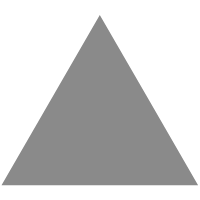
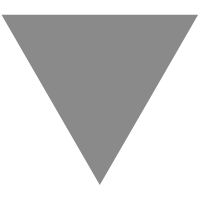
你应该学习的20个Python代码段-InfoQ
source link: https://www.infoq.cn/article/tzyzWOWUWFpX3ieByYbg
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python 是一门优秀的编程语言。 可读性和设计简单性是其广受欢迎的两个主要原因。
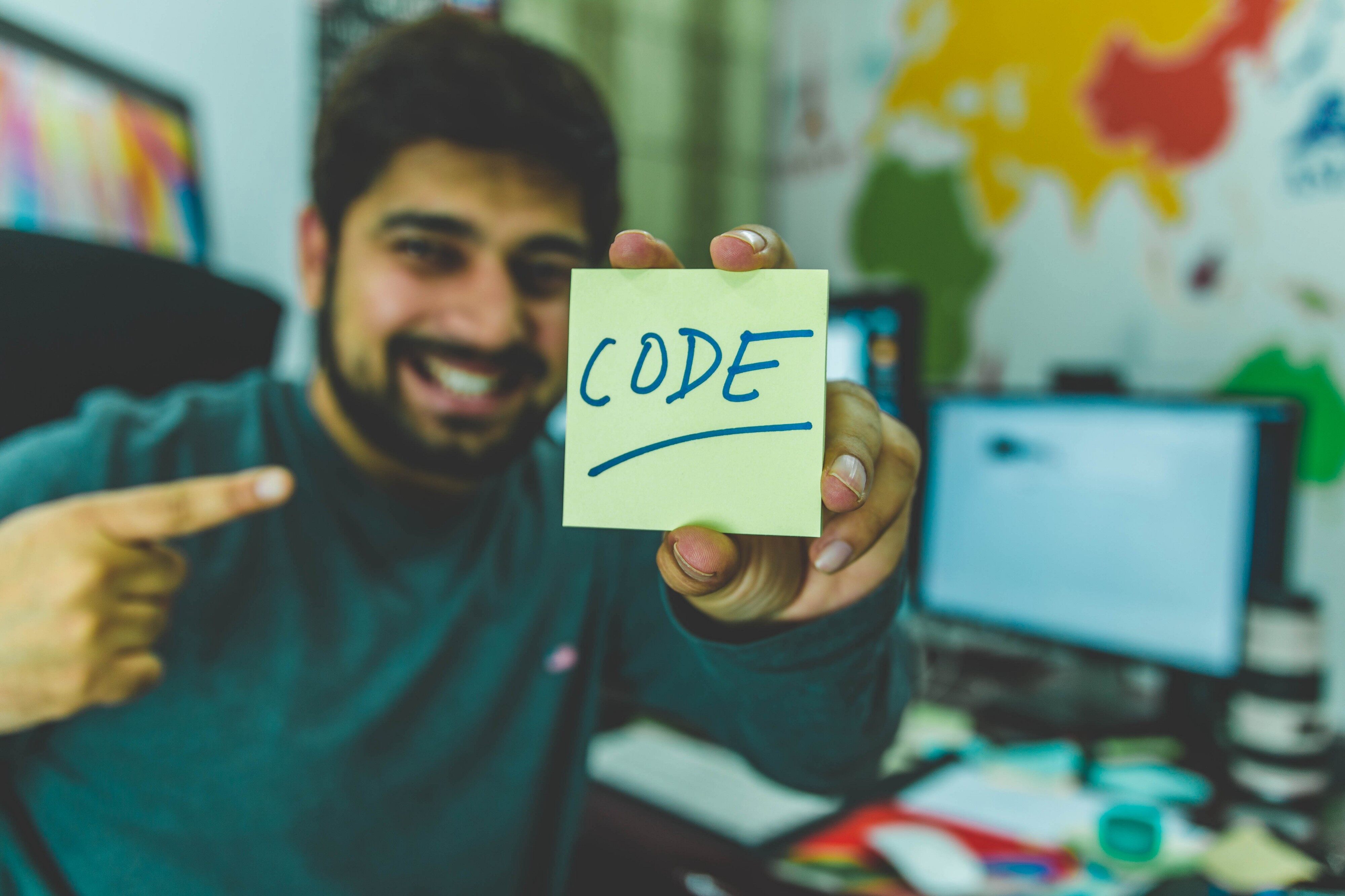
正如 Python 的禅宗所说:
美丽胜于丑陋。
明了胜于晦涩。
这就是为什么值得记住一些常见的 Python 技巧,可以帮助改善代码设计, 并为您节省大量时间。
在您的日常编码中,以下技巧将非常有用。
1. 字符串倒转
以下代码段使用 Python 切片操作反转字符串。
# Reversing a string using slicing
my_string = "ABCDE"
reversed_string = my_string[::-1]
print(reversed_string)
# Output
# EDCBA
2. 首字母大写
以下代码段可用于将字符串转换为标题首字母大写。 这是使用 title()方法完成的。
my_string = "my name is chaitanya baweja"
# using the title() function of string class
new_string = my_string.title()
print(new_string)
# Output
# My Name Is Chaitanya Baweja
3. 在字符串中查找唯一元素
以下代码段可用于查找字符串中所有的唯一元素。 我们使用集合中唯一元素。
my_string = "aavvccccddddeee"
# converting the string to a set
temp_set = set(my_string)
# stitching set into a string using join
new_string = ''.join(temp_set)
print(new_string)
4.n 次打印字符串或列表
您可以对字符串或列表使用乘法(*)。 我们可以将它们任意复制。
n = 3 # number of repetitions
my_string = "abcd"
my_list = [1,2,3]
print(my_string*n)
# abcdabcdabcd
print(my_list*n)
# [1,2,3,1,2,3,1,2,3]
一个有趣的用例是定义一个具有恒定值的列表——假设为零。
my_list = [0]*n # n denotes the length of the required list
# [0, 0, 0, 0]
5. 列表推导式
列表推导式为我们提供了一种在其他列表基础上创建列表的好方法。
以下代码段通过将旧列表的每个元素乘以 2 来创建新列表。
# Multiplying each element in a list by 2
original_list = [1,2,3,4]
new_list = [2*x for x in original_list]
print(new_list)
# [2,4,6,8]
6. 变量交换
Python 在两个变量之间交换值而不使用另一个变量变得非常简单。
a, b = b, a
print(a) # 2
print(b) # 1
7. 将字符串拆分为子字符串列表
我们可以使用字符串类中的.split()方法将字符串拆分为子字符串列表,还可以将要分割的分隔符作为参数传递。
string_1 = "My name is Chaitanya Baweja"
string_2 = "sample/ string 2"
# default separator ' '
print(string_1.split())
# ['My', 'name', 'is', 'Chaitanya', 'Baweja']
# defining separator as '/'
print(string_2.split('/'))
# ['sample', ' string 2']
8. 将字符串列表组合成单个字符串
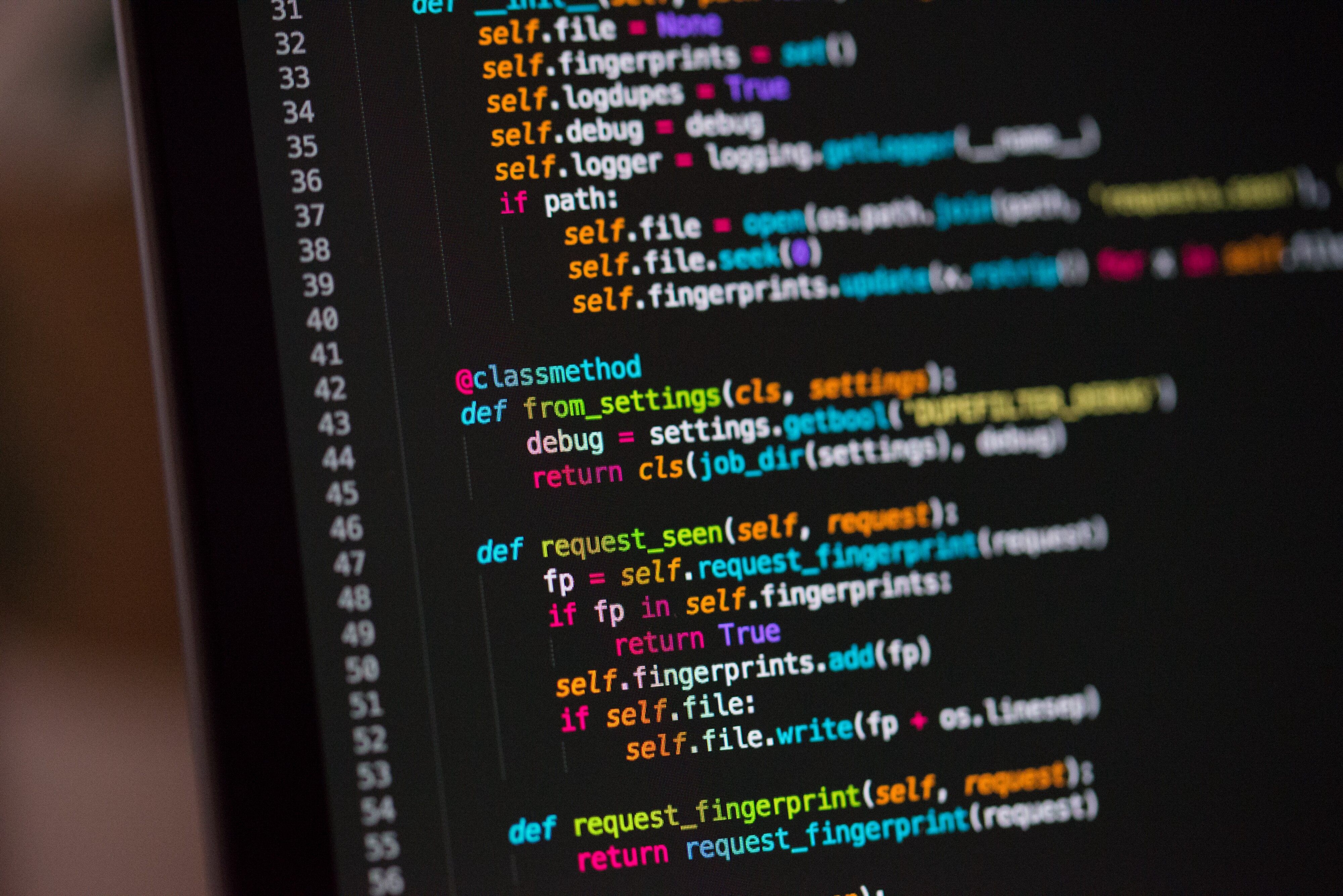
join()将作为参数传递的字符串列表组合为单个字符串。这种情况下,我们使用逗号分隔符将它们分开。
list_of_strings = ['My', 'name', 'is', 'Chaitanya', 'Baweja']
# Using join with the comma separator
print(','.join(list_of_strings))
# Output
# My,name,is,Chaitanya,Baweja
9. 检查回文字符串
我们已经讨论过如何反转字符串,因此回文字符串成为 Python 中一个简单的程序。
my_string = "abcba"
if my_string == my_string[::-1]:
print("palindrome")
else:
print("not palindrome")
# Output
# palindrom
10. 列表中的元素统计
这样做有多种方法,但是我最喜欢的是使用 Python Counter 类。
Python 计数器跟踪容器中每个元素的频率, Counter()返回一个字典,其中元素作为键,频率作为值。
我们还使用 most_common()函数来获取列表中的 most_frequent 元素。
# finding frequency of each element in a list
from collections import Counter
my_list = ['a','a','b','b','b','c','d','d','d','d','d']
count = Counter(my_list) # defining a counter object
print(count) # Of all elements
# Counter({'d': 5, 'b': 3, 'a': 2, 'c': 1})
print(count['b']) # of individual element
print(count.most_common(1)) # most frequent element
# [('d', 5)]
11. 查找两个字符串是否为字母
Counter 类的一个有趣应用是查找字谜。
字谜是通过重新排列不同单词或短语的字母而形成的单词或短语。
如果两个字符串的 Counter 对象相等,那么它们就是字谜。
from collections import Counter
str_1, str_2, str_3 = "acbde", "abced", "abcda"
cnt_1, cnt_2, cnt_3 = Counter(str_1), Counter(str_2), Counter(str_3)
if cnt_1 == cnt_2:
print('1 and 2 anagram')
if cnt_1 == cnt_3:
print('1 and 3 anagram')
12. 使用 try-except-else 块
使用 try / except 块可以轻松完成 Python 中的错误处理,向该块添加 else 语句可能会很有用,在 try 块中没有引发异常的情况下运行。
如果您需要运行某些程序,无需考虑异常,请继续使用。
a, b = 1,0
print(a/b)
# exception raised when b is 0
except ZeroDivisionError:
print("division by zero")
else:
print("no exceptions raised")
finally:
print("Run this always"
13. 使用枚举获取索引 / 值对
以下脚本使用枚举遍历列表中的值及其索引。
my_list = ['a', 'b', 'c', 'd', 'e']
for index, value in enumerate(my_list):
print('{0}: {1}'.format(index, value))
14. 检查对象的内存使用情况
以下脚本可用于检查对象的内存使用情况, 这里可以了解更多信息。
import sys
num = 21
print(sys.getsizeof(num))
# In Python 2, 24
# In Python 3, 28
15. 合并两个字典
在 Python 2 中,我们使用了 update()方法来合并两个字典, Python 3.5 使这一过程变得更加简单。
在下面给出的脚本中,两个字典被合并。 在相交的情况下,使用第二个字典中的值。
dict_1 = {'apple': 9, 'banana': 6}
dict_2 = {'banana': 4, 'orange': 8}
combined_dict = {**dict_1, **dict_2}
print(combined_dict)
# Output
# {'apple': 9, 'banana': 4, 'orange': 8}
16. 执行一段代码所需的时间
以下代码片段使用库函数来计算执行代码所需的时间消耗。
import time
start_time = time.time()
# Code to check follows
a, b = 1,2
c = a+ b
# Code to check ends
end_time = time.time()
time_taken_in_micro = (end_time- start_time)*(10**6)
print(" Time taken in micro_seconds: {0} ms").format(time_taken_in_micro)
17. 展平列表清单
有时,您不确定列表的嵌套深度,只希望将所有元素放在一个平面列表中。
您可以通过以下方式获得该信息:
from iteration_utilities import deepflatten
# if you only have one depth nested_list, use this
def flatten(l):
return [item for sublist in l for item in sublist]
l = [[1,2,3],[3]]
print(flatten(l))
# [1, 2, 3, 3]
# if you don't know how deep the list is nested
l = [[1,2,3],[4,[5],[6,7]],[8,[9,[10]]]]
print(list(deepflatten(l, depth=3)))
# [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
如果您具有正确格式化的数组,那 Numpy Flatten 是执行此操作的更好方法。
18. 从列表中随机取样
以下代码段使用随机库从给定列表中生成了 n 个随机样本。
import random
my_list = ['a', 'b', 'c', 'd', 'e']
num_samples = 2
samples = random.sample(my_list,num_samples)
print(samples)
# [ 'a', 'e'] this will have any 2 random values
我一直推荐秘密库生成随机样本进行加密, 以下代码段仅适用于 Python 3。
import secrets # imports secure module.
secure_random = secrets.SystemRandom() # creates a secure random object.
my_list = ['a','b','c','d','e']
num_samples = 2
samples = secure_random.sample(my_list, num_samples)
print(samples)
# [ 'e', 'd'] this will have any 2 random values
19. 数字化
以下代码段会将整数转换为数字列表。
num = 123456
# using map
list_of_digits = list(map(int, str(num)))
print(list_of_digits)
# [1, 2, 3, 4, 5, 6]
# using list comprehension
list_of_digits = [int(x) for x in str(num)]
print(list_of_digits)
# [1, 2, 3, 4, 5, 6]
20. 检查唯一性
以下函数将检查列表中的所有元素是否唯一。
def unique(l):
if len(l)==len(set(l)):
print("All elements are unique")
else:
print("List has duplicates")
unique([1,2,3,4])
# All elements are unique
unique([1,1,2,3])
# List has duplicates
这些都是一些代码简短片段,我觉得在日常工作是非常有用的,使用 30 秒来试试吧。
感谢您的阅读, 希望你喜欢。
英文原文:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK