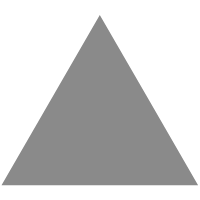
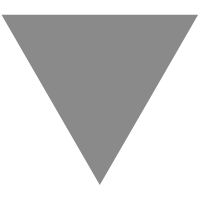
GitHub - lucasmrdt/nedux: ๐ฆ State manager - the next redux
source link: https://github.com/lucasmrdt/nedux
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Nedux - The n
ext redux
Why do you waste your time by creating actions/reducers/containers/sagas/... ?
Just create a store and that's it !
Installation
npm install nedux --save
Use Nedux
with ...
library | provider |
---|---|
React | react-nedux |
VueJS | todo |
Angular | todo |
Examples
Name | Source | Codesandbox |
---|---|---|
![]() |
here | here |
![]() |
here | here |
![]() |
here | here |
Basic Example
Use it with Typescript 
import { createStore } from 'nedux'; interface Todo { id: number; text: string; completed: boolean; } enum Filter { ShowAll = 'ShowAll', ShowCompleted = 'ShowCompleted', ShowActive = 'ShowActive', } // Create the store const todoStore = createStore({ todos: [] as Todo[], filter: Filter.ShowAll, }); // You can subscribe to field update. todoStore.subscribe('filter', newFilter => { console.log(`filter has changed with ${newFilter}`); }); // You can get a value. todoStore.get('filter'); // โ> 'ShowAll' // You can override a value. todoStore.set('filter', Filter.ShowCompleted); // Or extends value by the previous one. todoStore.set('todos', todos => [ ...todos, { id: 1, text: 'test', completed: false }, ]); // And that's it !
Or simply with Javascript
import { createStore } from 'nedux'; const todoStore = createStore({ todos: [], filter: 'ShowAll', }); todoStore.subscribe('filter', newFilter => { console.log(`filter has changed with ${newFilter}`); }); todoStore.get('filter'); todoStore.set('filter', 'ShowCompleted'); todoStore.set('todos', todos => [ ...todos, { id: 1, text: 'test', completed: false }, ]);
Documentation
Import
// ES6 import { createStore } from 'nedux'; // ES5 var createStore = require('nedux').createStore;
createStore(initialState, [middlewares])
Creates a Nedux store with the shape of the initialState
.
argument | required | type | description |
---|---|---|---|
initalState |
![]() |
object |
The intial state of your store. |
middlewares |
![]() |
Middleware[] | Middlewares are used to enhance your store see the middleware section to know more. |
store
The store
object created by createStore
it'll allow you to interact with your store.
store.get(key)
argument | required | type | description |
---|---|---|---|
key |
![]() |
string |
The key of the store that you want to get |
store.set(key, value)
argument | required | type | description |
---|---|---|---|
key |
![]() |
string |
The key of the store that you want to override |
value |
![]() |
any or (prevValue: any) => any |
The new value of the key |
store.subscribe(key, observer)
argument | required | type | description |
---|---|---|---|
key |
![]() |
string |
The key of the store that you'll subscribe to changes. (give a value of '' will subscribe to all keys changes) |
observer |
![]() |
observer or (value: any) => any |
An rxjs observer or a simple callback which will be fired when the store has been updated for the given key |
Middlewares
Middleware is the suggested way to extend Nedux with custom functionality. The created store is provided to each middleware. It's easy to subscribe
/get
/set
value to the store inside your middleware. The key feature of middleware is that it is composable. Multiple middleware can be combined together, where each middleware requires no knowledge of what comes before or after it in the chain.
Middleware | Description |
---|---|
Allow you to persist your nedux store |
Basic Logger Middleware
import { createStore } from 'nedux'; const loggerMiddleware = store => // we subscribe to all modifications store.subscribe('', value => console.log(value)); const store = createStore( { a: 0, b: 'b', }, [loggerMiddleware], ); store.set('b', 'a'); store.set('a', 1); store.set('a', a => a * 2); store.set('b', 'not b');
Advised Structure
It usually a good idea to keep the store as small as possible. You can manage your application by structure it as services. Each service will have its own store (if it's needed)
my-service โโโ components # Your components. โย ย โโโ AddTodo.tsx โย ย โโโ App.tsx โย ย โโโ FilterLink.tsx โย ย โโโ Footer.tsx โย ย โโโ Link.tsx โย ย โโโ Todo.tsx โย ย โโโ TodoList.tsx โโโ controler.ts # Where you wrap your business logic (link between api/store/ui) โโโ index.tsx # Where you export elements to other services. โโโ store.ts # Where the store is created with the initial state. โโโ types.ts # Where you put your service types.
Why choose Nedux
over Redux
?
- No more actions
- No more dispatch
- No more reducers
- No more provider
- Fully functionnal usage
- Easiest to understand
- No "magical" effect (all is traceable)
- No need to use external tools to debug (again all is traceable)
- Easiest to learn
- Fully typed (if you're coding in typescript you will
it !)
- Less code to write
- Faster and lighter (no react context, no HOC)
You just write less to do the same.
Redux todos VS Nedux todos (same code)
Feel free to inspect the structure of both of them (Redux and Nedux) and how Nedux is implemented.
Redux | Nedux | Diff (less is better) | |
---|---|---|---|
number of files | 13 |
11 |
-15.4% |
number of lines | 224 |
174 |
-22.3% |
number of characters | 4343 |
3298 |
-24.0% |
time for first render | ~10.5 ms |
~8.5 ms |
-23.5% |
add todo | ~0.8 ms |
~0.6 ms |
-33.3% |
Redux Counter VS Nedux Counter (same code)
Again feel free to test it yourself here.
Render time | Redux | Nedux | Diff (less is better) |
---|---|---|---|
with 9999 items | 0.743s |
0.481s |
-35.3% |
Structure
# Redux Todos โโโ actions โย ย โโโ index.js โโโ components โย ย โโโ App.js โย ย โโโ Footer.js โย ย โโโ Link.js โย ย โโโ Todo.js โย ย โโโ TodoList.js โโโ containers โย ย โโโ AddTodo.js โย ย โโโ FilterLink.js โย ย โโโ VisibleTodoList.js โโโ index.js โโโ reducers โโโ index.js โโโ todos.js โโโ visibilityFilter.js
# Nedux Todos โโโ components โย ย โโโ AddTodo.tsx โย ย โโโ App.tsx โย ย โโโ FilterLink.tsx โย ย โโโ Footer.tsx โย ย โโโ Link.tsx โย ย โโโ Todo.tsx โย ย โโโ TodoList.tsx โโโ controler.ts โโโ index.tsx โโโ store.ts โโโ types.ts
Scripts used
# Compute number of files find $SRC_FOLDER -type f | wc -l # Compute number of lines find $SRC_FOLDER -type f -exec cat {} \; | grep -v -e '^$' | grep -v -e '^//' | wc -l # Compute number of characters find $SRC_FOLDER -type f -exec cat {} \; | grep -v -e '^$' | grep -v -e '^//' | tr -d '[:space:] ' | wc -c
Profiling method
Profiling is made with React Profiling following this configuration :
Navigator | Chrome 78.0.3904.108 (64-bit) |
Profiling Software | React Developer Tools 4.2.1 |
OS | MacOS Catalina 10.15.1 |
Model | MacBook Pro (15-inch, 2018) |
Processor | 2.2 GHz 6-Core Intel Core i7 |
Memory | 16 GB 2400 MHz DDR4 |
Graphic | Intel UHD Graphics 630 1536 MB |
Todos
- Add sandbox for each examples
- Add tests
- Be more accurate on performance comparison
- Add more examples
- Type cleaning
- Add CI
- Add VueJS connector
- Add Angular connector
๐๐ผ Contributions
All Pull Requests, Issues and Discussions are welcomed !
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK