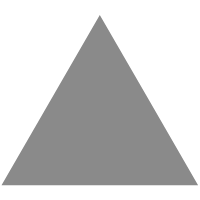
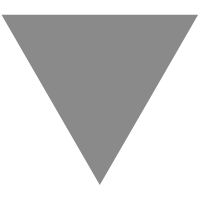
6 Ways to Make HTTP Requests with Node.js
source link: https://www.tuicool.com/articles/MZfYfya
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Introduction
Making HTTP requests is one of the core skills in software development. And Node.js provides many easy and efficient ways to achieve this.
In this article, we will go over 6 different ways to make a HTTP
request in Node.js. And we will use test data from JSON Placeholder to test the HTTP
requests.
To get the code snippets in this article to work, you need to have Node.js installed on your machine .
If you need one, we created a guide on installing Node.js .
Table of Contents
1. HTTP - Node.js Standard Library Module
The first method we'll go over is the built-in Node.js HTTP
module. This one comes bundled with Node.js when you install it, so you don't need to install any additional dependencies. But the downside is this module isn't as user-friendly compared to other methods.
The code below will send a GET
request to the JSON Placeholder REST API and return data.
const https = require ( "https" )
https . get ( "https://jsonplaceholder.typicode.com/todos/1" , function (response) {
response . setEncoding ( "utf8" )
response . on ( "data" , console . log )
response . on ( "error" , console . error )
}). on ( "error" , console . error )
Compared to the other methods in this article, it may take more effort to get the data you're after. But the upside with using this module is that you won't have to add any additional dependencies to your project.
For more information on the Node.js HTTP module or additional use cases and methods, head on over to the Node.js documentation website .
2. Axios
Axios is a promise-based HTTP
client for both the browser and Node.js. This package could be a good choice if you are making requests and need to handle them in a chain of events.
To install the npm
package:
$ npm install axios
The code below will accomplish the same goal as before, sending a GET
request to the JSON Placeholder REST API and return data.
const axios = require ( "axios" )
axios. get ( " https://jsonplaceholder.typicode.com/todos/1 " )
. then ( function (response) {
console . log ( response.data )
})
. catch ( function (error) {
console . log (error)
})
Making requests where callbacks or async behavior is involved can be an unpleasant experience. Axios aims to tackle that problem by making those things easier to deal with.
For more information on the Axios package or for additional use cases and methods, check out their Github page .
Advertisement

Get $50 in Free Credit for 30 Days!
Try the simplest cloud server platform for developers & teams.
3. Node-Fetch
Node-Fetch is a light-weight module that brings the browser window.fetch
method to Node.js.
To install the npm
package:
$ npm install node-fetch
The code below will accomplish the same goal as before, sending a GET
request to the JSON Placeholder REST API and return data.
const fetch = require ( "node-fetch" )
fetch ( " https://jsonplaceholder.typicode.com/todos/1 " )
. then ( function (response) {
return response . json ()
}). then ( function (data) {
console . log (data)
}). catch ( function (error) {
console . log (error)
})
For more information on the Node-Fetch package or for additional use cases and methods, check out their Github page .
4. Request-Promise
Request-Promise is a simplified version of the HTTP Request client with Promise support added. Powered by Bluebird . In short, Request Promise adds Bluebird powered .then(...)
method to the Request call objects.
Let's see one in action!
To install the npm
package:
$ npm install request
$ npm install request-promise
Request
is a peer-dependency, so it must be installed separately.
The code below will accomplish the same goal as before, sending a GET
request to the JSON Placeholder REST API and return data.
const requestPromise = require ( "request-promise" )
requestPromise ({uri: " https://jsonplaceholder.typicode.com/todos/1 " , headers: { "User-Agent" : "Request-Promise" }, json: true })
.then(function(response) {
console.log(response)
})
.catch(function(error) {
console.log(error)
})
For more information on the Request-Promise package or for additional use cases and methods, check out their Github page .
5. Got
Got is a human-friendly and powerful HTTP
request package. It was created to provide a less bloated version of the Request
package.
To install the npm
package:
$ npm install got
The code below will accomplish the same goal as before, sending a GET
request to the JSON Placeholder REST API and return data.
const got = require ( "got" )
got ( " https://jsonplaceholder.typicode.com/todos/1 " , { json: true })
. then ( function (response) {
console . log ( response.body )
})
. catch ( function (error) {
console . log (error)
})
For more information on the Got package or for additional use cases and methods, check out their Github page .
6. Simple-Get
Simple-Get is a package that provides a light wrapper on top of the Node.js HTTP module . And does so in less than 100 lines of code.
To install the npm
package:
$ npm install simple-get
The code below will accomplish the same goal as before, sending a GET
request to the JSON Placeholder REST API and return data.
const simpleGet = require ( "request-promise" )
simpleGet ( " https://jsonplaceholder.typicode.com/todos/1 " , function (error, response) {
if (error) {
console . log (error)
} else {
response . pipe ( process . stdout )
}
})
For more information on the Simple-Get package or for additional use cases and methods, check out their Github page .
Conclusion
As you can see from this article, there are many ways to make a HTTP
request in Node.js! And this article doesn't even cover all the solutions that are out there.
The code written in this tutorial can be found on our Github page .
Did we miss any ways that you like to use? Let us know in the comments!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK