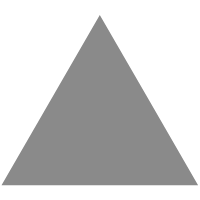
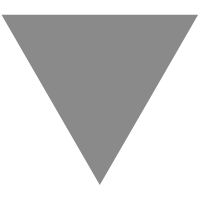
Getting Started with React (2019 Edition) ― Scotch.io
source link: https://scotch.io/starters/react/getting-started-with-react-2019-edition
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Getting Started with React (2019 Edition) ― Scotch.io
We're live-coding on Twitch! Join us!
React is the uber popular JavaScript library that is almost everywhere you look these days. Learning React opens up a whole new way to build front-end experiences like websites, mobile apps, and more.
Much has changed since React came out and now is a great time to jump into the React fray. React is mature and has been used across the web in places like Facebook, Instagram, Airbnb, Uber, and much much more. Let's dive in!
For those that want a video walkthrough so you can see every step of the way, check out our React Starter Video!
What is React?
React is a UI library built by Facebook. React gives us the ability to logically think about our frontend sites and apps.
It’s also a really fun and quick way to build the projects we dream up! Even the site you’re on, scotch.io is built with React.
React allows us to build apps that:
- Can be made quickly
- Are easy to understand
- Allow us to logically see the flow of data
- Scale well with small and large teams
- Transfer knowledge from desktop to mobile apps
React lets us build out our interactive UIs declaratively. One of its greatest abilities is the way it can update our UIs automatically and efficiently whenever our data changes.
Imagine changing a JavaScript variable and immediately seeing it change on your site. Like magic!
In this React Starter, we’ll go through all the bells and whistles of React. We’ll build apps and learn many real-world techniques. This Starter is for anyone that wants to learn React from scratch in a neat and linear path. Follow along and you’ll gain all the React knowledge needed to start building in React.
Recommended Knowledge (Prerequisites)
Building React applications requires very little base knowledge. This is one of its big benefits! It’s very easy to get up and running with React if you’re coming from an HTML/CSS/JS background.
I would recommend having:
- Knowledge of HTML & CSS
- Knowledge of JavaScript and ES6 (3 main things to know)
- Some knowledge about the DOM
- Some knowledge about Node & npm (and installed)
Note that in a future article, we’ll go over prerequisite knowledge more in-depth. For this first starter article, let’s just start seeing how we can work in React; then we can take a step back and look further at prerequisites.
Using React Quickly
There are many many ways to use React in our projects. This is one of the main benefits of React. It isn’t too opinionated about how you should use it. The two main ways to use React are:
- Add React to an already-existing website (this is the approach scotch.io uses)
- Use React to build a full single-page-app (SPA)
Many parts of scotch.io are built using React.
We’ll go over the many ways to use React in following articles. To get us up and running quickly in this article, we’ll be using CodePen. CodePen is an online coding playground where you can very easily play around with HTML/CSS/JS right in your browser. We’ll be using this for our first Starter article since we don’t want to dive into all the installation details just yet.
Give the next few articles a look to see all the ways React can be used including the CLI, create-react-app.
Setting Up a CodePen
I’ve gone ahead and created a starter CodePen for us to use for this article.
Once you open up this CodePen, you’ll be greeted with a page like this:
Note: Your editor boxes may be on the top or the side. I like mine on the left side. You can change this with the Change View button.
Steps to Create this Starter CodePen
For clarity, I want to let you know everything that went into making this React Starter CodePen. It was 3 quick steps (2 if you don’t count the “Create a CodePen” step):
- Create a new CodePen
- Add the react and react-dom scripts (hit the settings icon in the JS editor)
- Change the JS processor to Babel since we’ll be using ES6 code and Babel transpiles our code to ES5.
The main core of React is found in the react.js
script while the things needed to work with React in a browser are in react-dom.js
. These were split apart because React can be used in more places than just the browser. See React Native for an example.
That’s it! That’s how we created this starter CodePen. Your JS settings popup should look like this:
Let’s move forward and start working with React.
A Teeny Tiny React App
How Does React Work?
React works by taking over an HTML div and injecting JavaScript into it. React will inject all of the awesome cool JavaScript into this section of our site.
Adding an HTML div
We need to create an HTML div and then we can start working with React. In the HTML panel, create a div called root
or app
. Naming it root or app is standard across the React landscape since this is the main div where our React app will take place.
In the HTML section of our CodePen, add:
<div id="root"></div>
That’s all we need in HTML!
React Elements
Now that we have a div we can target, let’s start writing our JavaScript and our first piece of React code (finally!)
Feel free to collapse the HTML/CSS editors since we won’t need them anymore.
Let’s use React to inject some data into our website.
In the JavaScript section, write the following:
ReactDOM.render(
<h1>I AM HERE!</h1>,
document.getElementById('root')
);
By including the react.js
and react-dom.js
scripts, we now have access to the JavaScript objects of React
and ReactDOM
.
ReactDOM.render()
takes two parameters:
- The element (or component as we’ll see soon)
- The HTML element where React will inject things
Data in React
Now this app isn’t too useful since you’re not always going to have a site that says I AM HERE.
Let’s grab some data and see how we can add it to our React app.
In JavaScript, let’s create a variable called message
and display that:
const message = 'i am here to save the day!';
Now we can display that in our ReactDOM.render
:
const message = 'i am here to save the day!';
ReactDOM.render(
<h1>my message is: {message}</h1>,
document.getElementById('root')
);
We now have this:
Here’s the completed demo for you to take a look at:
A Talk About Components
We’ve made a React element and shown that in our site now. Moving forward, we’re going to be making React components. Components are a React tool that are made up of elements like <h1>
, <div>
, and more.
Components are the building blocks of sites and apps.
What exactly is a component?
In the simplest terms, a component is comprised of:
- a template (HTML)
- interactivity (JavaScript)
- styles (CSS)
By organizing our sites and apps into components, we are able to work with our UI as separate parts. React makes creating components as easy as creating a JavaScript function.
If you look at scotch.io, you can think of the following as components:
- site header
- site footer
- left sidebar
- main content
- article title
- article content
By having many components, we can piece together components to make any number of site pages and combinations. Websites and apps are combinations of components. Give Facebook, Google, or Twitter a look and see if you can point out the components on those sites.
Let’s move forward and start creating React components. It’s super easy!
Creating Your First React Component
Now you may be wondering how the last example is useful. We have a variable here and have displayed it using React.
Why couldn’t we just do that with HTML?
While this is a simple example, the truth of today’s websites and applications is that data comes from not just the app itself, but from various sources. You could pull data from:
- your own database
- your own API
- a 3rd party API like the Facebook/Google/Twitter APIs
- data stored in browser storage like localStorage
Managing data from those multiple sources can be tough without a UI library like React. Another big benefit to React is that once we have our data from those sources, we can automagically have the UI updated without any extra code from us. We’ll see exactly what this means soon.
Automatically update our UI whenever data changes. Building dynamic sites is good UI/UX.
If we wanted to update our UI in just plain HTML, we would have to reload the page. That leads to a bad user experience for our users. Imagine if Facebook or Twitter had to update every time new data came in!
Creating the React Component
Let’s remove the message
variable we made earlier and create a React component using a JavaScript class. Here’s our component:
function App() {
// my component goes here
}
This syntax may look familiar to you. That’s because it’s just a plain old JavaScript function!
Creating React components is as simple as creating a JavaScript function.
Note: React style conventions state that components should be PascalCase. If you had a component that you want to call site header, then the component would be named SiteHeader
.
We’ve created our first React component, but it doesn’t do anything just yet. Like we stated earlier, we are going to have our components a combination of JavaScript and a template. In React, templates are written using JSX.
We’ll cover JSX in more detail in a following article. JSX is a syntax extension to JavaScript, but for this tutorial, just think of JSX as “HTML inside of JavaScript.”
A Note About JSX
JSX is the templating language that React uses and it can be a little bit of a learning curve to many people trying to learn React.
JSX isn’t that scary. People just aren’t used to seeing the syntax after coming from HTML.
I am a firm believer that writing JSX will make you a better JavaScript developer.
We’re not going to go too far in depth with JSX in this article; JSX deserves its own articles coming up. My recommendation to you if you are wary of JSX is to give it a chance. Hold any judgment until we’ve built a few things and you’ll see it’s not as scary as you may have thought.
Adding a Template to our Component
Back to our component, let’s add a template.
Components are great conceptually because tying JavaScript to a specific template makes code far more readable and easier to manage, especially in large codebases.
Since this function is a component, where do we put our JavaScript and where do we put our template? In React functional components, we will return our template out of the function:
function App() {
// javascript stuff goes here
return (
// template goes here
);
}
Now that we know where our template goes, let’s add an <h1>
:
function App() {
// javascript stuff goes here
return (
<h1>im in a fancy component now!</h1>
);
}
Now that we have this component, we need to tell React to use it.
Using Our New Component
In React, when you create a component, you will be able to use them as if they were HTML tags in React.
For instance, we created this App
, now we can use it in React as <App />
. Notice that it has a closing forward-slash. When using React components, we need to use some kind of closing tag.
<App />
// or
<App></App>
Back in our ReactDOM.render()
, let’s use it:
function App() {
// javascript stuff goes here
return (
<h1>im in a fancy component now!</h1>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
Components Are Reusable
One of the benefits of component based design is that we can keep our code DRY (dont-repeat-yourself). We could even reuse this <App />
component multiple times
ReactDOM.render(
<div>
<App />
<App />
<App />
</div>,
document.getElementById('root')
);
Note: React always wants only 1 parent element when defining a template. This is why we wrapped everything in a single <div>
.
Components are very powerful tools that we can use to build out our websites and apps. Component architecture is everywhere you look in web development these days, whether you’re looking at web components, React, Vue, Angular, and many other frameworks, components are logical ways to split up our applications.
Let’s move forward and work with data in this app.
Displaying Data
In the previous example, before we were using components, we created a variable called message
. In components, when you want to create data, you have a couple different options:
- Create a variable (like earlier)
- Create a
state
variable
If you want to update data and have the template show that change automatically, then we will use a React concept called state.
Creating a simple variable
This is how we can recreate the earlier example. The downside to this approach is that whenever we update message
, our template won’t update to show the changes.
function App() {
const message = 'javascript is so cool';
return (
<h1>{message}</h1>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
The problem with using a simple variable like this is that React won't look to rerender whenever it changes. To be 100% sure that React will update the template with new data, we'll use a concept called React state.
Using React State to display a variable
When we want variable updates to reflect automatically in our template, we want to use React state. Let’s change this example to use React state. Then whenever the message
variable changes, React will re-render our template with the new data.
Let’s write the full syntax here and we’ll talk about what is happening. You may see some JavaScript syntax that you aren’t familiar with called array destructuring.
function App() {
const [message, setMessage] = React.useState('javascript is so cool');
return (
<h1>{message}</h1>
);
}
Notice the line that has useState:
const [message, setMessage] = React.useState('javascript is so cool');
This is called array destructuring.
- React.useState gives us two parts
- the variable which we name
message
- a function to set the variable which we’ve called
setMessage
- the variable which we name
You can name the variable and the function anything you want. We’re calling it message
and setMessage
because that is what we want our variable to be called.
We'll talk more about destructuring in an upcoming post about React prerequisites.
Inside of useState()
we can set the default value for our message
variable.
Essentially we’ve done 3 things:
- Create a
message
variable - Created a way to update the
message
variable (setMessage
) - Set a default value for
message
Whenever we use setMessage to update our message, React will update our views.
Let’s make sure that our setMessage
function is going to work.
Handling User Interaction (Events)
Now that we’ve displayed some data, the next step in any app is to listen for user input and act on it. We are going to listen for an event like a button click.
Let’s do a simple action. We’ll:
- Create a button
- Listen for a click
- Update our message using the
setMessage
function
Creating the Button
We’ll add the button next to our <h1>
. Remember that React always wants only 1 parent element. We’ll add a surrounding <div>
so that there’s only 1 parent element.
Let’s create a button that when clicked will update our message. Update your App component to have a button that takes a function when clicked:
function App() {
const [message, setMessage] = React.useState('javascript is so cool');
return (
<div>
<h1>{message}</h1>
<button>
Update the Message!
</button>
</div>
);
}
Listening for Clicks
In HTML, each element in the DOM can emit events like click
, mouseover
, keypress
, and more.
In order to listen to an event on an element in React, we prepend the event with on
. For instance, if we want to listen for a click
on this button, we will add an attribute for onClick
:
<button onClick={}>Click Me!</button>
In our onClick
, we are using the curly brackets to tell JSX that we need to evaluate what’s in these brackets {}
.
Whenever we open brackets {}, we’re telling React to evaluate this area with JavaScript.
Let’s add a function to our onClick
to make sure we can update our message using setMessage
:
function App() {
const [message, setMessage] = React.useState('javascript is so cool');
return (
<div>
<h1>{message}</h1>
<button onClick={() => setMessage('my new message')}>
Update the Message!
</button>
</div>
);
}
Let’s look further at the onClick
handler. In JSX, we will open brackets {}
whenever we want React to evaluate with JavaScript. Since we want to run a function when the button is clicked, we have opened the brackets and given a function.
This could also be written by creating a function outside of our template. The following has the same effect but keeps our template cleaner.
function App() {
const [message, setMessage] = React.useState('javascript is so cool');
function handleClick() {
setMessage('my new message')
}
return (
<div>
<h1>{message}</h1>
<button onClick={handleClick}>
Update the Message!
</button>
</div>
);
}
Now try clicking your button and seeing the message update automatically!
By using React state, we can easily update variables and have React update our views!
Recap
Let’s do a recap to see all the React concepts we’ve touched on:
- Starting a React app using
react.js
andreact-dom.js
- Rendering React into an HTML div using
ReactDOM.render
- Display React data
- Listening for React events
- Using React state
- Updating variables and having React update the UI
We’ve covered a lot of the main concepts of React. These foundational concepts will be used in every React app you make in the future!
Conclusion
We’ve only scratched the surface with this Getting Started with React article. Follow along in this React Starter Guide to learn more and more about the world’s most popular JavaScript library.
Throughout this React starter, we'll go much further in depth and diving into things like:
- Building React apps multiple ways
- Talking about components
- Routing apps
- Forms and validation
- Redux,
- Styling components with Sass and CSS-in-JS
- Animations
- And much much more...
Like this article? Follow @chrisoncode on Twitter
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK