8-functional-interface.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Functional interface is a new feature Java 8 introduced. Functional interfaces provides target types for lambda expressions and method references.
// Assignment context Predicate<String> p = String::isEmpty; // Method invocation context stream.filter(e -> e.getSize() > 10)... // Cast context stream.map((ToIntFunction) e -> e.getSize())...
特性
- 函数式接口都是表达一种行为
- @FunctionalInterface 保证了函数式接口只有一个抽象方法,但是注解的使用是不必须的
java.util.function
相关的新的函数式接口定义在 java.util.function
包下:
void accept(T t) R apply(T t) T get() boolean test(T t)
实例
class Test { public static void main(String args[]) { // create anonymous inner class object new Thread(new Runnable() { @Override public void run() { System.out.println("New thread created"); } }).start(); } }
使用函数式接口后
class Test { public static void main(String args[]) { // lambda expression to create the object new Thread(()-> {System.out.println("New thread created");}).start(); } }
定义和使用
@FunctionalInterface interface Square { int calculate(int x); } class Test { public static void main(String args[]) { int a = 5; // lambda expression to define the calculate method Square s = (int x)->x*x; // parameter passed and return type must be // same as defined in the prototype int ans = s.calculate(a); System.out.println(ans); } }
reference
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK