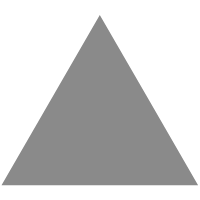
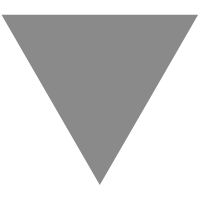
你可能不知道的 JSON.stringify 用法 - 码力全开 - SegmentFault 思否
source link: https://segmentfault.com/a/1190000020872405
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
你可能不知道的 JSON.stringify 用法
JS 中有许多常见的函数,我们可能每天都在使用它们,但是却不知道它们的一些额外功能。JSON.stringify
就是这样的一个函数,今天就来看下它的特殊用法。
JSON.stringify
方法接收一个变量,并将它转换成 JSON 表示形式。
const boy = {
name: 'John',
age: 23
};
JSON.stringify(boy);
// {"name":"John","age":23}
JSON 就是纯字符串,它本质上是 JS 的一个子集,所以并不是所有的 JS 对象都能转换为 JSON:
const boy = {
name: 'John',
age: 23,
hobbies: new Map([[0, 'coding'], [1, 'earn money']])
}
JSON.stringify(boy)
// {"name":"John","age":23,"hobbies":{}}
上面的例子中 Map 对象就会被忽略并转换为普通对象。而如果属性是函数的话则这个属性就会被忽略,感兴趣的同学可以试下。
第二个参数
JSON.stringify
可以接收第二个参数,可以称为 replacer
替换器。
你可以传入一个字符串数组,这个数组中具有的属性才会被转换,就像一个白名单。
const boy = {
name: 'John',
age: 23
}
JSON.stringify(boy, ['name'])
// {"name":"John"}
我们可以利用这个特性,只转换需要转换的属性,过滤掉如很长的数组、错误对象等。
这个 replacer
参数还可以接收一个函数。这个函数会遍历整个对象,并将键和值传入,让你决定该如何替换它们。
const boy = {
name: 'John',
age: 23,
hobbies: new Map([[0, 'coding'], [1, 'earn money']])
}
JSON.stringify(boy, (key, value) => {
if (value instanceof Map) {
return [...value.values()]
}
return value
})
// {"name":"John","age":23,"hobbies":["coding","earn money"]}
而如果你返回了 undefined
(返回 null
不行),就将这个属性移除了:
JSON.stringify(boy, (key, value) => {
if (typeof value === 'string') {
return undefined
}
return value
})
// {"age":23,"hobbies":{}}
第三个参数
第三个参数 space
控制了转换后的 JSON 串的间距。
如果参数是数字,则以该数字个数的空格进行缩进:
JSON.stringify(boy, null, 2)
// {
// "name": "John",
// "age": 23,
// "hobbies": {}
// }
而如果参数是字符串,则以该字符串进行缩进:
JSON.stringify(boy, null, '--')
// {
// --"name": "John",
// --"age": 23,
// --"hobbies": {}
// }
toJSON 方法
如果我们要转换的对象具有一个 toJSON
方法,那么就可以定制自己被序列化的过程。你甚至可以返回一个新的对象。
const boy = {
name: 'John',
age: 23,
hobbies: new Map([[0, 'coding'], [1, 'earn money']]),
toJSON() {
return {
name: `${this.name} (${this.age})`,
favorite: this.hobbies.get(0)
}
}
}
JSON.stringify(boy)
// {"name":"John (23)","favorite":"coding"}
是不是很有趣?有时候仔细看一些文档还是很有用的~
欢迎关注我的公众号:码力全开(codingonfire)
每周更新一篇原创或翻译文章~
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK