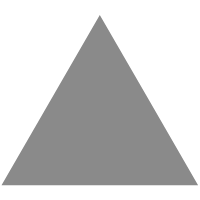
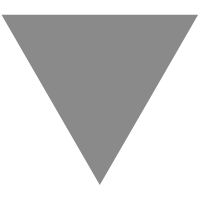
golang template使用自定义函数
source link: https://studygolang.com/articles/26891
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
golang template使用自定义函数
需求来源是这样的:如何拿到一个slice的最后一个元素
在模版里面,我们可以获取slice的任意元素,只要给定下标即可,例如:
package main import ( "os" "log" "text/template" ) const templateText = ` Friends : {{.Friends}} 1st Friend : {{index .Friends 0}} 2nd Friend : {{index .Friends 1}} 3rd Friend : {{index .Friends 2}} ` func main() { type Recipient struct { Name string Friends []string } recipient := Recipient{ Name : "Jack", Friends : []string {"Bob", "Json", "Tom"}, } t := template.Must(template.New("").Parse(templateText)) err := t.Execute(os.Stdout, recipient) if err != nil { log.Println("Executing template:", err) } }
运行:
Friends : [Bob Json Tom] 1st Friend : Bob 2nd Friend : Json 3rd Friend : Tom
然而,如何获取最后一个元素呢?因为这个下标不是一个常量,是根据slice大小动态变化的。
我们首先会想到使用len(.Friends)行不行?
const templateText = ` Nrd Friend : {{index .Friends (len .Friends)}} `
执行模版会报错:
Executing template: template: :<line>:<column>: executing "" at <index .Friends (len .Friends)>: error calling index: index out of range: 3
因为slice最后一个元素下标是(len()-1),看来用一个算术运行就可以了,遗憾的是golang template并没有提供简单的加减运算,也没有相应的函数(golang template的所有系统函数都在这儿: https://golang.org/pkg/text/template/#hdr-Functions
);所以我们必须自定义一个减函数(sub)
const templateText = ` Nrd Friend : {{index .Friends (sub (len .Friends) 1)}} ` templateFunc := map[string]interface{} { "sub": func(a, b int) int { return a - b }, } t := template.Must(template.New("").Funcs(template.FuncMap(templateFunc)).Parse(templateText))
运行结果:
Nrd Friend : Tom
可见函数sub起效了。
这里我们定义的是通用的add/sub函数,那么我们能不能直接定义last函数呢,返回slice的最后一个元素:
const templateText = ` Nrd Friend : {{last .Friends}} ` templateFunc := map[string]interface{} { "last": func(s []interface{}) interface{} { return s[len(s)-1] }, } t := template.Must(template.New("").Funcs(template.FuncMap(templateFunc)).Parse(templateText))
运行:
Executing template: template: :<line>:<column>: executing "" at <.Friends>: wrong type for value; expected []interface {}; got []string
是不是很遗憾,为啥通用类型interface{}不支持呢,一定要具体类型吗?我们重新定义last函数:
templateFunc := map[string]interface{} { "last": func(s []string) string { return s[len(s)-1] }, }
再运行:
Nrd Friend : Tom
竟然好了。这个说来我觉得还是有点遗憾了,为啥不支持通用类型interface{}呢,本来slice取其中的元素操作本身并不需要关系元素类型,用interface{}是很好的方法。
抱怨归抱怨,我也没有办法,只能遵从这个设计吧。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK