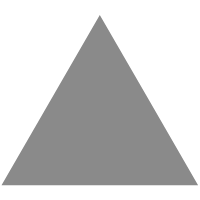
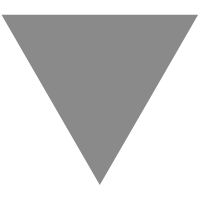
Building a Countdown Timer in JavaScript
source link: https://alligator.io/js/building-countdown-timer/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Countdown timers can serve many purposes. They can communicate to a user how long they’ve been doing something or how much time until some event happens, like the launch of your new website.
In the sales and marketing context they can be used to create a sense of urgency to encourage the conversion. FOMO can be hard to beat, but building a countdown timer in pure JavaScript is easy!
Getting Started
Since we’re leveraging the power of JavaScript in it’s purest form, without any front-end libraries, there’s not a ton of bootstrapping that has to be done.
All we need is a .html
file with a <div>
to inject the countdown timer’s output into and a <script>
block that will house our code:
<!DOCTYPE html> <html> <body> <div id="countdown"></div> <script> // This is where our sweet timer code will go! </script> </body> </html>
Calculating the Time Remaining
To calculate the time remaining, we need to find the difference between the current time and the time that our countdown timer will expire. One of the more notable times that we countdown to is New Year’s Day, so we’ll use the upcoming new year as our end time:
const difference = +new Date("2020-01-01") - +new Date();
The +
before the new Date
is shorthand to tell JavaScript to cast the object as an integer, which gives us the object’s Unix time stamp represented as microseconds since the epoch.
Formatting to Days, Hours, Minutes and Seconds
Now that we know the total number of milliseconds until the countdown time expires, we need to convert the number of milliseconds to something a bit more friendly and human readable.
We’re going to calculate the total number of hours, minutes and of course, seconds, by doing some math and using the modulus %
operator:
const parts = { days: Math.floor(difference / (1000 * 60 * 60 * 24)), hours: Math.floor((difference / (1000 * 60 * 60)) % 24), minutes: Math.floor((difference / 1000 / 60) % 60), seconds: Math.floor((difference / 1000) % 60), };
By rounding the numbers down, we’re able to drop the remainder to get the whole number value.
With an object full of the days, hours, minutes and seconds remaining, we can put things together into a string:
const remaining = Object.keys(parts) .map((part) => { if (!parts[part]) return; return `${parts[part]} ${part}`; }) .join(" ");
Show the Time Remaining on the Page
With the time parts assembled into a string, we can update our <div>
to contain the value:
document.getElementById("countdown").innerHTML = remaining;
Automatically Updating the Timer
Thus far, we’ve calculated the time difference between the current time and the time that our countdown expires. We’ve broken that time into hours, minutes and seconds, and then updated the page with the time remaining.
Then time stood still.
Without additional logic to update the page periodically, the timer is stuck in place until the next time the page is loaded. Without an update, it’s hard to even describe it as a timer.
No big deal, we can easily create an interval to run every second (or 1000ms) and update the timer’s display:
setInterval(() => { // This is where we'd recalculate the time remaining }, 1000);
Putting it All Together
Obviously that last example was a bit lacking. That’s because it’s time to put it all together into something usable, and even add in some additional niceties like updating the timer on page load (instead of waiting for the first run of the interval) and handling things when the timer has expired:
<!DOCTYPE html> <html> <body> <div id="countdown"></div> <script> function countdownTimer() { const difference = +new Date("2020-01-01") - +new Date(); let remaining = "Time's up!"; if (difference > 0) { const parts = { days: Math.floor(difference / (1000 * 60 * 60 * 24)), hours: Math.floor((difference / (1000 * 60 * 60)) % 24), minutes: Math.floor((difference / 1000 / 60) % 60), seconds: Math.floor((difference / 1000) % 60) }; remaining = Object.keys(parts) .map(part => { if (!parts[part]) return; return `${parts[part]} ${part}`; }) .join(" "); } document.getElementById("countdown").innerHTML = remaining; } countdownTimer(); setInterval(countdownTimer, 1000); </script> </body> </html>
You can check a working example of this code over on CodeSandbox .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK