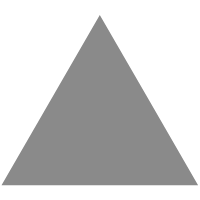
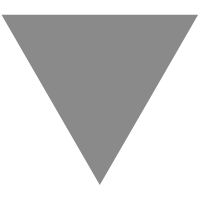
Go的条件语句「遇到问题该怎么办?先假设、后执行」
source link: https://studygolang.com/articles/27566
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
package main import "fmt" func main() { var A, B int = 520, 1314 if A > B { fmt.Println("A > B") } if A < B { fmt.Println("B < A") } fmt.Println("程序到此运行结束......") } /* 输出: B < A 程序到此运行结束...... */ 复制代码
上面我们声明两个 int 变量 A = 520 和 B = 1314
第一个 IF 语句后面跟着的是 A > B,这个结果得出 False,在这里 A 是小于B的,所以括号里的内容不执行
第二个 IF 语句后面跟着的是 A < B,这个结果得出 True,所以执行 IF 括号中的内容
IF 的格式
if 布尔表达式 {} //如果为 True,执行括号内容;如果为 False,不执行括号内容 复制代码
IF...ELSE 条件语句
IF 和 ELSE IF的情况
package main import "fmt" func main() { var A, B, C int = 22, 21, 30 if B > A { fmt.Println("B说:我的年龄怎么可能比A小,你怎么不执行我") }else if C > A{ fmt.Println("C说:我才是老大哥") }else { fmt.Println("上面所有条件都不符合的时候,才会执行我") } fmt.Println("程序到此运行结束......") } /* 输出: C说:我才是老大哥 程序到此运行结束...... */ 复制代码
声明 3 个变量,A = 22,B = 21,C = 30
第一个 IF 中,B > A,返回 False,条件不执行,执行下面的ELSE IF
第二个 ELSE IF中,C > A,返回 True,条件执行,执行完毕,跳过ELSE
程序结束
当 IF 执行的时候,下面的其他 ELSE IF 和 ELSE 都不会执行
IF 和 ELSE的情况
package main import "fmt" func main() { var A, B, C int = 22, 21, 30 if B > A { fmt.Println("B说:我的年龄怎么可能比A小,你怎么不执行我") }else if B > C{ fmt.Println("B说:我比A小就算了,还比C小") }else { fmt.Println("上面所有条件都不符合的时候,才会执行我") } fmt.Println("程序到此运行结束......") } /* 输出: 上面所有条件都不符合的时候,才会执行我 程序到此运行结束...... */ 复制代码
当 IF 和 ELSE IF都不满足条件的时候,执行 ELSE中的语句
ELSE 也可以单独接在 IF 后面
package main import "fmt" func main() { var A, B int = 22, 21 if B > A { fmt.Println("B说:我的年龄怎么可能比A小,你怎么不执行我") }else { fmt.Println("只要你IF不满足条件,就会执行我!!") } fmt.Println("程序到此运行结束......") } /* 输出: 只要你IF不满足条件,就会执行我!! 程序到此运行结束...... */ 复制代码
在这里,只要IF 不满足条件,就会执行ELSE
IF 嵌套语句
package main import "fmt" func main() { var A, B, C int = 22, 21, 30 if C > B { fmt.Println("C说:我的年龄比B大") if C > A { fmt.Println("C说:我的年龄甚至比A还大") } }else { fmt.Println("只要你IF不满足条件,就会执行我!!") } fmt.Println("程序到此运行结束......") } /* 输出: C说:我的年龄比B大 C说:我的年龄甚至比A还大 程序到此运行结束...... */ 复制代码
外面一层、里面一层都可以跟 ELSE IF 和 ELSE 搭配
Switch 语句
匹配 int 类型
switch 后没跟内容的
package main import "fmt" func main() { var A, B, C, D int = 11, 22, 33, 44 switch { case B > A: fmt.Println("B > A") case C > B: fmt.Println("C > B") case D > C: fmt.Println("D > C") } } /* 输出: B > A */ 复制代码
Go 语言的 case,只要执行了,自动停止后面的 case语句,自带 break
switch 后面带内容的
package main import "fmt" func main() { var A, B, C, D int = 11, 22, 33, 44 var num int = 11 switch num { case D : fmt.Println("与D匹配") case C: fmt.Println("与C匹配") case B: fmt.Println("与B匹配") case A: fmt.Println("与A匹配") } } /* 输出: 与A匹配 */ 复制代码
带内容的,需要与下面的变量或者内容匹配,如果匹配正确就执行
匹配字符串类型
switch 后面带内容的
package main import "fmt" func main() { var A, B, C, D string = "A", "B", "C", "D" var num string = "D" switch num { case D : fmt.Println("与D匹配") case C: fmt.Println("与C匹配") case B: fmt.Println("与B匹配") case A: fmt.Println("与A匹配") } } /* 输出: 与D匹配 */ 复制代码
switch 后面不带内容的
package main import "fmt" func main() { var A, B, C, D string = "A", "B", "C", "D" var num string = "A" switch { case D == num : fmt.Println("与D匹配") case C == num: fmt.Println("与C匹配") case B == num: fmt.Println("与B匹配") case A == num: fmt.Println("与A匹配") } } /* 输出: 与A匹配 */ 复制代码
支持多条件匹配
package main import "fmt" func main() { var A, B, C, D string = "A", "B", "C", "D" var num string = "A" switch { case D == num : fmt.Println("与D匹配") case C == num, A == num: fmt.Println("与C匹配 或者 与A匹配") case B == num: fmt.Println("与B匹配") } } /* 输出: 与C匹配 或者 与A匹配 */ 复制代码
两个表达式,只要匹配一个,即可执行以下内容
执行一个case后,继续执行case的方法,加关键字 fallthrough
package main import "fmt" func main() { switch { case false: fmt.Println("1、false执行了") fallthrough case true: fmt.Println("2、我上面是个带fallthrough的false,而我是true") fallthrough case false: fmt.Println("3、我上面是个带fallthrough的true,而我是false") fallthrough case true: fmt.Println("4、我上面是个带fallthrough的true,而我是true") case true: fmt.Println("5、我上面是个没有带fallthrough的true,而我是true") fallthrough case false: fmt.Println("我上面是个带fallthrough的true,而我是false") fallthrough default: fmt.Println("只要上面没有一个符号条件,我就执行") } } /* 输出: 2、我上面是个带fallthrough的false,而我是true 3、我上面是个带fallthrough的true,而我是false 4、我上面是个带fallthrough的true,而我是true */ 复制代码
无论 fallthrough 下面的case 是 true 或者 false都会执行
default 语句是哪个 switch 里面都可以用的,作用是其它 case 都不执行的情况下,执行default 里面的语句
总结:
- switch 后面带内容的,会拿这个内容与下面的内容匹配
- switch 后面不带内容的,下面的 case后面的条件,要是 布尔类型
- case 支持多条件匹配,只要一个条件匹配,就执行里面的内容
- case 后面自带 break,与语言不同
- case 执行完毕后,要想执行后面的case,需要添加关键字 fallthrough,而后面case 的条件,不看是否 true 或者 false,都会执行
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK