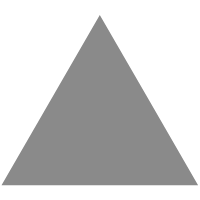
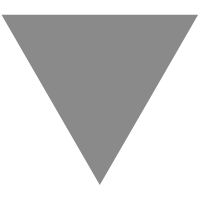
Java SimpleDateFormat Is Not Simple
source link: https://www.tuicool.com/articles/qIfuYvI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Formatting and parsing dates is a daily (painful) task. Every day, it gives us another headache.
A common way to format and parse dates in Java is using SimpleDateFormat
. Here is a common class we can use.
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; public final class DateUtils { public static final SimpleDateFormat SIMPLE_DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd"); private DateUtils() {} public static Date parse(String target) { try { return SIMPLE_DATE_FORMAT.parse(target); } catch (ParseException e) { e.printStackTrace(); } return null; } public static String format(Date target) { return SIMPLE_DATE_FORMAT.format(target); } }
Do you think this is working as we expect? Let's try it.
private static void testSimpleDateFormatInSingleThread() { final String source = "2019-01-11"; System.out.println(DateUtils.parse(source)); } // Fri Jan 11 00:00:00 IST 2019
Yes, it worked. Let's try it with more threads.
private static void testSimpleDateFormatWithThreads() { ExecutorService executorService = Executors.newFixedThreadPool(10); final String source = "2019-01-11"; System.out.println(":: parsing date string ::"); IntStream.rangeClosed(0, 20) .forEach((i) -> executorService.submit(() -> System.out.println(DateUtils.parse(source)))); executorService.shutdown(); }
Here is the result I got.
:: parsing date string ::
... omitted
Fri Jan 11 00:00:00 IST 2019Sat Jul 11 00:00:00 IST 2111Fri Jan 11 00:00:00 IST 2019... omittedYes, it worked. Let's try it with more threads.
Interesting result, isn't it? This is a common mistake most of us have made when formatting dates in Java. Why? because we are not aware of thread safety. Here is what the Java doc says about SimpleDateFormat
:
"Date formats are not synchronized.
It is recommended to create separate format instances for each thread.
If multiple threads access a format concurrently, it must be synchronized
externally."
Tip: When we use instance variables, we should always check whether it is a thread safe class or not.
As the doc says, we can solve this by having separate instances for each thread. What if we want to share it? What are the solutions?
Solution 1: ThreadLocal
This can be solved by using a ThreadLocal
variable. ThreadLocal
's get()
method will give us the correct value for the current thread.
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; public final class DateUtilsThreadLocal { public static final ThreadLocal SIMPLE_DATE_FORMAT = ThreadLocal.withInitial(() -> new SimpleDateFormat("yyyy-MM-dd")); private DateUtilsThreadLocal() {} public static Date parse(String target) { try { return SIMPLE_DATE_FORMAT.get().parse(target); } catch (ParseException e) { e.printStackTrace(); } return null; } public static String format(Date target) { return SIMPLE_DATE_FORMAT.get().format(target); } }
Solution 2: Java 8 Thread-Safe Date-Time API
Java 8 came with a new date-time API. We have a better alternative to SimpleDateFormat
with less trouble. If we really need to stick with SimpleDateFormat
,
we can go ahead with ThreadLocal
. But when we have a better option, we should consider using it.
Java 8 comes with several thread-safe date classes.
And here is what the Java docs say:
"This class is immutable and thread-safe."
It is worth studying more about these classes, including DateTimeFormatter , OffsetDateTime , ZonedDateTime , LocalDateTime , LocalDate , and LocalTime .
Our solution:
import java.time.LocalDate; import java.time.format.DateTimeFormatter; public class DateUtilsJava8 { public static final DateTimeFormatter DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd"); private DateUtilsJava8() {} public static LocalDate parse(String target) { return LocalDate.parse(target, DATE_TIME_FORMATTER); } public static String format(LocalDate target) { return target.format(DATE_TIME_FORMATTER); } }
Conclusion
Java 8 solution uses an immutable class, which is a good practice for solving multi-threading problems. Immutable classes are thread-safe by nature, so use them whenever possible.
Happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK