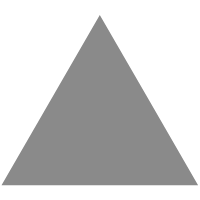
16
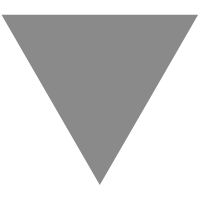
Golang入门-时间处理
source link: https://studygolang.com/articles/26068
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
import time func getCurTime() { // 本地时间(如果是在中国,获取的是东八区时间) curLocalTime := time.Now() // UTC时间 curUTCTime := time.Now().UTC() fmt.Println(curLocalTime, curUTCTime) } 复制代码
设置时区
func setTimeZone() { curLocalTime := time.Now() curUtcTime := curLocalTime.In(time.UTC) fmt.Println(curUtcTime) } 复制代码
时间类型转字符串: Format函数
func time2TimeStr() { localTimeStr := time.Now().Format("2006-01-02 15:04:05") // UTC时间 utcTimeStr := time.Now().UTC().Format("2006-01-02 15:04:05") fmt.Println(localTimeStr, utcTimeStr) } 复制代码
时间类型转时间戳: Unix函数
func getCurTimeStamp() { // 时间戳,精确到秒 timestamp := time.Now().Unix() // 时间戳,精确到纳秒 timestampNano := time.Now().UnixNano() fmt.Println(timestamp, timestampNano) } 复制代码
时间戳转时间类型:Unix函数
func timestamp2Time() { timestamp := time.Now().Unix() localTimeObj := time.Unix(timestamp, 0) fmt.Println(localTimeObj) } 复制代码
时间字符串转时间类型:parse函数
func timeStr2Time() { timeStr := "2020-01-13 22:32:17" // 返回的是UTC时间 2020-01-13 22:32:17 +0000 UTC utcTimeObj, err := time.Parse("2006-01-02 15:04:05", timeStr) if err == nil { fmt.Println(utcTimeObj, utcTimeObj.Unix()) } // 返回的是当地时间 2020-01-13 22:32:17 +0800 CST localTimeObj, err := time.ParseInLocation("2006-01-02 15:04:05", timeStr, time.Local) if err == nil { fmt.Println(localTimeObj) } } 复制代码
时间字符串转时间戳:
parse函数 + Unix函数
时间戳转字符串:
Unix函数 + Format函数
时间加减
注:(转换为Time类型比较容易做加减)
func addTime() { curTime := time.Now() // 加1秒 addSecondTime := curTime.Add(time.Second * 1) // 加1分钟 addMinuteTime := curTime.Add(time.Minute * 1) addMinuteTime2 := curTime.Add(time.Second * time.Duration(60*1)) fmt.Println(addSecondTime, addMinuteTime, addMinuteTime2) } 复制代码
注: 这部分摘抄自想入飞飞的《Golang周期任务、定时任务》: www.jianshu.com/p/4955e5d65…
周期任务:每隔5秒
func intervalTask() { ticker := time.NewTicker(time.Second * 5) go func() { for _ = range ticker.C { fmt.Println("do the thing") } }() time.Sleep(time.Minute) } 复制代码
倒计时任务:主线程阻塞
func countDownSyncTask() { timer1 := time.NewTimer(time.Second * 5) <-timer1.C fmt.Println("do the thing") } 复制代码
倒计时任务:主线程不阻塞
func countDownAsyncTask() { timer1 := time.NewTimer(time.Second * 3) go func() { //等触发时的信号 <-timer1.C fmt.Println("do the thing") }() //由于上面的等待信号是在新线程中,所以代码会继续往下执行,停掉计时器 time.Sleep(time.Second * 10) } 复制代码
定时任务,每天10:00:00触发
func scheduleTask() { go func() { for { fmt.Println("触发的事件") now := time.Now() // 计算下一个零点 next := now.Add(time.Hour * 24) next = time.Date(next.Year(), next.Month(), next.Day(), 10, 0, 0, 0, next.Location()) fmt.Println(next.Sub(now)) t := time.NewTimer(next.Sub(now)) <-t.C } }() time.Sleep(time.Hour * 24) } 复制代码
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK