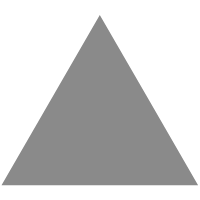
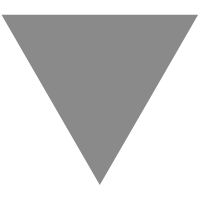
JavaScript Tips & Tricks – Mohammad S. Jaber
source link: https://msjaber.com/javascript-tips-and-tricks/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
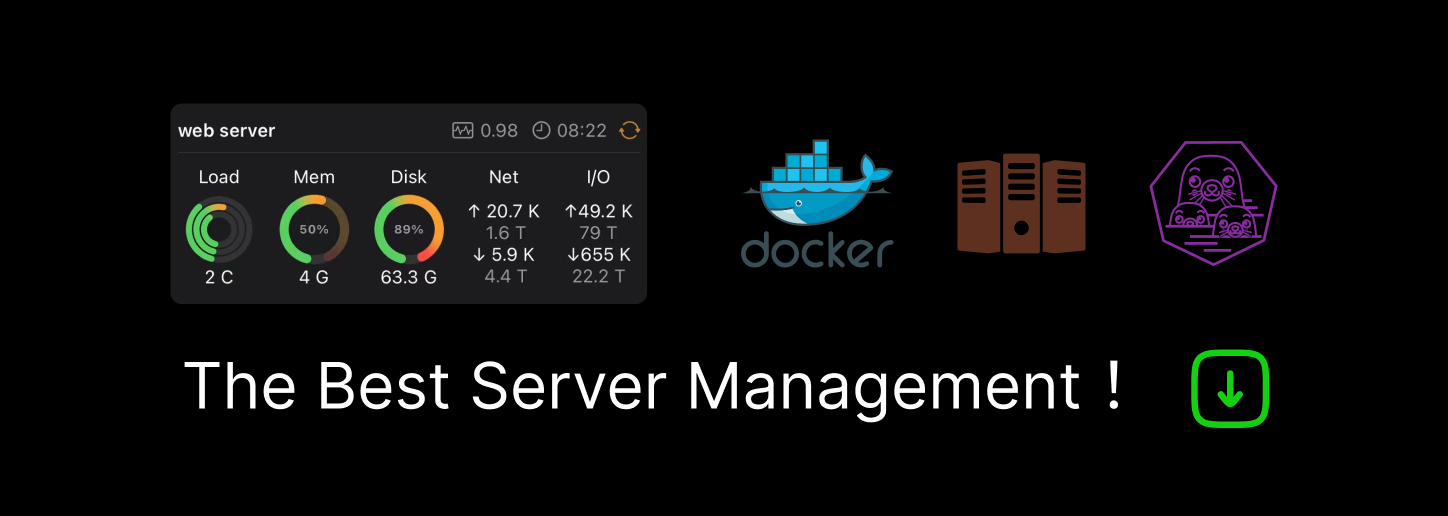
Continuously updated collection of the tips and tricks I use in my daily work. Some introduce complexity or reduce readability – the trade off is to use what suites you in your own context.
1. If statement alternative
In JavaScript, the conditional statement is executed from left to right. If a single condition is false, all the conditions on its right will not be executed.
So false && console.log('foo')
will never print a foo.
This could be useful as a replacement for if
statement.
// Instead of this...
if (foo !== undefined) {
console.log(foo)
}
You can type..
foo && console.log(foo)
A practical example is when you try to access objects properties, if the object is undefined
it will raise an excpetion.
let foo
console.log(foo.bar) // Excpetion: `Cannot read property 'bar' of undefined`
To be in the safe side, only access the object’s property if it’s not undefined
:
foo && console.log(foo.bar)
2. The not not !! operator
Any variable could be turned into a boolean form by using the precedent !!
.
A null
, undefined
, 0
and empty string ""
will evaluate to false. Otherwise, true.
Instead of strictly checking if a variable equals undefined
or null
… or if the string is empty foo.length == 0
if (foo === undefined || foo === null ) { ... }
if (foo.length == 0 ) { ... }
You can just type !!foo
if (!! foo) { ... }
3. Conditional spread operator
Spread operator is used to exapand an array and add more elements to it.
let foo = [1,2,3]
let bar = [4,5,6]
let fooBar = [...foo, ...bar] // return [1,2,3,4,5,6]
We can append an array based on certain conditions by surronding the conditional statement with two braces ( )
. This can be useful whenever you’re forced to write inline JavaScript.
let fooBar = [...(foo.length > 10 ? foo : [] ), ...bar]
This line of code implies the following: only append foo
if it has more than 10 elements.
⚠️ Caution: This may make your code less readable, I only use it when I’m forced to write inline JavaScript.
4. Return Object with Arrow Function
Arrow functions implicitly return a value if its body has only single expression, without using braces.
const foo = () => 'bar' // => bar
If braces are used, it should explicitly return a value
const foo = () => { 'bar' } // => undefined
const foo = () => { return 'bar' } // => bar
Now, if you want to return an oject implicitly (since the object literal already has braces), you can surround it with parentheses
const foo = () => { foo: 'bar' } // => undefined
const foo = () => ({ foo: 'bar' }) // => {foo: 'bar'}
Recommend
-
90
Looking at the picture above, you see an illustration of my preferred road to setup continuous integration. In this article, I am going to be explaining how to properly setup CI process for your iOS…
-
11
Top 10 Chrome DevTools tips & tricks Arek Nawo | 17 May 2021 | 9 min read DevTools are u...
-
9
Human ai Posted on Dec 8...
-
9
Protecting Website from Hackers: Tips & Tricks Nowadays, the question — how to save your website from hackers and other nasty attacks — is prevalent. In addition, after the...
-
10
Many .NET developers use Visual Studio as their Integrated Development Environment (IDE). Visual Studio has many features designed to simplify developers’ lives and be more productive. In this article, I want to show 13 tips and trick...
-
4
Laravel Livewire is a great tool to achieve dynamic behavior on the page, without directly writing JavaScript code. And, like any tool, it has a lot of "hidden gems", both in its official docs, and practical extra tips provided by developers....
-
11
Augmented Reality using AFrame: Tips & Tricks AFrame is a very cool javascript library which allows you to create Virtual Reality environments completely within the browser.
-
17
Tips & Tricks to Solve Accenture PsuedocodesTips & Tricks to Solve Accenture PsuedocodesHi, all Welcome to our platform Geeks for...
-
11
11 Tips & Tricks In Model-driven Power Apps 2022-06-16 Categories: Dynamics & Power Platform I'm...
-
6
Mohammad Jaber: My BSV blockchain journey—from counterfeiting to carbon credits Interviews 12 hours ago
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK