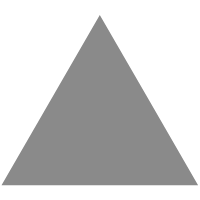
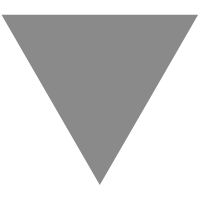
Automlpipeline.jl – machine learning tooling from IBM
source link: https://github.com/IBM/AutoMLPipeline.jl
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
AutoMLPipeline
is a package that makes it trivial to create complex ML pipeline structures using simple expressions. Using Julia macro programming features, it becomes trivial to symbolically process and manipulate the pipeline expressions and its elements to automatically discover optimal structures for machine learning prediction and classification.
Load the AutoMLPipeline package and submodules
using AutoMLPipeline, AutoMLPipeline.FeatureSelectors, AutoMLPipeline.EnsembleMethods using AutoMLPipeline.CrossValidators, AutoMLPipeline.DecisionTreeLearners, AutoMLPipeline.Pipelines using AutoMLPipeline.BaseFilters, AutoMLPipeline.SKPreprocessors, AutoMLPipeline.Utils
Load some of filters, transformers, learners to be used in a pipeline
#### Decomposition pca = SKPreprocessor("PCA"); fa = SKPreprocessor("FactorAnalysis"); ica = SKPreprocessor("FastICA") #### Scaler rb = SKPreprocessor("RobustScaler"); pt = SKPreprocessor("PowerTransformer"); norm = SKPreprocessor("Normalizer"); mx = SKPreprocessor("MinMaxScaler") #### categorical preprocessing ohe = OneHotEncoder() #### Column selector catf = CatFeatureSelector(); numf = NumFeatureSelector() #### Learners rf = SKLearner("RandomForestClassifier"); gb = SKLearner("GradientBoostingClassifier") lsvc = SKLearner("LinearSVC"); svc = SKLearner("SVC") mlp = SKLearner("MLPClassifier"); ada = SKLearner("AdaBoostClassifier") jrf = RandomForest(); vote = VoteEnsemble(); stack = StackEnsemble(); best = BestLearner();
Load data
using CSV profbdata = CSV.read(joinpath(dirname(pathof(AutoMLPipeline)),"../data/profb.csv")) X = profbdata[:,2:end] Y = profbdata[:,1] |> Vector; head(x)=first(x,5) head(profbdata)
Filter categories and hot-encode them
pohe = @pipeline catf |> ohe tr = fit_transform!(pohe,X,Y) head(tr)
Filter numeric features, compute ica and pca features, and combine both features
pdec = @pipeline (numf |> pca) + (numf |> ica) tr = fit_transform!(pdec,X,Y) head(tr)
A pipeline expression example for classification using the Voting Ensemble learner
# take all categorical columns and hotbit encode each, # concatenate them to the numerical features, # and feed them to the voting ensemble pvote = @pipeline (catf |> ohe) + (numf) |> vote pred = fit_transform!(pvote,X,Y) sc=score(:accuracy,pred,Y) println(sc) ### cross-validate crossvalidate(pvote,X,Y,"accuracy_score",5)
Print corresponding function call of the pipeline expression
@pipelinex (catf |> ohe) + (numf) |> vote # outputs: :(Pipeline(ComboPipeline(Pipeline(catf, ohe), numf), vote))
Another pipeline example using the RandomForest learner
# combine the pca, ica, fa of the numerical columns, # combine them with the hot-bit encoded categorial features # and feed all to the random forest classifier prf = @pipeline (numf |> rb |> pca) + (numf |> rb |> ica) + (catf |> ohe) + (numf |> rb |> fa) |> rf pred = fit_transform!(prf,X,Y) score(:accuracy,pred,Y) |> println crossvalidate(prf,X,Y,"accuracy_score",5)
A pipeline for the Linear Support Vector for Classification
plsvc = @pipeline ((numf |> rb |> pca)+(numf |> rb |> fa)+(numf |> rb |> ica)+(catf |> ohe )) |> lsvc pred = fit_transform!(plsvc,X,Y) score(:accuracy,pred,Y) |> println crossvalidate(plsvc,X,Y,"accuracy_score",5)
Extending AutoMLPipeline
# If you want to add your own filter/transformer/learner, it is trivial. # Just take note that filters and transformers expect one input argument # while learners expect input and output arguments in the fit! function. # transform! function always expect one input argument in all cases. # First, import the abstract types and define your own mutable structure # as subtype of either Learner or Transformer. Also load the DataFrames package using DataFrames import AutoMLPipeline.AbsTypes: fit!, transform! #for function overloading export fit!, transform!, MyFilter # define your filter structure mutable struct MyFilter <: Transformer variables here.... function MyFilter() .... end end #define your fit! function. # filters and transformer ignore Y argument. # learners process both X and Y arguments. function fit!(fl::MyFilter, X::DataFrame, Y::Vector=Vector()) .... end #define your transform! function function transform!(fl::MyFilter, X::DataFrame)::DataFrame .... end # Note that the main data interchange format is a dataframe so transform! # output should always be a dataframe as well as the input for fit! and transform!. # This is necessary so that the pipeline passes the dataframe format consistently to # its filters/transformers/learners. Once you have this filter, you can use # it as part of the pipeline together with the other learners and filters.
Feature Requests and Contributions
We welcome contributions, feature requests, and suggestions. Here is the link to open an issue for any problems you encounter. If you want to contribute, please follow the guidelines in contributors page .
Help usage
Usage questions can be posted in:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK