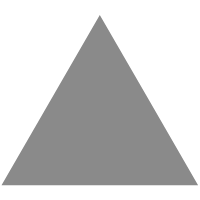
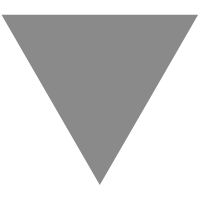
GitHub - jogboms/time.dart: ⏰ Type-safe DateTime and Duration calculations, powe...
source link: https://github.com/jogboms/time.dart
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Time
With shiny extensions, if you have ever written something like this, then look no further:
final DateTime fourHoursFromNow = DateTime.now() + Duration(hours: 4);
Installation
dependencies: time: "^1.3.0"
Import
import 'package:time/time.dart';
Usage
final Duration tenMinutes = 10.minutes; final Duration oneHourThirtyMinutes = 1.5.hours; final DateTime afterTenMinutes = DateTime.now() + 10.minutes; final Duration tenMinutesAndSome = 10.minutes + 15.seconds; final int tenMinutesInSeconds = 10.minutes.inSeconds; final DateTime tenMinutesFromNow = 10.minutes.fromNow;
You can perform all basic arithmetic operations on Duration
as you always have been:
final Duration interval = 10.minutes + 15.seconds - 3.minutes + 2.hours; final Duration doubled = interval * 2;
You can also use these operations on DateTime
:
final DateTime oneHourAfter = DateTime() + 1.hours;
Duration
is easily convertible as it always has been:
final int twoMinutesInSeconds = 2.minutes.inSeconds;
You can also convert Duration
to DateTime
, if needed:
final DateTime timeInFuture = 5.minutes.fromNow; final DateTime timeInPast = 5.minutes.ago;
Iterate through a DateTime
range:
final DateTime start = DateTime(2019, 12, 2); final DateTime end = start + 1.weeks; final DateTime tuesday = start.to(end).firstWhere((date) => date.weekday == DateTime.tuesday);
You can also delay code execution:
void doSomething() async { await 5.seconds.delay; // Do the other things }
You can also use the popular copyWith
:
final initial = DateTime(2019, 2, 4, 24, 50, 45, 1, 1); final expected = initial.copyWith( year: 2021, month: 10, day: 28, hour: 12, minute: 45, second: 10, millisecond: 0, microsecond: 12, );
Bugs/Requests
If you encounter any problems feel free to open an issue. If you feel the library is missing a feature, please raise a ticket on Github and I'll look into it. Pull request are also welcome.
Inspiration
License
MIT License
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK