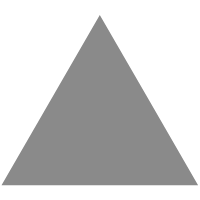
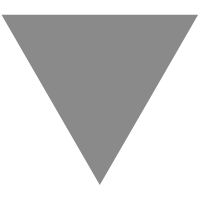
javascript - Creating object with dynamic keys - Stack Overflow
source link: https://stackoverflow.com/questions/19837916/creating-object-with-dynamic-keys
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Creating object with dynamic keys
By using our site, you acknowledge that you have read and understand our Cookie Policy, Privacy Policy, and our Terms of Service.
First off, I'm using Cheerio for some DOM access and parsing with Node.js. Good times.
Heres the situation:
I have a function that I need to create an object. That object uses variables for both its keys and values, and then return that single object. Example:
stuff = function (thing, callback) {
var inputs = $('div.quantity > input').map(function(){
var key = this.attr('name')
, value = this.attr('value');
return { key : value }
})
callback(null, inputs);
}
It outputs this:
[ { key: '1' }, { key: '1' } ]
(.map()
returns an array of objects fyi)
I need key
to actually be the string from this.attr('name')
.
Whats the best way to assign a string as a key in Javascript, considering what I'm trying to do?
In the new ES2015 standard for JavaScript (formerly called ES6), objects can be created with computed keys: Object Initializer spec.
The syntax is:
var obj = {
[myKey]: value,
}
If applied to the OP's scenario, it would turn into:
stuff = function (thing, callback) {
var inputs = $('div.quantity > input').map(function(){
return {
[this.attr('name')]: this.attr('value'),
};
})
callback(null, inputs);
}
Note: A transpiler is still required for browser compatiblity.
Using Babel or Google's traceur, it is possible to use this syntax today.
In earlier JavaScript specifications (ES5 and below), the key in an object literal is always interpreted literally, as a string.
To use a "dynamic" key, you have to use bracket notation:
var obj = {};
obj[myKey] = value;
In your case:
stuff = function (thing, callback) {
var inputs = $('div.quantity > input').map(function(){
var key = this.attr('name')
, value = this.attr('value')
, ret = {};
ret[key] = value;
return ret;
})
callback(null, inputs);
}
You can't define an object literal with a dynamic key. Do this :
var o = {};
o[key] = value;
return o;
There's no shortcut (edit: there's one now, with ES6, see the other answer).
Not the answer you're looking for? Browse other questions tagged javascript object or ask your own question.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK