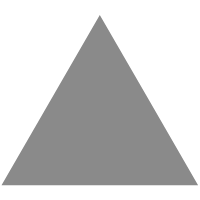
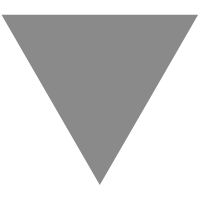
A Quick Full-Screen Hover Effect with CSS and JavaScript
source link: https://www.tuicool.com/articles/UZvq2iz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In today’s quick tutorial we’ll learn how to build a useful full-screen background hover effect.
As the saying goes: “a picture is worth a thousand words”, so let’s have a look at what we’re going to create:
In a real world scenario you might use this kind of effect as a quick view modal for your products, portfolio items, photos, and so on. Let’s get started!
1. Begin With the Page Markup
We’ll start by marking up just two elements:
<div class="bg"></div> <div class="container">...</div>
The .bg
element will be an empty element.
The .container
element will hold the page contents. Inside it, we’ll put four links. We’ll associate each link with an image via the custom data-bg
attribute like this:
<a data-bg="IMG_SRC">...</a>
2. Add the CSS
Let’s continue with some basic CSS.
We’ll give the .container
a maximum width and horizontally align its contents:
.container { max-width: 800px; margin: 0 auto; padding: 0 10px; }
For stylistic reasons, instead of the default text-decoration: underline
property value, we’ll use a border-bottom
to underline the text links:
/*CUSTOM VARIABLES HERE*/ .container a { position: relative; border-bottom: 2px dashed var(--red); transition: all 0.2s; }
Coming up next, we’ll define the ::before
pseudo-element for the links. This will initially be hidden. It will appear with a “shutter out” horizontal effect, each time we hover over its parent link.
Its initial styles:
/*CUSTOM VARIABLES HERE*/ .container a::before { content: ''; position: absolute; top: 50%; left: 50%; width: 100%; height: 100%; min-height: 30px; transform: translate(-50%, -50%) scaleX(0); padding: 10px; z-index: -1; transform-origin: 50%; background: var(--white); }
The .bg
element will be also visually hidden by default ( opacity: 0
). Plus, it will be absolutely positioned and cover the full width and height of its container (in this case the body
).
Here are its initial styles:
.bg { position: absolute; top: 0; left: 0; right: 0; bottom: 0; opacity: 0; background-repeat: no-repeat; background-position: center; background-size: cover; transition: all 0.3s ease-out; }
3. Adding the JavaScript
Preload the Images
With the HTML and CSS in place, the next necessary step is to preload the data-bg
images. We’ll do that with just a few lines of code:
const links = document.querySelectorAll(".container a"); for(const link of links) { const img = new Image(); img.src = link.dataset.bg; }
This code iterates through all the page links, grabs the value of each one’s data-bg
attribute, and creates a few img
instances by using the Image()
constructor.
So now if we reload the demo page and look at the Network tab of our browser inspector, we’ll see that all images have been downloaded/preloaded:

This is a vital action for preventing the white flash that would occur the first time we hover over a link. Without preloading, at that point the browser won’t yet have downloaded the image and it might take a while until the image appears.
If you want to test this behavior, first comment the aforementioned code, then open the Network tab and reload the page.
Toggle the Images
Each time we hover over a link, we should do the following:
- Grab its related image which is stored in the
data-bg
attribute. - Set that image as the background image of the
.bg
element. - Add the
bg-show
class to thebody
. This class will ensure two things. Firstly, that the.bg
will appear with a fade-in effect and sit on top of every element, apart from the active link. Secondly, that the pseudo-element of the active link will appear with a shutter out horizontal effect.
On the other hand, every time the cursor leaves a link, we’ll remove the bg-show
class from the body
and the .bg
will disappear.
Here’s the corresponding JavaScript code:
const links = document.querySelectorAll(".container a"); const bg = document.querySelector(".bg"); const showClass = "bg-show"; for(const link of links) { link.addEventListener("mouseenter", function() { bg.style.backgroundImage = `url(${this.dataset.bg})`; document.body.classList.add(showClass); }); link.addEventListener("mouseleave", () => { document.body.classList.remove(showClass); }); }
And the related styles:
/*CUSTOM VARIABLES HERE*/ .bg-show .bg { z-index: 1; opacity: 1; } .bg-show a:hover { z-index: 2; border-bottom-color: transparent; color: var(--red); } .bg-show .container a:hover::before { transform: translate(-50%, -50%) scaleX(1); transition: transform 0.2s 0.1s ease-out; }
Conclusion
That’s all folks! With just a few lines of code we managed to create an interesting full-screen background hover effect.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK