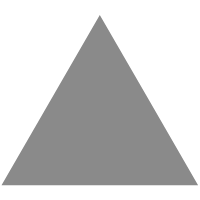
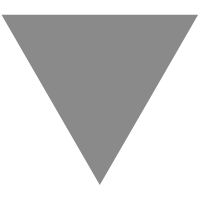
Image Augmentation with skimage — Python
source link: https://mc.ai/image-augmentation-with-skimage - python-2/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

Image Augmentation with skimage — Python
Hey buddies, recently I was working on an image classification problem. But unfortunately, there were no enough samples in one of class. I searched on the internet and learned about a technique called Image Augmentation. Here I have shared my understanding of this technique and shared some codes using skimage, you can find the jupyter notebook at the bottom.
What is Image Augmentation?
Image Augmentation is a technique used to artificially increase the size of your image dataset. It can be achieved by applying random transformations to your image.
We know Deep learning models are able to generalize well when it is able to see more data, Data Augmentation can create variations of existing images which helps to generalize well. Image Augmentation can be applied mainly on two domains of Image
- Position Augmentation
- Color Augmentation
What is Position Augmentation?
Positon Augmentation is simple where we apply different transformations on pixel positions.
Scaling, Rotation, Cropping, Flipping, Padding, Zoom, Translation, Shearing, and other Affine transformations are examples for the Position Augmentation. Let us try applying some of these transformations.
import numpy as np from skimage.io import imread, imsave import matplotlib.pyplot as plt from skimage import transform from skimage.transform import rotate, AffineTransform from skimage.util import random_noise from skimage.filters import gaussian from scipy import ndimage# load Image img = imread('./butterfly.jpg') / 255# plot original Image plt.imshow(img) plt.show()
# image rotation using skimage.transformation.rotate rotate30 = rotate(img, angle=30) rotate45 = rotate(img, angle=45) rotate60 = rotate(img, angle=60) rotate90 = rotate(img, angle=90)fig = plt.figure(tight_layout='auto', figsize=(10, 7))fig.add_subplot(221) plt.title('Rotate 30') plt.imshow(rotate30)fig.add_subplot(222) plt.title('Rotate 45') plt.imshow(rotate45)fig.add_subplot(223) plt.title('Rotate 60') plt.imshow(rotate60)fig.add_subplot(224) plt.title('Rotate 90') plt.imshow(rotate90)plt.show()
# image shearing using sklearn.transform.AffineTransform # try out with differnt values of shear tf = AffineTransform(shear=-0.5) sheared = transform.warp(img, tf, order=1, preserve_range=True, mode='wrap')sheared_fig = plot_side_by_side(img, sheared, 'Original', 'Sheared')
# Image rescaling with sklearn.transform.rescale rescaled = transform.rescale(img, 1.1)rescaled_fig = plot_side_by_side(img, rescaled, 'Original', 'Rescaled') plt.show()print('Original Shape: ',img.shape) print('Rescaled Shape: ',rescaled.shape)Output: Original Shape: (684, 1024, 3) Rescaled Shape: (752, 1126, 3)
# flip up-down using np.flipud up_down = np.flipud(img)fig_updown = plot_side_by_side(img, up_down, 'Original', 'Up-Down') plt.show()
# flip up-down using np.flipud left_right = np.fliplr(img)fig_lr = plot_side_by_side(img, left_right, 'Original', 'Up-Right') plt.show()
What is Color Augmentation?
Color Augmentation is the technique where we play with the intensity value of pixels.
We reproduce different images by tweaking Brightness, Contrast, Saturation, and also we can add random noise to the Image.
# Apply Random Noise to image using skimage.utils.random_noise noised = random_noise(img, var=0.1**2)fig_noised = plot_side_by_side(img, noised, 'Original', 'Noised') plt.show()
# Increasing the brighness of the Image # Note: Here we add 100/255 since we scaled Intensity values of Image when loading (by dividing it 255) highB = img + (100/255)fig_highB = plot_side_by_side(img, highB, 'Original', 'highB') plt.show()
# Increasing the contrast of the Image # Note: Here we add 100/255 since we scaled Intensity values of Image when loading (by dividing it 255) highC = img * 1.5fig_highB = plot_side_by_side(img, highC, 'Original', 'highC') plt.show()
Did you notice one thing, we have already created 11 different images from 1 Image. Note we can still play with parameters and create a lot more.
When training neural networks we can add Random transformations to the ImageLoader. There are other advanced techniques like using GAN for Data Augmentation, let us see that in another article. I hope now you understand what is Image Augmentation.
You can find the notebook at https://github.com/Mathanraj-Sharma/sample-for-medium-article/blob/master/image-augmentation-skimage/image-augmentation.ipynb
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK