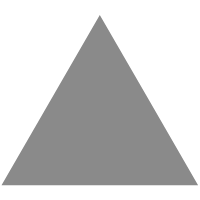
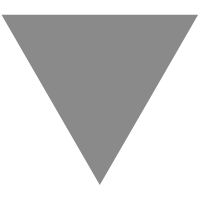
GitHub - PrasadG193/kyaml2go: K8s Go client code generator from Kubernetes resou...
source link: https://github.com/PrasadG193/kyaml2go
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
kyaml2go (Pronounced as camel2go 🐫)
Go client code generator from Kubernetes resource specs yaml
Supported resources
List of supported Kinds and Groups can be found here: https://github.com/PrasadG193/kyaml2go/blob/master/pkg/kube/map.go#L38
Installation
Install From Source
Step 1: Clone the repo
$ git clone https://github.com/PrasadG193/kyaml2go.git
Step 2: Build binary using make
$ make
Step 3: Convert K8s specs into Go client code
$ cat testdata/service.yaml apiVersion: v1 kind: Service metadata: name: mysql-service labels: app: mysql spec: ports: - name: server port: 8080 targetPort: 5000 protocol: TCP type: ClusterIP selector: app: mysql
$ kyaml2go create -f testdata/service.yaml // Auto-generated by kyaml2go - https://github.com/PrasadG193/kyaml2go package main import ( "fmt" corev1 "k8s.io/api/core/v1" metav1 "k8s.io/apimachinery/pkg/apis/meta/v1" "k8s.io/apimachinery/pkg/util/intstr" "k8s.io/client-go/kubernetes" "k8s.io/client-go/tools/clientcmd" "k8s.io/client-go/util/homedir" "os" "path/filepath" ) func main() { // Create client var kubeconfig string kubeconfig, ok := os.LookupEnv("KUBECONFIG") if !ok { kubeconfig = filepath.Join(homedir.HomeDir(), ".kube", "config") } config, err := clientcmd.BuildConfigFromFlags("", kubeconfig) if err != nil { panic(err) } clientset, err := kubernetes.NewForConfig(config) if err != nil { panic(err) } kubeclient := clientset.CoreV1().Services("default") // Create resource object object := &corev1.Service{ TypeMeta: metav1.TypeMeta{ Kind: "Service", APIVersion: "v1", }, ObjectMeta: metav1.ObjectMeta{ Name: "mysql-service", Labels: map[string]string{ "app": "mysql", }, }, Spec: corev1.ServiceSpec{ Ports: []corev1.ServicePort{ corev1.ServicePort{ Name: "server", Protocol: corev1.Protocol("TCP"), Port: 8080, TargetPort: intstr.IntOrString{ Type: intstr.Type(0), IntVal: 5000, }, NodePort: 0, }, }, Selector: map[string]string{ "app": "mysql", }, Type: corev1.ServiceType("ClusterIP"), HealthCheckNodePort: 0, }, } // Manage resource _, err = kubeclient.Create(object) if err != nil { panic(err) } fmt.Println("Service Created successfully!") }
Usage
kyaml2go is available at https://kyaml2go.prasadg.dev
Alternatively, you can also use CLI to generate code
CLI
$ kyaml2go --help NAME: kyaml2go - Generate go code to manage Kubernetes resources using go-client sdks USAGE: kyaml2go [global options] command [command options] [arguments...] VERSION: 0.0.0 COMMANDS: create Generate code for creating a resource update Generate code for updating a resource get Generate code to get a resource object delete Generate code for deleting a resource help, h Shows a list of commands or help for one command GLOBAL OPTIONS: --help, -h show help --version, -v print the version
$ kyaml2go create/get/update/delete -f /path/to/resource_specs.yaml
Workflow/Algorithm:
End-to-end workflow and algorithm to find imports can be found here
Contributing
We love your input! We want to make contributing to this project as easy and transparent as possible, whether it's:
- Reporting a bug
- Discussing the current state of the code
- Submitting a fix
- Proposing new features
Credits
The Go Gopher is originally by Renee French
This artwork is borrowed from an awesome artwork collection by Egon Elbre
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK