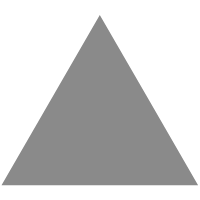
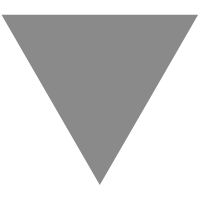
Kehaw's blog-Electron使用串口通信
source link: http://www.kehaw.com/electron/2019/11/13/Electron%E4%BD%BF%E7%94%A8%E4%B8%B2%E5%8F%A3%E9%80%9A%E4%BF%A1.html?
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Electron使用串口通信
Electron使用串口通信比调用DLL更加方便,在串口通信中,需要首先安装依赖:
npm install --save serialport
然后我们在src/background.ts
中加入调用串口的代码即可。
一个简单的例子如下:
const SerialPort = require('serialport')
const port = new SerialPort('/dev/tty-usbserial1', {
baudRate: 57600
})
其中,串口的路径是必须的(即第一个形参),而所有的选项则都不是必须的(第二个形参)。
构造SerialPort对象会立即打开一个端口。 尽管可以随时读写(操作将排队直到打开端口),但是大多数端口功能都需要打开端口。 有三种方法可以检测端口何时打开。
- 打开端口时始终会发出open事件。
- 如果尚未禁用
autoOpen
选项,则将构造函数的openCallback
传递给.open()
函数。 如果已禁用它,则回调将被忽略。 .open()
函数接受在打开端口后调用的回调。 如果禁用了autoOpen
选项或先前已关闭开放端口,则可以使用此功能。
const SerialPort = require('serialport')
const port = new SerialPort('/dev/tty-usbserial1')
port.write('main screen turn on', function(err) {
if (err) {
return console.log('Error on write: ', err.message)
}
console.log('message written')
})
// Open errors will be emitted as an error event
port.on('error', function(err) {
console.log('Error: ', err.message)
})
上面的代码是通过.on()
函数来检测错误,同时检测错误的代码也可以写入到.write()
函数的回调函数中。
const SerialPort = require('serialport')
const port = new SerialPort('/dev/tty-usbserial1', function (err) {
if (err) {
return console.log('Error: ', err.message)
}
})
port.write('main screen turn on', function(err) {
if (err) {
return console.log('Error on write: ', err.message)
}
console.log('message written')
})
Auto Open
如果你禁用了 autoOpen
选项,则你就需要手动的通过 .open()
函数打开。
const SerialPort = require('serialport')
const port = new SerialPort('/dev/tty-usbserial1', { autoOpen: false })
port.open(function (err) {
if (err) {
return console.log('Error opening port: ', err.message)
}
// Because there's no callback to write, write errors will be emitted on the port:
port.write('main screen turn on')
})
// The open event is always emitted
port.on('open', function() {
// open logic
})
获取数据可能是串口通信最简单的部分了(其实其他的也很简单)
// Read data that is available but keep the stream in "paused mode"
port.on('readable', function () {
console.log('Data:', port.read())
})
// Switches the port into "flowing mode"
port.on('data', function (data) {
console.log('Data:', data)
})
// Pipe the data into another stream (like a parser or standard out)
const lineStream = port.pipe(new Readline())
测试也比较简单,直接通过port
对象写入一些数据就可以了:
port.write('Hi Mom!')
port.write(Buffer.from('Hi Mom!'))
就是这么简单。
更详细的可以参考这里
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK