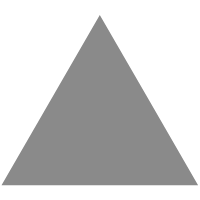
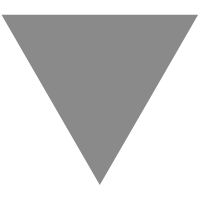
GitHub - hmlongco/Resolver: Swift Ultralight Dependency Injection / Service Loca...
source link: https://github.com/hmlongco/Resolver
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
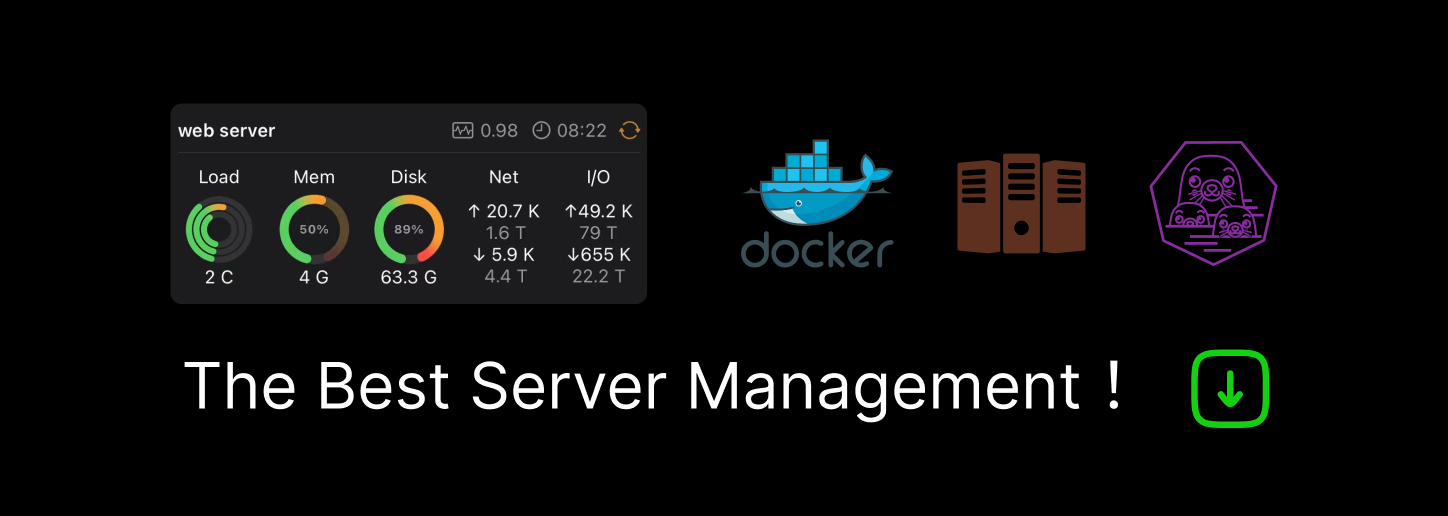
README.md
Resolver 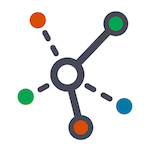
An ultralight Dependency Injection / Service Locator framework for Swift 5.1 on iOS.
Introduction
Dependency Injection frameworks support the Inversion of Control design pattern. Technical definitions aside, dependency injection pretty much boils down to:
| Giving an object the things it needs to do its job.
That's it. Dependency injection allows us to write code that's loosely coupled, and as such, easier to reuse, to mock, and to test.
For more, see: A Gentle Introduction to Dependency Injection.
Dependency Injection Strategies
There are six classic dependency injection strategies:
- Interface Injection
- Property Injection
- Constructor Injection
- Method Injection
- Service Locator
- Annotation (NEW)
Resolver supports them all. Follow the links for a brief description, examples, and the pros and cons of each.
Property Wrappers
Resolver now supports resolving services using the new property wrapper syntax in Swift 5.1.
class BasicInjectedViewController: UIViewController { @Injected var service: XYZService @LazyInjected var service2: XYZLazyService }
Just add the Injected keyword and your dependencies will be resolved automatically. See the Annotation documentation for more on this and other strategies.
Features
Resolver is implemented in just over 300 lines of actual code, but it packs a ton of features into those 300 lines.
- Automatic Type Inference
- Scopes: Application, Cached, Graph, Shared, and Unique
- Protocols
- Optionals
- Named Instances
- Argument Passing
- Custom Containers & Nested Containers
- Cyclic Dependency Support
- Storyboard Support
TLDR: If nothing else, make sure you read about Automatic Type Inference, Scopes, and Optionals.
Using Resolver
Using Resolver is a simple, three-step process:
- Add Resolver to your project.
- Register the classes and services your app requires.
- Use Resolver to resolve those instances when needed.
Installation
Resolver supports CocoaPods and the Swift Package Manager.
pod "Resolver"
Resolver itself is just a single source file (Resolver.swift), so it's also easy to simply download the file and add it to your project.
Note that the current version of Resolver (1.1) supports Swift 5.1. For Swift 5 or Swift 4, checkout an earlier version.
Why Resolver?
As mentioned, Resolver is an ultralight Dependency Injection system, implemented in just over 300 lines of code and contained in a single file.
Resolver is also designed for performance. SwinjectStoryboard, for example, is a great dependency injection system, but Resolver clocks out to be about 800% faster at resolving dependency chains than Swinject.
And unlike some other systems, Resolver is written in 100% Swift 5, with no Objective-C code, method swizzling, or internal dependencies on the Objective-C runtime.
Further, Resolver:
- Is tested in production code.
- Is thread safe (assuming your objects are thread safe).
- Has a complete set of unit tests.
- Is well-documented.
Finally, with Automatic Type Inference you also tend to write about 40-60% less dependency injection code using Resolver.
Author
Resolver was designed, implemented, and documented by Michael Long, a Senior Lead iOS engineer at CRi Solutions. CRi is a leader in developing cutting edge iOS, Android, and mobile web applications and solutions for our corporate and financial clients.
- Email: [email protected]
- Twitter: @hmlco
License
Resolver is available under the MIT license. See the LICENSE file for more info.
Additional Resouces
- API Documentation
- Inversion of Control Design Pattern ~ Wikipedia
- Inversion of Control Containers and the Dependency Injection pattern ~ Martin Fowler
- Nuts and Bolts of Dependency Injection in Swift\
- Dependency Injection in Swift
- SwinjectStoryboard
- Swift 5.1 Takes Dependency Injection to the Next Level
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK