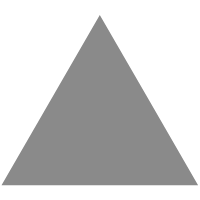
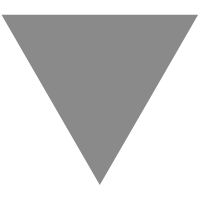
Hexagon
source link: https://hexagonkt.com
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The Hexagon Toolkit provides several libraries to build server applications. These libraries provide single standalone features1 and are referred to as "Ports".
The main ports are:
- The HTTP server: supports HTTPS, HTTP/2, mutual TLS, static files (serve and upload), forms processing, cookies, sessions, CORS and more.
- The HTTP client: which supports mutual TLS, HTTP/2 and cookies among other features.
Each of these features or ports may have different implementations called "Adapters".
Hexagon is designed to fit in applications that conform to the Hexagonal Architecture (also called Clean Architecture or Ports and Adapters Architecture). Its design principles also fit into this architecture.
Hello World¶
Simple Hello World HTTP example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
You can check the code examples and demo projects for more complex use cases.
Features¶
Hexagon's goals and design principles:
-
Put you in Charge: There is no code generation, no runtime annotation processing, no classpath based logic, and no implicit behaviour. You control your tools, not the other way around.
-
Modular: Each feature (Port) or adapter is isolated in its own module. Use only the modules you need without carrying unneeded dependencies.
-
Pluggable Adapters: Every Port may have many implementations (Adapters) using different technologies. You can swap adapters without changing the application code.
-
Batteries Included: It contains all the required pieces to make production-grade applications: settings management, serialization, dependency injection2 and build helpers.
-
Kotlin First: Take full advantage of Kotlin instead of just calling Java code from Kotlin. The library is coded in Kotlin for coding with Kotlin. No strings attached to Java (as a Language).
-
Properly Tested: The project's coverage is checked in every Pull Request. It is also stress-tested at TechEmpower Frameworks Benchmark.
Architecture¶
How Hexagon fits in your architecture in a picture.
Using this toolkit won't make your application compliant with Hexagonal Architecture (by its nature, no tool can do that), you have to provide a layer of abstraction by yourself.
Ports¶
Ports with their provided implementations (Adapters).
Comments
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK