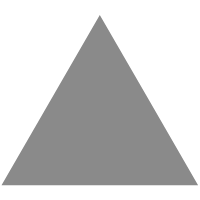
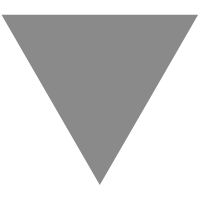
Rules of Exception Handling with Java Method Overriding
source link: https://www.scientecheasy.com/2019/04/exception-handling-method-overriding.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
When a super class method (overridden method) declares that it can throw an exception then sub class method (overriding method) must also declare that it can throw the same kind of exception or a sub type of that exception.
To handle the exception while you overriding a method in Java, you will have to follow three important rules. They are as follows.
1. If an overridden method does not throw an exception using throws clause then
➲ The overriding method can not throw any checked or compile-time exception.
➲ The overriding method can throw any unchecked or runtime exception.
2. If an overridden method throws an unchecked or runtime exception then
➲ The overriding method can throw any unchecked or runtime exception.
➲ The overriding method can throw the same exception which is thrown by the overridden method.
➲ The overriding method can ignore the method level exception.
3. If the superclass method throws checked or compile-time exception then
➲ Subclass method can throw the exception which is a subclass of the super class method's exception.
➲ Subclass method cannot throw the exception which is a super class of the super class method's exception.
➲ Subclass method can throw any unchecked or runtime exception.
Let's take different example programs based on these rules.
Program source code 1:
package overriding; public class Parent { // Overridden method is not throwing an exception. void msg() { System.out.println("msg-Parent"); } } import java.io.IOException; public class Child extends Parent { void msg() throws IOException // Compile-time error because the overriding method is throwing a checked exception. { System.out.println("msg-Child"); } public static void main(String[] args) throws IOException { Parent p = new Child(); p.msg(); Child c = new Child(); c.msg(); } } Output: Unresolved compilation problem: Exception IOException is not compatible with throws clause in Parent.msg()In the above example program, if the overriding method throws an unchecked exception, there will be no compile-time error. Let's see the program source code.
Program source code 2:
package overriding; public class Parent { // Overridden method is not throwing an exception. void msg() { System.out.println("msg-Parent"); } } import java.io.IOException; public class Child extends Parent{ void msg() throws ArithmeticException // No compile-time error because the overriding method is throwing an unchecked exception. { System.out.println("msg-Child"); } public static void main(String[] args) throws IOException { Parent p = new Child(); p.msg(); Child c = new Child(); c.msg(); } } Output: msg-Child msg-ChildProgram source code 3:
package overriding; public class Parent { // Overridden method is throwing an unchecked exception. void msg() throws ArithmeticException { System.out.println("msg-Parent"); } } public class Child extends Parent{ void msg() throws ClassCastException // No Compile-time error because the overriding method is throwing an unchecked exception. { System.out.println("msg-Child"); } public static void main(String[] args){ Parent p = new Child(); p.msg(); Child c = new Child(); c.msg(); } } Output: msg-Child msg-ChildQ. What will be the error in the following program?
Assumption X is superclass and Y is subclass.
1. In superclass
public void m1() throws IOException
{
System.out.println("Hello");
}
In subclass
public void m1() throws Exception
{
System.out.println("Hi");
}
A. Compile-time error because the overriding method is throwing an exception which is the superclass of the superclass method' exception. i.e, Exception is the superclass of IOExecption.
public void m1() throws Throwable
{
System.out.println("Parent");
}
In subclass
public void m1() throws Exception
{
System.out.println("Child");
}
A. No compile-time error because Throwable is the superclass of all exception.
3. In base class
public void m1() throws Exception
{
System.out.println("Base");
}
In derived class
void m1() throws InterrurptedException
{
System.out.println("Child");
}
A. Compile-time error because you cannot reduce the visibility of the inherited method from Parent. Access modifiers must be bigger or the same.
4. In base class
protected void m1() throws Exception
{
System.out.println("Base");
}
In derived class
public void m1() throws Exception
{
System.out.println("Child");
}
A. No error.
5. In superclass
protected void m1(char c) throws Throwable
{
System.out.println("Parent");
}
In subclass
void m1(char c)
{
System.out.println("Child");
}
A. Error because we cannot reduce the visibility while overriding method.
Final words
Hope that this tutorial has covered almost all important rules of exception handling with method overriding in Java with example programs. I hope that you will have understood nicely this topic and enjoyed it.
Thanks for reading!
Next ➤ Difference between Overloading & Overriding ⏪ Prev Next ⏩
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK