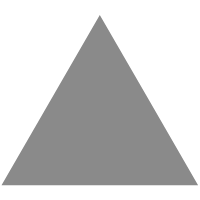
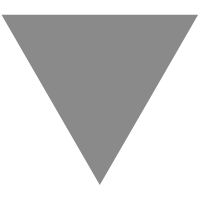
GitHub - neovim/nvim-lsp: Common configurations for Neovim Language Servers
source link: https://github.com/neovim/nvim-lsp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
nvim-lsp
WIP Common configurations for Language Servers.
This repository aims to be a central location to store configurations for
Language Servers which leverage Neovim's built-in LSP client vim.lsp
as the
client backbone. The vim.lsp
implementation is made to be customizable and
greatly extensible, but most users just want to get up and going. This
plugin/library is for those people, although it still lets you customize
things as much as you want in addition to the defaults that this provides.
NOTE: Requires current Neovim master as of 2019-11-13
CONTRIBUTIONS ARE WELCOME!
There's a lot of language servers in the world, and not enough time. See
lua/nvim_lsp/*.lua
for examples and ask us questions in the Neovim
Gitter to help us complete configurations for
all the LSPs! Read CONTRIBUTING.md
for some instructions. NOTE: don't
modify README.md
; it is auto-generated.
If you don't know where to start, you can pick one that's not in progress or
implemented from this excellent list compiled by the coc.nvim
contributors or
this other excellent list from the emacs lsp-mode
contributors
and create a new file under lua/nvim_lsp/SERVER_NAME.lua
. We recommend
looking at lua/nvim_lsp/texlab.lua
for the most extensive example, but all of
them are good references.
Progress
Implemented language servers:
Planned servers to implement (by me, but contributions welcome anyway):
In progress:
- ...
Install
Plug 'neovim/nvim-lsp'
Usage
Servers configurations can be set up with a "setup function." These are functions to set up servers more easily with some server specific defaults and more server specific things like commands or different diagnostics.
The "setup functions" are call nvim_lsp#setup({name}, {config})
from vim and
nvim_lsp[name].setup(config)
from Lua.
Servers may define extra functions on the nvim_lsp.SERVER
table, e.g.
nvim_lsp.texlab.buf_build({bufnr})
.
Auto Installation
Many servers can be automatically installed with the :LspInstall
command. Detailed installation info can be found
with the :LspInstallInfo
command, which optionally accepts a specific server name.
For example:
LspInstall elmls silent LspInstall elmls " useful if you want to autoinstall in init.vim LspInstallInfo LspInstallInfo elmls
Example
From vim:
call nvim_lsp#setup("texlab", {})
From Lua:
require 'nvim_lsp'.texlab.setup { name = "texlab_fancy"; log_level = vim.lsp.protocol.MessageType.Log; settings = { latex = { build = { onSave = true; } } } } local nvim_lsp = require 'nvim_lsp' -- Customize how to find the root_dir nvim_lsp.gopls.setup { root_dir = nvim_lsp.util.root_pattern(".git"); } -- Build the current buffer. require 'nvim_lsp'.texlab.buf_build(0)
Setup function details
The main setup signature will be:
nvim_lsp.SERVER.setup({config})
{config} is the same as |vim.lsp.start_client()|, but with some
additions and changes:
{root_dir}
May be required (depending on the server).
`function(filename, bufnr)` which is called on new candidate buffers to
attach to and returns either a root_dir or nil.
If a root_dir is returned, then this file will also be attached. You
can optionally use {filetype} to help pre-filter by filetype.
If a root_dir is returned which is unique from any previously returned
root_dir, a new server will be spawned with that root_dir.
If nil is returned, the buffer is skipped.
See |nvim_lsp.util.search_ancestors()| and the functions which use it:
- |nvim_lsp.util.root_pattern(patterns...)| finds an ancestor which
- contains one of the files in `patterns...`. This is equivalent
to coc.nvim's "rootPatterns"
- More specific utilities:
- |nvim_lsp.util.find_git_root()|
- |nvim_lsp.util.find_node_modules_root()|
- |nvim_lsp.util.find_package_json_root()|
{name}
Defaults to the server's name.
{filetypes}
A set of filetypes to filter for consideration by {root_dir}.
Can be left empty.
A server may specify a default value.
{log_level}
controls the level of logs to show from build processes and other
window/logMessage events. By default it is set to
vim.lsp.protocol.MessageType.Warning instead of
vim.lsp.protocol.MessageType.Log.
{settings}
This is a table, and the keys are case sensitive. This is for the
window/configuration event responses.
Example: `settings = { keyName = { subKey = 1 } }`
{on_attach}
`function(client)` will be executed with the current buffer as the
one the {client} is being attaching to. This is different from
|vim.lsp.start_client()|'s on_attach parameter, which passes the {bufnr} as
the second parameter instead. This is useful for running buffer local
commands.
{on_new_config}
`function(new_config)` will be executed after a new configuration has been
created as a result of {root_dir} returning a unique value. You can use this
as an opportunity to further modify the new_config or use it before it is
sent to |vim.lsp.start_client()|.
LSP Implementations
bashls
https://github.com/mads-hartmann/bash-language-server
Language server for bash, written using tree sitter in typescript.
Can be installed in neovim with :LspInstall bashls
nvim_lsp.bashls.setup({config}) nvim_lsp#setup("bashls", {config}) Default Values: cmd = { "bash-language-server", "start" } filetypes = { "sh" } log_level = 2 root_dir = vim's starting directory settings = {}
ccls
https://github.com/MaskRay/ccls/wiki
ccls relies on a JSON compilation database specified as compile_commands.json or, for simpler projects, a compile_flags.txt.
nvim_lsp.ccls.setup({config}) nvim_lsp#setup("ccls", {config}) Default Values: capabilities = default capabilities, with offsetEncoding utf-8 cmd = { "ccls" } filetypes = { "c", "cpp", "objc", "objcpp" } log_level = 2 on_init = function to handle changing offsetEncoding root_dir = root_pattern("compile_commands.json", "compile_flags.txt", ".git") settings = {}
clangd
https://clang.llvm.org/extra/clangd/Installation.html
clangd relies on a JSON compilation database specified as compile_commands.json or, for simpler projects, a compile_flags.txt.
nvim_lsp.clangd.setup({config}) nvim_lsp#setup("clangd", {config}) Default Values: capabilities = default capabilities, with offsetEncoding utf-8 cmd = { "clangd", "--background-index" } filetypes = { "c", "cpp", "objc", "objcpp" } log_level = 2 on_init = function to handle changing offsetEncoding root_dir = root_pattern("compile_commands.json", "compile_flags.txt", ".git") settings = {}
elmls
https://github.com/elm-tooling/elm-language-server#installation
If you don't want to use neovim to install it, then you can use:
npm install -g elm elm-test elm-format @elm-tooling/elm-language-server
Can be installed in neovim with :LspInstall elmls
nvim_lsp.elmls.setup({config}) nvim_lsp#setup("elmls", {config}) Default Values: capabilities = default capabilities, with offsetEncoding utf-8 cmd = { "elm-language-server" } filetypes = { "elm" } init_options = { elmAnalyseTrigger = "change", elmFormatPath = "elm-format", elmPath = "elm", elmTestPath = "elm-test" } log_level = 2 on_init = function to handle changing offsetEncoding root_dir = root_pattern("elm.json") settings = {}
flow
https://flow.org/ https://github.com/facebook/flow
See below for how to setup Flow itself. https://flow.org/en/docs/install/
See below for lsp command options.
npm run flow lsp -- --help
nvim_lsp.flow.setup({config}) nvim_lsp#setup("flow", {config}) Default Values: cmd = { "npm", "run", "flow", "lsp" } filetypes = { "javascript", "javascriptreact", "javascript.jsx" } log_level = 2 root_dir = root_pattern(".flowconfig") settings = {}
gopls
https://github.com/golang/tools/tree/master/gopls
Google's lsp server for golang.
nvim_lsp.gopls.setup({config}) nvim_lsp#setup("gopls", {config}) Default Values: cmd = { "gopls" } filetypes = { "go" } log_level = 2 root_dir = root_pattern("go.mod", ".git") settings = {}
hie
https://github.com/haskell/haskell-ide-engine
the following init_options are supported (see https://github.com/haskell/haskell-ide-engine#configuration):
init_options = { languageServerHaskell = { hlintOn = bool; maxNumberOfProblems = number; diagnosticsDebounceDuration = number; liquidOn = bool (default false); completionSnippetsOn = bool (default true); formatOnImportOn = bool (default true); formattingProvider = string (default "brittany", alternate "floskell"); } }
nvim_lsp.hie.setup({config}) nvim_lsp#setup("hie", {config}) Default Values: cmd = { "hie-wrapper" } filetypes = { "haskell" } log_level = 2 root_dir = root_pattern("stack.yaml", "package.yaml", ".git") settings = {}
pyls
https://github.com/palantir/python-language-server
python-language-server, a language server for Python
the following settings (with default options) are supported:
settings = { pyls = { enable = true; trace = { server = "verbose"; }; commandPath = ""; configurationSources = { "pycodestyle" }; plugins = { jedi_completion = { enabled = true; }; jedi_hover = { enabled = true; }; jedi_references = { enabled = true; }; jedi_signature_help = { enabled = true; }; jedi_symbols = { enabled = true; all_scopes = true; }; mccabe = { enabled = true; threshold = 15; }; preload = { enabled = true; }; pycodestyle = { enabled = true; }; pydocstyle = { enabled = false; match = "(?!test_).*\\.py"; matchDir = "[^\\.].*"; }; pyflakes = { enabled = true; }; rope_completion = { enabled = true; }; yapf = { enabled = true; }; }; }; };
nvim_lsp.pyls.setup({config}) nvim_lsp#setup("pyls", {config}) Default Values: cmd = { "pyls" } filetypes = { "python" } log_level = 2 root_dir = vim's starting directory settings = {}
rls
https://github.com/rust-lang/rls
rls, a language server for Rust
Refer to the following for how to setup rls itself. https://github.com/rust-lang/rls#setup
See below for rls specific settings. https://github.com/rust-lang/rls#configuration
If you want to use rls for a particular build, eg nightly, set cmd as follows:
cmd = {"rustup", "run", "nightly", "rls"}
nvim_lsp.rls.setup({config}) nvim_lsp#setup("rls", {config}) Default Values: cmd = { "rls" } filetypes = { "rust" } log_level = 2 root_dir = root_pattern("Cargo.toml") settings = {}
texlab
A completion engine built from scratch for (La)TeX.
See https://texlab.netlify.com/docs/reference/configuration for configuration options.
nvim_lsp.texlab.setup({config}) nvim_lsp#setup("texlab", {config}) Commands: - TexlabBuild: Build the current buffer Default Values: cmd = { "texlab" } filetypes = { "tex", "bib" } log_level = 2 root_dir = vim's starting directory settings = { bibtex = { formatting = { lineLength = 120 } }, latex = { build = { args = { "-pdf", "-interaction=nonstopmode", "-synctex=1" }, executable = "latexmk", onSave = false }, forwardSearch = { args = {}, onSave = false }, lint = { onChange = false } } }
tsserver
https://github.com/theia-ide/typescript-language-server
typescript-language-server
can be installed via :LspInstall tsserver
or by yourself with npm
:
npm install -g typescript-language-server
Can be installed in neovim with :LspInstall tsserver
nvim_lsp.tsserver.setup({config}) nvim_lsp#setup("tsserver", {config}) Default Values: capabilities = default capabilities, with offsetEncoding utf-8 cmd = { "typescript-language-server", "--stdio" } filetypes = { "javascript", "javascriptreact", "javascript.jsx", "typescript", "typescriptreact", "typescript.tsx" } log_level = 2 on_init = function to handle changing offsetEncoding root_dir = root_pattern("package.json") settings = {}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK