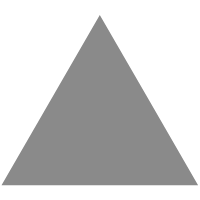
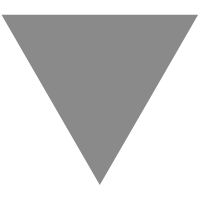
实例探索进程信号处理-优雅退出 | 逝水无痕
source link: http://guojianxiang.com/posts/2019-06-01-ProcessMgr_SigQuit.html?
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
实例探索进程信号处理-优雅退出
应用程序在处理工作的过程中通常会申请很多系统资源,通常我们希望在应用程序结束前提前释放资源,也就是优雅退出。这时候SIG信号处理就派上用场了。
之前使用Golang的时候通常会保证服务是优雅退出的,会保证正在运行的Goroutine都stop之后,主Goroutine才退出。近期在做PHP相关项目时,会启动一些常驻脚本执行任务,于是想在脚本中增加优雅退出机制。
SIG 列表
在Linux终端下我们执行 kill -l
可以看到POSIX中定义的信号列表。

但是在linux系统中,SIGKILL(9号信号)和 SIGSTOP (19号信号)这两个信号是无法被我们自己捕获和处理的,SIGKILL总是会结束进程运行,SIGSTOP总是能暂停进程。
Golang中的信号处理
在Golang中我们是使用os/signal包来处理信号的。一个简单的实例如下:
1 | package main |
PHP中的信号处理
在PHP中提供了pcntl扩展以及posix扩展可以让我们来操作信号。
1 | pcntl_signal_dispatch — 调用等待信号的处理器 |
方案一 ticks
在官方文档里是这样写的:As of PHP 4.3.0 PCNTL uses ticks as the signal handle callback mechanism, which is much faster than the previous mechanism. This change follows the same semantics as using "user ticks". You must use the declare() statement to specify the locations in your program where callbacks are allowed to occur for the signal handler to function properly (as used in the example below).
意思是在4.3.0之后版本PCNTL使用ticks捕获触发信号处理函数。
官方的pcntl_signal性能比较差,主要是PHP的函数无法直接注册到操作系统信号设置中,所以在老版本的PHP里pcntl信号需要依赖tick机制来完成。
pcntl_signal的实现原理是,维护一个信号队列,有信号后会Push进入队列,然后在ticks回调函数中检查是否有信号,有的话就执行信号对应的处理函数。
ticks通过declare定义:declare(ticks = 1);
ticks=1表示每执行1行PHP低级代码就回调此函数。实际过程中大部分运行时间是没有信号的,所以这样就会导致每次语句都会不必要的执行检查,导致系统性能有所下降。而且当有语句进行阻塞无法调用时,进程会一直hang住,接收不到任何信号。
方案二 pcntl_signal_dispatch
在PHP5.3.0版本以后增加了pcntl_signal_dispatch
函数,我们可以在需要等待信号的地方调用该函数,来执行信号回调。这样我们既降低了代码执行频率也实现了类实时的信号通知。
示例如下:
cliBase.php
1 | <?php |
logic.php
1 | <?php |
执行logic.php
后,在终端发送 kill -2 pid 可以看到我们能捕捉到信号并优雅退出了。
方案三 pcntl_async_signals
在PHP>=7.1.0版本后引入了pcntl_async_signals
可以代替ticks。
1 | <?php |
综上,在PHP中使用信号处理可以按照版本号支持情况选择更好的方案二、三。
通常信号处理也还用在多进程模型下主进程与子进程之间的通讯等。
[参考资料]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK