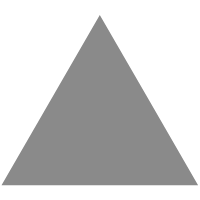
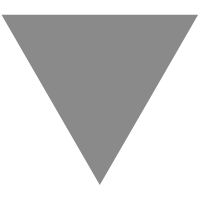
Spruce up your GR visualizations in Julia
source link: https://www.tuicool.com/articles/f2Qv6vj
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Spruce up your GR visualizations in Julia

Oct 12 ·6min read
That’s right, it’s Julia again, I promise R, Scala, and Python are still in this love-circle
Plots.JL is such a diverse package when it comes to visualization in Julia. Although most of the back-ends use Python, using Plots.jl is comprable to using Plotly, Matplotlib(PyPlot), and even more tools inside of one package. For a full list of backends including Python-based backends, as well as Julia, C, and even LaTex backends for Plots.jl, it can be found here.
All of these back-ends are useful for the use-case that they’re applied to. The GR back-end is typically concerned with quick, light, and easy visualizations with minimal parameters to set to alter your plot. That being said, it is still a very useful tool. In fact, you may frequently see me using GR for visualizations when working with Julia. As always, the notebook is here.
Ensure you’re on the GR back-end
Although it feels relatively rudimentary, this will return your selected back-end:
using Plots # Using function we can return what backend we are in: println("Current Plots.jl Backend:") Plots.backend()
And if it isn’t GR, you can set it to GR by doing:
Plots.gr()
The Basics
As always, there are some (almost) universal parameters that will apply to every type of plot, like background color, grid-lines, xticks, yticks, labels, etc. Here’s a fairly basic scatter-plot with some of the available parameters:
scatter(x,y, # title: title = "GR Scatter Plot", # X label: xlabel = "Random data", # Y label ylabel = "Random data", # Grid: grid = false, # Legend position legend=:bottomright, # Color of the marker color = :lightblue, # Marker stroke width and opacity markerstrokewidth = 4, markerstrokealpha = .75, # Marker stroke color markerstrokecolor = :lightblue, # Adjust out y ticks yticks = [20,40,50], # Our font options fontfamily = :Courier,xtickfontsize=7,ytickfontsize=9, ytickfont=:Courier,xtickfont = :Courier,titlefontsize=13, # We can also add annotations, super easily: )
Title
Of course, the title is pretty simple, just set title equal to a string, relatively straight forward. We can also change the title’s font using
titlefont = :FontName titlefontsize = fontsize
We can also set the font globally, of course I prefer to keep them in a single instance, but it may be useful for some:
gr(tickfont=font("serif"), titlefont=font("serif"))
Grid and legend
To remove the grid, which is enabled by default, we just add
grid = false
into our parameters. The legend’s position is a bit more tricky, as there are only preset positions that we can put it into. For example, here I use :bottom-right. I don’t deem it necessary to elaborate because the other positions are relative to the syntax of that.
Additionally, changing the text in the legend is relatively straight forward,
label="Sample"
X — Label and Ticks, Y — Label and Ticks
In order to set the label = to a string, it’s as simple as:
xlabel = "MyLabel" ylabel = "MyLabel"
The ticks list must be in the range of the X and Y, of course, and is taken into the parameters as an array.
yticks = [20,40,50]
Marker Shape, Color, Size, and Alpha
Note:On plots which mix Lines and other elements, color will only change the color of the coordinated points, not the line. Marker stroke will determine the size of our outline, in order to apply the same properties as this:
# Marker stroke width and opacity markerstrokewidth = 4, markerstrokealpha = .75, # Marker stroke color markerstrokecolor = :lightblue,
We do the same, but just remove the “ markerstroke.”
color = :lightblue, width = 4 alpha = .75
Line Plot with different parameter input
x = [1,2,3,4,5,6,7,8] y = [3,4,5,6,7,8,9,10] gr(bg=:whitesmoke) plot(x,y,arrow = true, linewidth = 6, color = :pink, yticks=([0,250000,500000,750000,100000]), grid = false,legend=:bottomright,label="Sample", title = "Price per square footage in NYC", xlabel = "Square Footage", ylabel = "Sale Price", fontfamily = :Courier,xtickfontsize=4,ytickfontsize=4, ytickfont=:Courier,xtickfont = :Courier,titlefontsize=10 )
New parts:
- Arrow
Arrow is a boolean, and simply puts a small triangle on the end of your line.
- linewidth
Integer that determines the width of the line.
- Background color
We just use the gr() function and put the following into the parameters:
gr(bg=:whitesmoke)
Now we will go about scattering, and plotting a line together.
gr(leg=false, bg=:lightblue) scatter(x,y,smooth = true, title = "NYC Housing Pricing",arrow = true, markershape = :hexagon, markersize = 3, markeralpha = 0.6, markercolor = :blue, markerstrokewidth = 1, markerstrokealpha = 0.0, markerstrokecolor = :black, linealpha = 0.5, linewidth = 5, linecolor = :orange, textcolor = :white, xlabel = "Area",ylabel = "Pricing", fontfamily = :Courier,grid = false)
New Parts
- textcolor
Changes the color of the x-ticks, and the y-ticks.
- linealpha
- linewidth
- linecolor
As I explained, lines have separate parameters when used in conjunction with other plots. This seg-ways into “ linealpha, width, and color.” This will change the properties of our line rather than our marker, so we can change our points and our line independently.
- markershape
Of course, this changes the shape of the points that we plot, here we use a hexagonal shape.
Basic Layouts
Layouts are also frequently an integral part of any graphing library.
gr(leg=false, bg=:black)
l = @layout [ a{0.3w} [grid(3,3)
b{0.2h} ]]
plot(
rand(200,11),
layout = l, legend = false, seriestype = [:bar :scatter :path], textcolor = :white,
markercolor = :green, linecolor = :orange, markerstrokewidth = 0,yticks = [.5,1],
xticks = [0,100,200]
)
Step one is to create our layout, which is actually a called constructor. We do this using:
layout = @layout [[parameters]]
So now, let’s break down what each piece of that legend part means, we will start with:
l = @layout [ a{0.3w} [grid(1,1)
b{0.5h}]]
The a portion before isn’t all that important, just the local accessor for the constructor; you can put whatever you want into here. All that {0.3w} does is determine the width of the visualization.
l = @layout [ a{0.3w} [grid(1,1)
b{0.5h}]]
As for this last portion, it creates a grid, which in the demonstration above is 3 x 3, and visualizations will be repeated into these if the list is too small.
New Parts
- seriestype
This will set our ordered array of model types to apply to our layout.
- layout
This sets the layout to our visualization.
- legend
Legend is a boolean to remove the legend pasted over-top our visualization.
Basic Animation
That’s right, GR also supports animation. Animation is as easy as creating a for loop and adding :
@gif
To the beginning of it.
p = plot([sin, cos], zeros(0), leg=false,linecolor=:white)
anim = Animation()
@gif for x = range(0, stop=10π, length=100)
push!(p, x, Float64[sin(x), cos(x)])
frame(anim)
end
GR is a great tool
For increasing your workflow. The GR back-end makes a lot of graphing buttery smooth, and very easy. I plan on talking more about GR, and some of the other back-ends associated with Julia’s Plots.jl, but for now, GR is fast and suites nicely, especially for its speed and ease of use.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK