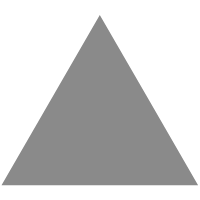
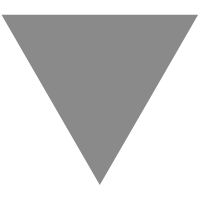
RealmQL
source link: https://www.tuicool.com/articles/ZJ32Evy
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
RealmQL
RealmQL is a hobby project, it is not meant to do anything more than a query API but I would be glad if you could contribute anything new to it.
RealmQL uses the Realm database as its back-end for managing the models and queries, the schema's are pre-defined and can be linked to eachother.
Express is used for the request/response parsing and data exchange.
Schema and commands
CREATE
Creates a schema model with the provided values
{ "Person": { "firstName": "John", "lastName" : "Doe" } }
Creates a schema model with a nested model, first you need to create the object, which will be linked to this one
{ "License": { "privateId": "9999999999" } }
And then link it to the person
{ "Person": { "firstName": "John", "lastName" : "Doe", "license": {"id": 1} } }
READ
Reads the schema model for specific values and returns it as an object
{ "Person": { "id": 1, "values": ["firstName", "lastName"] } }
Reads the schema model for values and returns it as an object
{ "Person": { "id": 1, "values": ["*"] } }
Reads the schema model for specific nested model values and returns it as an object
{ "Person": { "id": 1, "values": [ "*", {"license": ["privateId"]} ] } }
Reads the schema model for nested model values and returns it as an object
{ "Person": { "id": 1, "values": [ "*", {"license": ["*"]} ] } }
UPDATE
Updates the schema model with the provided values
{ "Person": { "id": 1, "values": { "firstName": "Joseph", "lastName": "Momma" } } }
DELETE
Deletes the schema model
{ "Person": { "id": 1, "onDelete": {} } }
The onDelete function will contain funtionality similar to the Django ORM, for example:
{ "Person": { "id": 1, "onDelete": { "CASCADE": { "objectReferences": [] }, "PROTECT": { "objectReferences": [] }, "SET_NULL": { "objectReferences": [] } } } }
Installation
$REPOSITORY - Current repository
git clone $REPOSITORY # Clone the git repository cd $REPOSITORY npm i # Install the required dependencies npm start # Start the project using nodemon
Usage
Using with Express.js
const express = require('express') const realmql = require('realmql') const bodyParser = require('body-parser') /* Schemas are from Realm DB, head over to the documentation for more details Additional resources: https://realm.io/docs/javascript/latest#models */ const ExampleSchema = { name: 'Example', primaryKey: 'id', properties: { id: 'int', property: 'string', } } const app = express() /* Set the schema array in the app constant so the RealmQL parser can use it keep in mind that the schema must be contained in an array and not a single object. */ app.set('schema', [ExampleSchema]) /* Use the body-parser package since it is the easiest way of extracting POST data */ app.use(bodyParser.json()) /* Use the RealmQL in the express stack for the /realmql endpoint */ app.use(realmql) app.listen(3000, ()=> { console.log("Listening at port 3000 !") })
License
MIT License
Copyright (c) 2019 Giorgi Kavrelishvili
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK