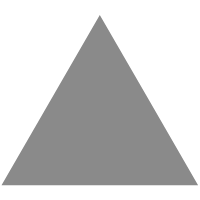
0
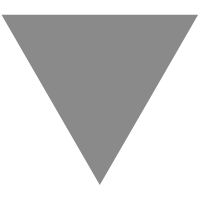
TypeScript to C++
source link: https://github.com/ASDAlexander77/TypeScript2Cxx
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TypeScript to C++
License
TypeScript2Cxx is licensed under the MIT license.
Chat Room
Want to chat with other members of the TypeScript to C++ community?
Quick Start
- Build Project
npm install npm run build
- Compile test.ts
create file test.ts
class Person { protected name: string; constructor(name: string) { this.name = name; } } class Employee extends Person { private department: string; constructor(name: string, department: string) { super(name); this.department = department; } public get ElevatorPitch() { return `Hello, my name is ${this.name} and I work in ${this.department}.`; } } const howard = new Employee("Howard", "Sales"); console.log(howard.ElevatorPitch);
node __out\main.js test.ts
Now you have test.cpp and test.h
test.h:
#ifndef TEST_H #define TEST_H #include "core.h" using namespace js; class Person; class Employee; class Person : public object { public: string name; Person(string name); }; class Employee : public Person { public: string department; Employee(string name, string department); virtual any get_ElevatorPitch(); Employee(string name); }; extern Employee* howard; #endif
test.cpp:
#include "test.h" using namespace js; Person::Person(string name) { this->name = name; } Employee::Employee(string name, string department) : Person(name) { this->department = department; } any Employee::get_ElevatorPitch() { return "Hello, my name is "_S + this->name + " and I work in "_S + this->department + "."_S; } Employee::Employee(string name) : Person(name) { } Employee* howard = new Employee("Howard"_S, "Sales"_S); void Main(void) { console->log(howard->get_ElevatorPitch()); } int main(int argc, char** argv) { Main(); return 0; }
- Compile it.
cl /W3 /GR /EHsc /std:c++latest /Fe:test.exe /I ../cpplib ../cpplib/core.cpp test.cpp
- Run it.
test.exe
Result:
Hello, my name is Howard and I work in Sales.
Enjoy it.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK