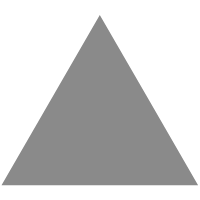
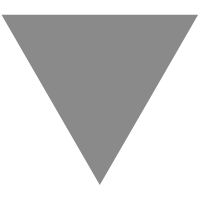
GitHub - yannickcr/eslint-plugin-react: React specific linting rules for ESLint
source link: https://github.com/yannickcr/eslint-plugin-react
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
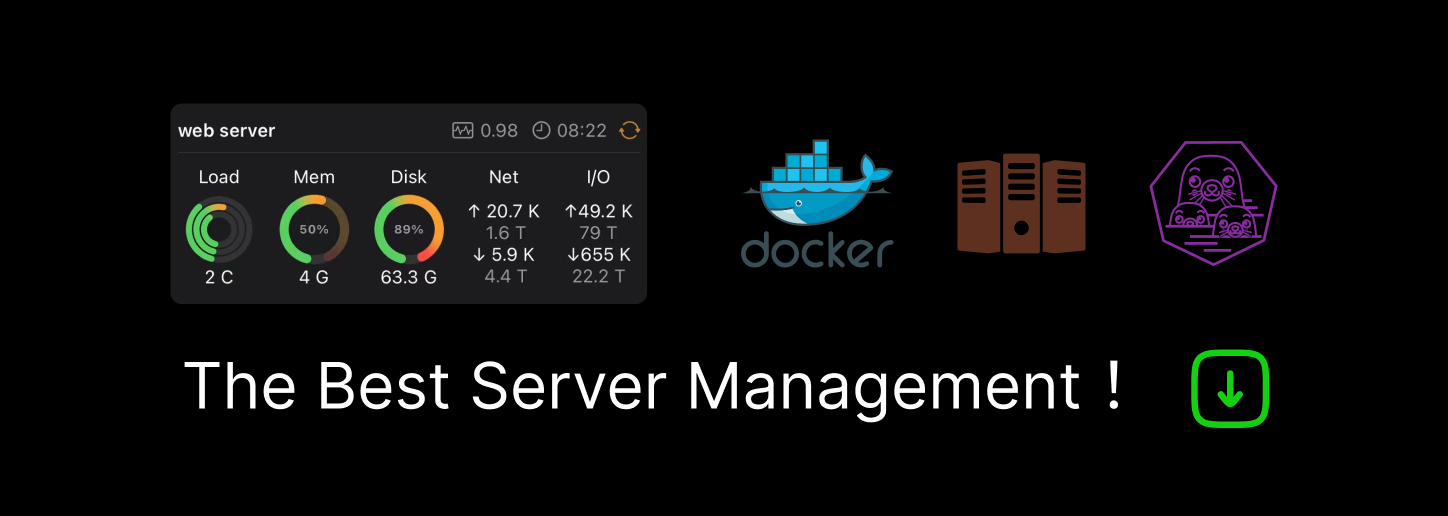
README.md
ESLint-plugin-React
React specific linting rules for ESLint
Installation
Install ESLint either locally or globally. (Note that locally, per project, is strongly preferred)
$ npm install eslint --save-dev
If you installed ESLint
globally, you have to install React plugin globally too. Otherwise, install it locally.
$ npm install eslint-plugin-react --save-dev
Configuration
Use our preset to get reasonable defaults:
"extends": [ "eslint:recommended", "plugin:react/recommended" ]
You should also specify settings that will be shared across all the plugin rules. (More about eslint shared settings)
{ "settings": { "react": { "createClass": "createReactClass", // Regex for Component Factory to use, // default to "createReactClass" "pragma": "React", // Pragma to use, default to "React" "version": "detect", // React version. "detect" automatically picks the version you have installed. // You can also use `16.0`, `16.3`, etc, if you want to override the detected value. // default to latest and warns if missing // It will default to "detect" in the future "flowVersion": "0.53" // Flow version }, "propWrapperFunctions": [ // The names of any function used to wrap propTypes, e.g. `forbidExtraProps`. If this isn't set, any propTypes wrapped in a function will be skipped. "forbidExtraProps", {"property": "freeze", "object": "Object"}, {"property": "myFavoriteWrapper"} ], "linkComponents": [ // Components used as alternatives to <a> for linking, eg. <Link to={ url } /> "Hyperlink", {"name": "Link", "linkAttribute": "to"} ] } }
If you do not use a preset you will need to specify individual rules and add extra configuration.
Add "react" to the plugins section.
{ "plugins": [ "react" ] }
Enable JSX support.
With ESLint 2+
{ "parserOptions": { "ecmaFeatures": { "jsx": true } } }
Enable the rules that you would like to use.
"rules": { "react/jsx-uses-react": "error", "react/jsx-uses-vars": "error", }
List of supported rules
- react/boolean-prop-naming: Enforces consistent naming for boolean props
- react/button-has-type: Forbid "button" element without an explicit "type" attribute
- react/default-props-match-prop-types: Prevent extraneous defaultProps on components
- react/destructuring-assignment: Rule enforces consistent usage of destructuring assignment in component
- react/display-name: Prevent missing
displayName
in a React component definition - react/forbid-component-props: Forbid certain props on Components
- react/forbid-dom-props: Forbid certain props on DOM Nodes
- react/forbid-elements: Forbid certain elements
- react/forbid-prop-types: Forbid certain propTypes
- react/forbid-foreign-prop-types: Forbid foreign propTypes
- react/no-access-state-in-setstate: Prevent using this.state inside this.setState
- react/no-array-index-key: Prevent using Array index in
key
props - react/no-children-prop: Prevent passing children as props
- react/no-danger: Prevent usage of dangerous JSX properties
- react/no-danger-with-children: Prevent problem with children and props.dangerouslySetInnerHTML
- react/no-deprecated: Prevent usage of deprecated methods, including component lifecycle methods
- react/no-did-mount-set-state: Prevent usage of
setState
incomponentDidMount
- react/no-did-update-set-state: Prevent usage of
setState
incomponentDidUpdate
- react/no-direct-mutation-state: Prevent direct mutation of
this.state
- react/no-find-dom-node: Prevent usage of
findDOMNode
- react/no-is-mounted: Prevent usage of
isMounted
- react/no-multi-comp: Prevent multiple component definition per file
- react/no-redundant-should-component-update: Prevent usage of
shouldComponentUpdate
when extending React.PureComponent - react/no-render-return-value: Prevent usage of the return value of
React.render
- react/no-set-state: Prevent usage of
setState
- react/no-typos: Prevent common casing typos
- react/no-string-refs: Prevent using string references in
ref
attribute. - react/no-this-in-sfc: Prevent using
this
in stateless functional components - react/no-unescaped-entities: Prevent invalid characters from appearing in markup
- react/no-unknown-property: Prevent usage of unknown DOM property (fixable)
- react/no-unsafe: Prevent usage of unsafe lifecycle methods
- react/no-unused-prop-types: Prevent definitions of unused prop types
- react/no-unused-state: Prevent definitions of unused state properties
- react/no-will-update-set-state: Prevent usage of
setState
incomponentWillUpdate
- react/prefer-es6-class: Enforce ES5 or ES6 class for React Components
- react/prefer-read-only-props: Enforce that props are read-only
- react/prefer-stateless-function: Enforce stateless React Components to be written as a pure function
- react/prop-types: Prevent missing props validation in a React component definition
- react/react-in-jsx-scope: Prevent missing
React
when using JSX - react/require-default-props: Enforce a defaultProps definition for every prop that is not a required prop
- react/require-optimization: Enforce React components to have a
shouldComponentUpdate
method - react/require-render-return: Enforce ES5 or ES6 class for returning value in render function
- react/self-closing-comp: Prevent extra closing tags for components without children (fixable)
- react/sort-comp: Enforce component methods order (fixable)
- react/sort-prop-types: Enforce propTypes declarations alphabetical sorting
- react/state-in-constructor: Enforce the state initialization style to be either in a constructor or with a class property
- react/static-property-placement: Enforces where React component static properties should be positioned.
- react/style-prop-object: Enforce style prop value being an object
- react/void-dom-elements-no-children: Prevent void DOM elements (e.g.
<img />
,<br />
) from receiving children
JSX-specific rules
- react/jsx-boolean-value: Enforce boolean attributes notation in JSX (fixable)
- react/jsx-child-element-spacing: Enforce or disallow spaces inside of curly braces in JSX attributes and expressions.
- react/jsx-closing-bracket-location: Validate closing bracket location in JSX (fixable)
- react/jsx-closing-tag-location: Validate closing tag location in JSX (fixable)
- react/jsx-curly-newline: Enforce or disallow newlines inside of curly braces in JSX attributes and expressions (fixable)
- react/jsx-curly-spacing: Enforce or disallow spaces inside of curly braces in JSX attributes and expressions (fixable)
- react/jsx-equals-spacing: Enforce or disallow spaces around equal signs in JSX attributes (fixable)
- react/jsx-filename-extension: Restrict file extensions that may contain JSX
- react/jsx-first-prop-new-line: Enforce position of the first prop in JSX (fixable)
- react/jsx-handler-names: Enforce event handler naming conventions in JSX
- react/jsx-indent: Validate JSX indentation (fixable)
- react/jsx-indent-props: Validate props indentation in JSX (fixable)
- react/jsx-key: Validate JSX has key prop when in array or iterator
- react/jsx-max-depth: Validate JSX maximum depth
- react/jsx-max-props-per-line: Limit maximum of props on a single line in JSX (fixable)
- react/jsx-no-bind: Prevent usage of
.bind()
and arrow functions in JSX props - react/jsx-no-comment-textnodes: Prevent comments from being inserted as text nodes
- react/jsx-no-duplicate-props: Prevent duplicate props in JSX
- react/jsx-no-literals: Prevent usage of unwrapped JSX strings
- react/jsx-no-script-url: Prevent usage of
javascript:
URLs - react/jsx-no-target-blank: Prevent usage of unsafe
target='_blank'
- react/jsx-no-undef: Disallow undeclared variables in JSX
- react/jsx-no-useless-fragment: Disallow unnecessary fragments (fixable)
- react/jsx-one-expression-per-line: Limit to one expression per line in JSX
- react/jsx-curly-brace-presence: Enforce curly braces or disallow unnecessary curly braces in JSX
- react/jsx-fragments: Enforce shorthand or standard form for React fragments
- react/jsx-pascal-case: Enforce PascalCase for user-defined JSX components
- react/jsx-props-no-multi-spaces: Disallow multiple spaces between inline JSX props (fixable)
- react/jsx-props-no-spreading: Disallow JSX props spreading
- react/jsx-sort-default-props: Enforce default props alphabetical sorting
- react/jsx-sort-props: Enforce props alphabetical sorting (fixable)
- react/jsx-space-before-closing: Validate spacing before closing bracket in JSX (fixable)
- react/jsx-tag-spacing: Validate whitespace in and around the JSX opening and closing brackets (fixable)
- react/jsx-uses-react: Prevent React to be incorrectly marked as unused
- react/jsx-uses-vars: Prevent variables used in JSX to be incorrectly marked as unused
- react/jsx-wrap-multilines: Prevent missing parentheses around multilines JSX (fixable)
Other useful plugins
- JSX accessibility: eslint-plugin-jsx-a11y
- React Native: eslint-plugin-react-native
Shareable configurations
Recommended
This plugin exports a recommended
configuration that enforces React good practices.
To enable this configuration use the extends
property in your .eslintrc
config file:
{ "extends": ["eslint:recommended", "plugin:react/recommended"] }
See ESLint documentation for more information about extending configuration files.
The rules enabled in this configuration are:
- react/display-name
- react/jsx-key
- react/jsx-no-comment-textnodes
- react/jsx-no-duplicate-props
- react/jsx-no-target-blank
- react/jsx-no-undef
- react/jsx-uses-react
- react/jsx-uses-vars
- react/no-children-prop
- react/no-danger-with-children
- react/no-deprecated
- react/no-direct-mutation-state
- react/no-find-dom-node
- react/no-is-mounted
- react/no-render-return-value
- react/no-string-refs
- react/no-unescaped-entities
- react/no-unknown-property
- react/prop-types
- react/react-in-jsx-scope
- react/require-render-return
All
This plugin also exports an all
configuration that includes every available rule.
This pairs well with the eslint:all
rule.
{ "plugins": [ "react" ], "extends": ["eslint:all", "plugin:react/all"] }
Note: These configurations will import eslint-plugin-react
and enable JSX in parser options.
License
ESLint-plugin-React is licensed under the MIT License.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK