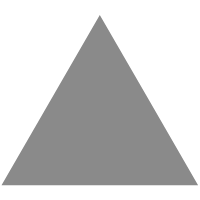
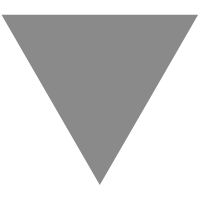
Pixi.js: Programming games with JavaScript
source link: https://www.tuicool.com/articles/UvE36n3
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article, I will show you pixi.js, a super fast rending tool, also a swiss-army-knife tool with a friendly API.
What is it?
Pixi.js is a display tool that allows you to use the power of WebGL and canvas to render the content you want on the screen continuously.
In fact, pixi.js includes WebGL and a canvas renderer, which can go back to the mode for lower-end devices. You can harness the power of WebGL and hardware-accelerated graphics on devices powerful enough to use it.
If one of the users is using an older device, the engine will automatically return to the canvas renderer and no differences will occur, so you don’t have to worry about this.
WebGL for 2D?
If you’ve heard or experienced a web product introduced using WebGL, you might have the impression of 3D games, 3D images of the earth, or something like that.
WebGL was initially marked and launched to the community for rendering 3D graphics on the browser, because it was the only way fast enough to allow them to do so. But technology is not primarily 3D, nor is it 2D, but you make it work the way you want. So the main idea behind in pixi.js is to provide speed and quality for rendering 2D graphics and games, and of course for the general community.
You can assume that I don’t need this fine-grain control and precision for 2D, and the WebGL API can be part of a 2D application, but with browsers becoming more powerful, the expectations of Users are getting taller and this technology with its speed allows you to compete with flash-only applications.
overview
Pixi.js was created by an old flash developer, so its syntax is very similar to ActionScript3.
This is a small guide to the important components that you need to create when using pixi.
The renderer
I have provided you with descriptions of its features and capabilities. So the only thing to remember is that there are two ways to create a renderer. You can specify the renderer you want or let the engine decide on the current device.
// When you let the engine decide : var renderer = PIXI.autoDetectRenderer(800,600); // When you specifically want one or the other renderer: var renderer = new PIXI.WebGLRenderer(800,600); // and for canvas you'd write : // var renderer = new PIXI.WebGLRenderer(800,600);
The stage
Pixi learns according to the Flash API in dealing with the position of the audience. Basically, the object’s coordinates are always relative to the parent’s container .
Flash and pixi allow you to create special objects called containers. They are not images or graphics, they are abstract ways to group objects together.
Suppose you have a landscape made of many different things like trees, rocks, and so on. If you add them to a container and move this container, you can move all of these objects by moving the container.
Here’s how it works:

Don’t worry if it’s hard to understand, here’s how the stage is mentioned. The stage is the root repository where everything is added.
The stage is not for moving, so when a spruce is added directly to the stage, it is possible to make sure its position is the same as its position on the screen (in the canvas you use).
// here is how you create a stage var stage = new PIXI.Stage();
Try doing something
Ok, enough theory for scene-graph, this is the time we will practice doing something.
As I wrote earlier, pixi is a rendering tool, so you will need to give the renderer its render stage, otherwise nothing will happen. So, this is the “main skeleton” you use for anything related to pixi:
// create an new instance of a pixi stage var stage = new PIXI.Stage(0x0212223); // create a renderer instance var renderer = PIXI.autoDetectRenderer(window.innerWidth, window.innerHeight); // add the renderer view element to the DOM document.body.appendChild(renderer.view); // create a new Sprite using the texture var bunny = new PIXI.Sprite.fromImage("assets/bunny.png"); bunny.position.set(200,230); stage.addChild(bunny); animate(); function animate() { // render the stage renderer.render(stage); requestAnimFrame(animate); }
First, you create a renderer and a stage, like I mentioned above. Then you create the main pixi object, a Sprite, for example, basically an image that is displayed on the screen.
var sprite = new PIXI.Sprite.fromImage("assets/image.png");
Sprites, are the core of the game, and are the ones you use the most in pixi and any game framework.
However, the pixi is not really a game framework , but at a lower level, you need to manually add the sprite to the stage. So, whenever something is not visible, be sure to check that you have added it to the following stage: stage.addChild(sprite);
stage.addChild(sprite);
Then you can write a function that initializes some sprites.
function createParticles () { for (var i = 0; i < 40; i++) { // create a new Sprite using the texture var bunny = new PIXI.Sprite.fromImage("assets/bunny.png"); bunny.xSpeed = (Math.random()*20)-10; bunny.ySpeed = (Math.random()*20)-10; bunny.tint = Math.random() * 0xffffff; bunny.rotation = Math.random() * 6; stage.addChild(bunny); } }
Then take advantage of the loops to update moving the surrounding sprites randomly:
if(count > 10){ createParticles(); count = 0; } if(stage.children.length > 20000){ stage.children.shift()} for (var i = 0; i < stage.children.length; i++) { var sprite = stage.children[i]; sprite.position.x += sprite.xSpeed; sprite.position.y += sprite.ySpeed; if(sprite.position.x > renderer.width){ sprite.position.x = 0; } if(sprite.position.y > renderer.height){ sprite.position.y = 0; } }; </code>
So that’s very simple. You can create your own simple game as you like.
Source: https://techtalk.vn/lap-trinh-game-voi-pixi-js.html
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK