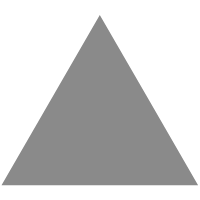
31
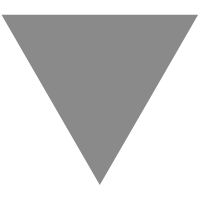
一种密码加密存储方案:加盐哈希
source link: http://www.cnblogs.com/Jason-blogs/p/12822361.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
今天来聊一聊一种被广泛使用的密码加密存储方案:加盐哈希。
本文包含以下内容:
- 什么是盐?
- 什么是哈希算法?
- 加密与校验过程。
- C#实现。
什么是盐?
In cryptography, a salt is random data that is used as an additional input to a one-way function that hashes data, a password or passphrase. -- From Wiki.
其实说白了,盐就是在密码学中用于加密的随机数据。
什么是哈希算法?
A cryptographic hash function (CHF) is a hash function that is suitable for use in cryptography. It is a mathematical algorithm that maps data of arbitrary size (often called the "message") to a bit string of a fixed size (the "hash value", "hash", or "message digest") and is a one-way function, that is, a function which is practically infeasible to invert. -- From Wiki.
密码哈希函数(CHF)是适用于密码学的哈希函数。 这是一种数学算法,可将任意大小的数据(通常称为“消息”)映射到固定大小的字符串上(“哈希值”,“哈希”或“消息摘要”),并且是单向的函数,不可逆转。这里包含了密码哈希函数重要的两个特点:1. 可将任意大小数据映射到固定大小数据上。2. 不可逆转。参考下面的图片(源于Wiki),不管input长短,经过哈希算法之后,都会生成一个固定长短的哈希值。并且在input上一个微小的改变,对哈希结果的影响都是巨大的。
加密与校验过程
C#实现
生成盐
1 /// <summary> 2 /// 生成盐 3 /// </summary> 4 /// <returns></returns> 5 public static byte[] GetSalt() 6 { 7 //Buffer storage. 8 var salt = new byte[8]; 9 using (var provider = new RNGCryptoServiceProvider()) 10 { 11 provider.GetBytes(salt); 12 } 13 return salt; 14 }
合并盐与明文
1 /// <summary> 2 /// 合并盐与明文 3 /// </summary> 4 /// <param name="byte1"></param> 5 /// <param name="byte2"></param> 6 /// <returns></returns> 7 private static byte[] CombineByteArray(byte[] byte1, byte[] byte2) 8 { 9 var ret = new byte[byte1.Length + byte2.Length]; 10 Array.Copy(byte1, 0, ret, 0, byte1.Length); 11 Array.Copy(byte2, 0, ret, byte1.Length, byte2.Length); 12 return ret; 13 }
哈希合并后数据
1 /// <summary> 2 /// Hash Salted input and salt 3 /// </summary> 4 /// <param name="input">input</param> 5 /// <param name="salt">salt</param> 6 /// <returns>return 32 bytes hashed values</returns> 7 public static byte[] HashSalted256(string input, byte[] salt) 8 { 9 var bytes = Encoding.Unicode.GetBytes(input); 10 byte[] hashSaltedValue = null; 11 using (var sha256 = new SHA256CryptoServiceProvider()) 12 { 13 var saltedInput = CombineByteArray(bytes, salt); 14 hashSaltedValue = sha256.ComputeHash(saltedInput); 15 } 16 return hashSaltedValue; 17 }
参考链接:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK