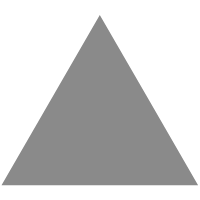
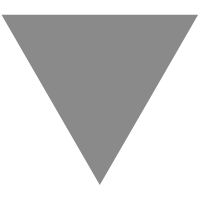
9 Tips for Building Awesome Reusable React Components
source link: https://blog.bitsrc.io/9-tips-for-building-awesome-reusable-react-components-b91f4846a30a
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Tips for building reusable and shareable React components.
Type-Checking Reusable Components
Type-checking is crucial for a good dev experience. When l etting others use and maintain your code , you should do your best to make it as easily understood as possible — no one likes long and tiresome code investigations.
1. Use TypeScript
TypeScript and prop-types are currently two of the most popular ways for type-checking React code. The former validates types at compile-time, whereas the latter performs at runtime.
As the two function differently and serve quite different purposes, the case could be made that they actually do not compete with each other.
That is true — in theory.
In the real world, TS and prop-types have considerable overlap in the way they’re used. That is why you would rarely see them both used on the same project. Writing fool-proof and comprehensible code is great but that is still secondary to actually delivering a good product, and doing that in a reasonable time.
As TypeScript offers much more than simple type-checking (better code suggestions in supported IDEs, etc.) and as it is increasingly becoming the standard for web development, I think it is the right pick for React, especially for reusable components.
Export & import shared components as source-code to enjoy the fun dev experience that TS delivers.
When sharing reusable components using tools like Bit , components can be exported and imported to any repo/codebase as source-code — making all the great benefits of TypeScript available to consumers and/or maintainers of these components.

Topping the “traditional” documentation with TS ensures an awsome dev experience.
Having your docs auto-generated using react-docgen / bit.dev is another major benefit of TS (in that case, a benefit shared with prop-types).
For example, this —
produces that ( on the component’s page ):

2. Define Components (Not Just Props & Events)
The component above is typed as a functional component ( React.FC<T>
). That’s important because otherwise, TS will not except those implicit things we take for granted when using functional components (e.g, having ‘children’ as a valid prop).
Here’s a short example:
when trying to label the Button component, like so:
function App() {return ( <div className="App"> <Button color='day'>a lable!</ButtonA> </div> ); }
this error will show up:
Type '{ children: string; color: "day"; }' is not assignable to type 'IntrinsicAttributes & IButtonProps'. Property 'children' does not exist on type 'IntrinsicAttributes & IButtonProps'.
3. Write Your TS in a Way that Enables Auto Documentation
When using react-docgen / bit.dev to auto-generate docs for your reusable components, the props’ type/interface should be defined both as a generic of React.FC<T>
and (directly) as the function's arguments type (otherwise, your props will not appear in the generated docs):
4. Inherit Prop Types for Native-HTML-like Components
A better way to define a React component that behaves like a native HTML element would be to extend its type with one of React’s HTML element types:
Structuring directories
5. One Directory For Each Component
Place all the component’s related files under the same directory. That should also include child components that are never used independently of their parent component (in that case, place the child component in a sub-directory).
Placing all files under the same directory is yet another way to make your code more comprehensible to other developers (maintainers and consumers of your reusable components). It’s much easier to understand how different files relate to each other when they’re grouped under the same directory. Moreover, it’s a way to make your reusable component more ‘mobile’ and autonomous.
Placing your component’s related files by their types, under different directories, is terribly cumbersome and not as clear to deal with.
6. Use Aliases
Reference other components using aliases. Having a path like the above makes it hard to move the component files around as you need to keep the reference valid. Using backward relative paths couples the component to the specific file structure of the project and forces the consuming project to use a similar structure. Webpack, Rollup, and Typescript provide methods for using fixed references instead of relative ones. Typescript uses the paths
mapping to create a mapping to reference components. Rollup uses the @rollup/plugin-alias
for creating similar aliases, and Webpack is using the setting of resolve.alias
for the same purpose.
APIs
The general rule of thumb is to keep your components’ API surface to the absolute necessary minimum. Again, you should always aim for simplicity of use— reusable components should not have a learning curve.
7. Use Enums (Instead of Multiple Booleans)
Use Enums instead of multiple booleans. Interdependencies between parameters make your components harder to use.
For example, do this:
instead of this:
8. Set Defaults
Setting defaults is yet another way to make your reusable components simple and easy to use. Customizing your reusable components should be other developer’s prerogative, not a mandate.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK