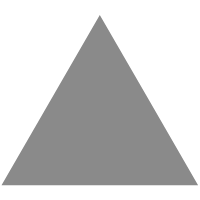
12
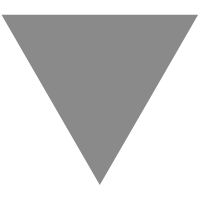
LeetCode1.两数之和
source link: https://studygolang.com/articles/28884
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
问题描述:
给定一个整数数组 nums 和一个目标值 target,请你在该数组中找出和为目标值的那 两个 整数,并返回他们的数组下标。
你可以假设每种输入只会对应一个答案。但是,你不能重复利用这个数组中同样的元素。
示例:
给定 nums = [2, 7, 11, 15], target = 9
因为 nums[0] + nums[1] = 2 + 7 = 9
所以返回 [0, 1]
解题思路:
1.暴力法
利用两层循环查找和为目标值的两个整数,并返回下标。
2.HashMap两层循环
先将数组中的值和下标作为key和value存到HashMap中,然后遍历数组,计算差值是否在HashMap中,若存在,返回对应两个目标值的下标。
[3, 3] 6 结果应为[0,0],实际为[1,1]
3.HashMap一次循环
将数组元素和下标依次存到HashMap中,同时寻找当前元素的目标差值是否已经在HashMap中,若存在,则返回目标差值的下标和当前循环下标。
代码展示:
Java版:
import java.util.HashMap; /* * @lc app=leetcode.cn id=1 lang=java * * [1] 两数之和 */ class Solution { //HashMap一次循环 public int[] twoSum(int[] nums, int target) { HashMap<Integer, Integer> map = new HashMap<>(); for(int j = 0; j < nums.length; j++){ int sub = target - nums[j]; if(map.containsKey(sub)){ return new int[]{map.get(sub), j}; } map.put(nums[j], j); } return new int[2]; } //HashMap两次循环 public int[] twoSum1(int[] nums, int target) { HashMap<Integer, Integer> map = new HashMap<>(); for(int i = 0; i < nums.length; i++){ map.put(nums[i], i); } for(int j = 0; j < nums.length; j++){ int sub = target - nums[j]; if(map.containsKey(sub) && sub != nums[j]){ return new int[]{map.get(sub), j}; } } return new int[2]; } //暴力法 public int[] twoSum2(int[] nums, int target){ for(int i = 0; i < nums.length; i++){ for(int j = i+1; j < nums.length; j++){ if(nums[i] + nums[j] == target){ return new int[]{i, j}; } } } return new int[2]; } }
Go版:
package leetcode // 暴力法 func twoSum(nums []int, target int) []int { result := make([]int, 2) for i := 0; i < len(nums); i++ { for j := i + 1; j < len(nums); j++ { if nums[i]+nums[j] == target { result[0] = i result[1] = j return result } } } return result } // HashMap一次循环 func twoSum2(nums []int, target int) []int { result := make([]int, 2) m := make(map[int]int) for j := 0; j < len(nums); j++ { sub := target - nums[j] v, ok := m[sub] if ok { result[0] = v result[1] = j return result } m[nums[j]] = j } return result } // HashMap两次循环,有缺陷 func twoSum3(nums []int, target int) []int { result := make([]int, 2) m := make(map[int]int) for i := 0; i < len(nums); i++ { m[nums[i]] = i } for j := 0; j < len(nums); j++ { sub := target - nums[j] v, ok := m[sub] if ok && v != nums[j] { result[0] = v result[1] = j return result } } return result }
欢迎关注我们的微信公众号,每天学习Go知识

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK