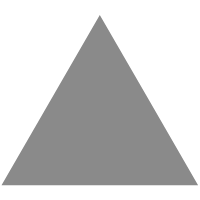
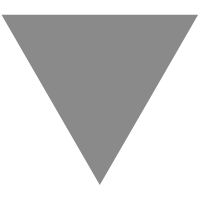
Modern Full-Stack Development with Nest.js, React, TypeScript, and MongoDB: Part...
source link: https://auth0.com/blog/modern-full-stack-development-with-nestjs-react-typescript-and-mongodb-part-1/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Nest.js
Modern Full-Stack Development with Nest.js, React, TypeScript, and MongoDB: Part 1
Learn how to build modern and secure Full-Stack applications with React, TypeScript, and Nest.js.

Olususi Oluyemi
Software Engineer / Technical Content Creator
January 27, 2020

Nest.js
Modern Full-Stack Development with Nest.js, React, TypeScript, and MongoDB: Part 1
Learn how to build modern and secure Full-Stack applications with React, TypeScript, and Nest.js.

Olususi Oluyemi
Software Engineer / Technical Content Creator
January 27, 2020
TL;DR:In this series, you will learn how to build a modern web application using React and Nest.js. In the end, you will also learn how to secure the application withAuth0. Rather than building a React application with JavaScript, we will deviate a bit and use TypeScript instead. Structuring the application this way comes with a lot of benefits such as type checking, enforcing, and associating every variable within the application with a datatype, cool, right? In this first part of the series, we will focus on building the backend API with Nest.js. The second part is about building the user interface and handling all frontend logic using React. The complete source code developed throughout this series can be found in this GitHub repository if you will prefer to head straight into the code.
Prerequisites
Basic knowledge and previous experience with building web applications with React will help you to get the best out of this series. Although not mandatory, you should know a few things about TypeScript . And to make it easy for everyone to follow along, I will endeavor to break down any complex implementation. Furthermore, you will also need to ensure that you have Node.js and Yarn package manager installed in your development machine. If you are yet to get them installed, kindly follow the instructions here to learn how to install Node.js properly and here for Yarn package manager .
Also, you need to have MongoDB installed on your machine. Follow the instructions here to download and install it for your choice of the operating system. To successfully install MongoDB, you can either install it by using Homebrew on Mac or by downloading it from the MongoDB website .
This tutorial uses a macOS machine for development. If you’re using another operating system, you may need to use sudo
for npm
commands in the first steps.
Introduction
Nest.js is a progressive Node.js framework with a modular architecture for building efficient, reliable, and scalable server-side applications. It is fully built with TypeScript, yet it still preserves compatibility with JavaScript and takes advantage of the latest JavaScript features. It brings design patterns and mature solutions to the Node.js development world. If you are conversant with the structure of Angular applications, you are going to feel more comfortable working with Nest.js. If you are new to Nest.js, check out this article on Nest.js Brings TypeScript to Node.js and Express to get yourself familiarized with some of the key concepts of Nest.js.
React is an open-source JavaScript frontend framework for building an intuitive and interactive user interface. It is widely adopted and a top choice among developers because of its great performance and simplicity in the rapid development of Single-Page Applications. Learn how React works and equip yourself with its key concepts in this React tutorial; Building and Securing Your First App .
MongoDB is a schema-less NoSQL database that can receive and store data in JSON-like documents. It takes away the idea of thinking and visualizing a database table in rows and columns. It allows you to be more productive by building JavaScript applications in a JSON format, making it not strange to any JavaScript developer. It supports arrays and nested objects values and allows for flexible and dynamic schemas. It is often used with Mongoose, an Object Data Modeling (ODM) library, that helps to manage relationships between data and provides schema validations.
"MongoDB allows you to be more productive by building JavaScript applications in a JSON format"
TypeScript , as described on its official website, is a superset of JavaScript that compiles down to plain JavaScript. It was designed and developed to help improve the productivity of developers when building large and complex programs by adding extra features that ensure the successful development of awesome applications with fewer bugs. You can easily focus on implementing new features without getting too worried over breaking an existing application in production, as TypeScript can allow you to easily spot errors in the code at a very early stage.
As pointed out earlier, in this tutorial, we will combine these awesome contemporary web tools and build an application with it. At the end of the article, you would have gathered enough knowledge for you to explore the benefits of building applications by combining React and TypeScript in either a new project or to enhance your existing projects.
Why Build React Applications with TypeScript
As you may be aware, React is a component-based frontend framework and make use of props and state objects here and there. Building React applications with TypeScript allows you to have strongly-typed components that have well defined and identifiable props and state objects. This will ensure that the usages of your components are type-checked by the compiler, and you can spot errors on time.
This will, in a nutshell, make you more productive as a developer and make your code easier to read and understand.
"React is a component-based frontend framework."
What You Will Build
You are going to build a blog application with which users can:
- Create and save a new post.
- View the newly saved post and all other created posts on the homepage.
- Carry out processes such as editing and deleting posts.
Also, to persist data into the database, you will make use of MongoDB. Here is a preview of what to expect at the end of this tutorial:
This application will allow users to create a blog post only if they have been authenticated and authorized. Otherwise, they will only be able to view the created posts by authenticated users. Authentication and authorization of users will be handled by Auth0.
You will start gradually and take things one step at a time, starting by building the complete backend API and allow any user to have access and make successful API calls to create, retrieve, and edit data in the database. Then you will proceed to secure the API by managing user authentication via Auth0. To test all the implementation in this part of the series, you will use Postman .
Building Backend APIs with Nest.js
As mentioned, the backend API will be built using Nest.js. Here, you will start by installing and configuring Nest.js and then proceed to flesh out the structure necessary for the API.
Installing and configuring Nest.js
You can easily install a new Nest.js project using the command-line interface built specifically for scaffolding Nest applications called Nest CLI .
You will start by using the command-line interface built specifically for scaffolding a new Nest.js application to create yours. Alternatively, you can clone the starter project for Nest.js here on GitHub . Even though both approaches will produce the same outcome, for the sake of this tutorial and as it recommended for a first time user by the Nest.js team, you will use the Nest CLI to create your project.
Install the CLI by running the following command:
npm install -g @nestjs/cli
Once the process is complete, confirm if Nest CLI has been installed by running the following command:
nest --version
You will see an output similar to the following indicating the version installed on your machine:
6.6.4
:bulb: Please note that this version might be different from yours.
Craft a new project for this tutorial by using the nest
command as shown here:
nest new blog-backend
Immediately after running the preceding command, nest
will prompt you to choose a package manager you would like to use. Select npm
and hit ENTER
on your computer to start installing Nest.js:
This will create a new Nest.js project in a blog-backend
directory within your local development folder. Now, move into the newly created directory and run a command to install other required server dependencies:
// change directory cd blog-backend // install dependency npm install --save @nestjs/mongoose mongoose
Because Nest.js supports integrating with MongoDB database by using Mongoose, what you have done here is to install mongoose
and the dedicated package created by Nest.js team for the integration named @nestjs/mongoose
.
Once the installation process is complete, you can then easily import the MongooseModule
into your application. More about this later in the tutorial.
Next, before you start the application, open the project using your code editor, and edit the default port as shown below:
// /blog-backend/src/main.ts import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create(AppModule); await app.listen(5000);// edit this } bootstrap();
What you have done here is to change the default port of Nest.js to 5000
to avoid port conflict with the React application, which you will build in the next part of this series. That will run on port 3000
by default.
With that done, start the application using the following command:
npm run start:dev
This will run the application on port 5000
on your local machine. Navigate to http://localhost:5000 from your browser of choice, and you will see your application running.
In this section, you have successfully installed the Nest CLI and leveraged it to generate the project for this tutorial. You then proceeded to run the application on the default port 5000
. Next, you will work on ensuring a successful connection between your app and its database that will be created.
Configuring a data
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK