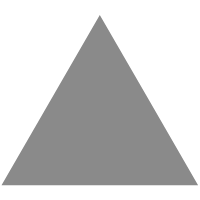
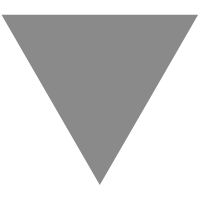
Springboot】Springboot整合邮件服务(HTML/附件/模板-QQ、网易)
source link: https://www.tuicool.com/articles/I3AV3uY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
介绍
邮件服务是常用的服务之一,作用很多,对外可以给用户发送活动、营销广告等;对内可以发送系统监控报告与告警。
本文将介绍Springboot如何整合邮件服务,并给出不同邮件服务商的整合配置。
如图所示:

Springboot整合邮件服务
开发过程
Springboot搭建
Springboot的搭建非常简单,我们使用 Spring Initializr 来构建,十分方便,选择需要用到的模块,就能快速完成项目的搭建:

Spring Initializr
引入依赖
为了使用邮件服务,我们需要引入相关的依赖,对于Springboot加入下面的依赖即可:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency>
配置文件
需要配置邮件服务提供商的相关参数,如服务地址、用户名及密码等。下面的例子是QQ的配置,其中密码并不是QQ密码,而是QQ授权码,后续我们再讲怎么获得。
Springboot的配置文件 application.yml
如下:
server: port: 8080 spring: profiles: active: qq --- spring: profiles: qq mail: host: smtp.qq.com username: [email protected] password: xxx properties: mail: smtp: auth: true starttls: enable: true required: true --- spring: profiles: netEase mail: host: smtp.163.com username: [email protected] password: xxx properties: mail: smtp: auth: true starttls: enable: true required: true
实现发送服务
将JavaMailSender注入,组装Message后,就可以发送最简单的文本邮件了。
@Autowired private JavaMailSender emailSender; public void sendNormalText(String from, String to, String subject, String text) { SimpleMailMessage message = new SimpleMailMessage(); message.setFrom(from); message.setTo(to); message.setSubject(subject); message.setText(text); emailSender.send(message); }
调用接口
服务调用实现后,通过Controller对外暴露REST接口,具体代码如下:
@Value("${spring.mail.username}") private String username; @Autowired private MailService mailService; @GetMapping("/normalText") public Mono<String> sendNormalText() { mailService.sendNormalText(username, username, "Springboot Mail(Normal Text)", "This is a mail from Springboot!"); return Mono.just("sent"); }
把实现的 MailService
注入到Controller里,调用对应的方法即可。本次的邮件发送人和收件人都是同一个帐户,实际实现可以灵活配置。
通过Postman调用接口来测试一下能不能正常发送:

Postman
成功返回"sent",并收到了邮件,测试通过。
多种类型邮件
简单文本邮件
简单文本邮件如何发送,刚刚已经讲解,不再赘述。
HTML邮件
纯文本虽然已经能满足很多需求,但很多时候也需要更加丰富的样式来提高邮件的表现力。这时HTML类型的邮件就非常有用。
Service代码如下:
public void sendHtml(String from, String to, String subject, String text) throws MessagingException { MimeMessage message = emailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(text, true); emailSender.send(message); }
与简单的文本不同的是,本次用到了 MimeMessage
和 MimeMessageHelper
,这是非常有用的类,后续我们经常会用到,组合使用能大大丰富邮件表现形式。
Controller的代码如下:
@GetMapping("/html") public Mono<String> sendHtml() throws MessagingException { mailService.sendHtml(username, username, "Springboot Mail(HTML)", "<h1>This is a mail from Springboot!</h1>"); return Mono.just("sent"); }
带附件邮件
邮件发送文件再正常不过,发送附件需要使用 MimeMessageHelper.addAttachment(String attachmentFilename, InputStreamSource inputStreamSource)
方法,第一个参数为附件名,第二参数为文件流资源。Service代码如下:
public void sendAttachment(String from, String to, String subject, String text, String filePath) throws MessagingException { MimeMessage message = emailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(text, true); FileSystemResource file = new FileSystemResource(new File(filePath)); helper.addAttachment(filePath, file); emailSender.send(message); }
Controller代码如下:
@GetMapping("/attachment") public Mono<String> sendAttachment() throws MessagingException { mailService.sendAttachment(username, username, "Springboot Mail(Attachment)", "<h1>Please check the attachment!</h1>", "/Pictures/postman.png"); return Mono.just("sent"); }
带静态资源邮件
我们访问的网页其实也是一个HTML,是可以带很多静态资源的,如图片、视频等。Service代码如下:
public void sendStaticResource(String from, String to, String subject, String text, String filePath, String contentId) throws MessagingException { MimeMessage message = emailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(text, true); FileSystemResource file = new FileSystemResource(new File(filePath)); helper.addInline(contentId, file); emailSender.send(message); }
其中, contentId
为HTML里静态资源的ID,需要对应好。
Controller代码如下:
@GetMapping("/inlinePicture") public Mono<String> sendStaticResource() throws MessagingException { mailService.sendStaticResource(username, username, "Springboot Mail(Static Resource)", "<html><body>With inline picture<img src='cid:picture' /></body></html>", "/Pictures/postman.png", "picture"); return Mono.just("sent"); }
模板邮件
Java的模板引擎很多,著名的有Freemarker、Thymeleaf、Velocity等,这不是本点的重点,所以只以Freemarker为例使用。
Service代码如下:
@Autowired private FreeMarkerConfigurer freeMarkerConfigurer; public void sendTemplateFreemarker(String from, String to, String subject, Map<String, Object> model, String templateFile) throws Exception { MimeMessage message = emailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); Template template = freeMarkerConfigurer.getConfiguration().getTemplate(templateFile); String html = FreeMarkerTemplateUtils.processTemplateIntoString(template, model); helper.setText(html, true); emailSender.send(message); }
注意需要注入 FreeMarkerConfigurer
,然后使用 FreeMarkerTemplateUtils
解析模板,返回 String
,就可以作为内容发送了。
Controller代码如下:
@GetMapping("/template") public Mono<String> sendTemplateFreemarker() throws Exception { Map<String, Object> model = new HashMap<>(); model.put("username", username); model.put("templateType", "Freemarker"); mailService.sendTemplateFreemarker(username, username, "Springboot Mail(Template)", model, "template.html"); return Mono.just("sent"); }
注意模板文件 template.html
要放在resources/templates/目录下面,这样才能找得到。
模板内容如下:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <h1>Hello ${username}</h1> <h1>This is a mail from Springboot using ${templateType}</h1> </body> </html>
其中 ${username}
和 ${templateType}
为需要替换的变量名,Freemarker提供了很多丰富的变量表达式,这里不展开讲了。
集成不同邮件服务商
邮件服务的提供商很多,国内最常用的应该是QQ邮箱和网易163邮箱了。
集成QQ邮件需要有必备的账号,还要开通授权码。开通授权码后配置一下就可以使用了,官方的文档如下:
需要注意的是,开通授权码是需要使用绑定的手机号发短信到特定号码的,如果没有绑定手机或者绑定手机不可用,那都会影响开通。
开通之后,授权码就要以作为密码配置到文件中。
163
网易的开通方式与QQ没有太大差别,具体的指导可以看如下官方文档:
同样也是需要绑定手机进行操作。
总结
本次例子发送后收到邮件如图所示:

邮件
邮件功能强大,Springboot也非常容易整合。技术利器,善用而不滥用。
欢迎关注公众号<南瓜慢说>,将为你持续更新...
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK