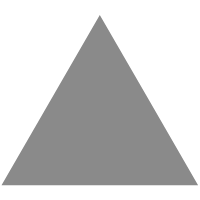
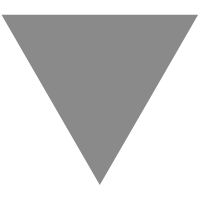
转发和重定向又是什么“垃圾”——教你再分类 - 泰斗贤若如
source link: https://www.cnblogs.com/zyx110/p/11229865.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
转发和重定向详解
转发与重定向的区别
前言:之前写了几篇JSP的博客,现在又回过头来看Servlet,温故而知新,再回顾回顾,总会有收获的。以前学习Servlet感觉内容很多,现在看的时候,其实也没多少东西,只需知道Servlet的生命周期,Servlet的实现方式,ServletContext作用域,接收和响应,转发和重定向,我觉得差不多够用了。当然,要是细究的话也不止这些,我针对的是新手。
转发与重定向简介
转发和重定向都是实现页面跳转,也就是说,当我们访问一个Servlet 的时候,Servlet帮我们跳转到另一个界面。
转发与重定向的区别
实现转发调用的是HttpServletRequest对象中的方法
实现重定向调用的是HttpServletResponse对象中的方法
转发时浏览器中的url地址不会发生改变
重定向时浏览器中的url地址会发生改变
转发时浏览器只请求一次服务器
重定向时浏览器请求两次服务器
转发能使用request带数据到跳转的页面
重定向能使用ServletContext带数据到跳转的页面
代码演示转发和重定向
package servlet; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet ( "/login" ) public class ServletDemo extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //获取表单提交过来的数据 //getParameter()方法可以获取请求的参数信息 String name = req.getParameter( "name" ); String password = req.getParameter( "password" ); //打印获取到的参数信息 System.out.println( "name:" +name); System.out.println( "password:" +password); //如果name=admin,password=123,则跳转到succee.jsp,否则跳转到fail.jsp if ( "admin" .equals(name)&& "123" .equals(password)){ //通过转发实现跳转 req.getRequestDispatcher( "/success.jsp" ).forward(req,resp); } else { //通过重定向实现跳转 resp.sendRedirect( "/fail.jsp" ); } } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } } |
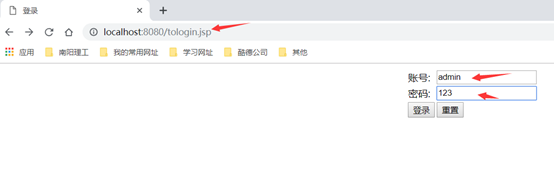
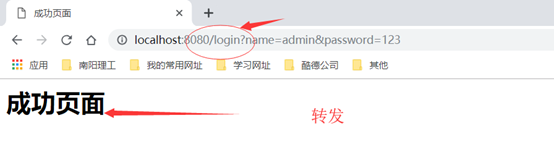
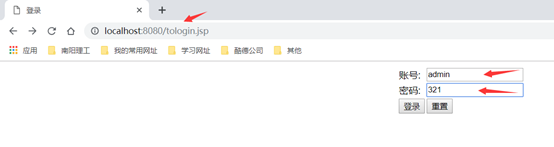
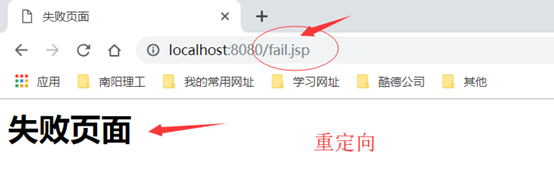
JSP代码
<%@ page contentType= "text/html;charset=UTF-8" language= "java" %> <html> <head> <title>登录</title> </head> <body> <form action= "/login" > <table align= "center" > <tr> <td>账号:</td> <td><input type= "text" name= "name" ></td> </tr> <tr> <td>密码:</td> <td><input type= "text" name= "password" ></td> </tr> <tr> <td><input type= "submit" value= "登录" ></td> <td><input type= "reset" value= "重置" ></td> </tr> </table> </form> </body> </html> |
转发和重定向如何带数据到某个页面
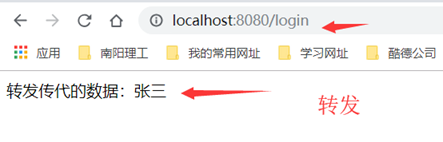
package servlet; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet ( "/login" ) public class ServletDemo extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //通过转发带数据 req.setAttribute( "name" , "张三" ); req.getRequestDispatcher( "/send.jsp" ).forward(req,resp); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } } |
send.jsp
<%@ page contentType= "text/html;charset=UTF-8" language= "java" %> <html> <head> <title>转发和重定向传代数据练习</title> </head> <body> <% //1、接收转发传代的数据 String name = (String) request.getAttribute( "name" ); out.println( "转发传代的数据:" +name); %> </body> </html> |
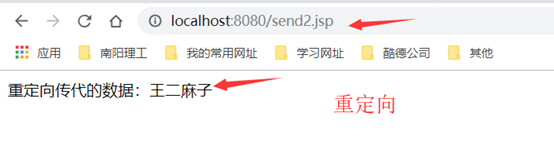
package servlet; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet ( "/login" ) public class ServletDemo extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //通过重定向带数据 ServletContext servletContext = this .getServletContext(); servletContext.setAttribute( "name" , "王二麻子" ); resp.sendRedirect( "/send2.jsp" ); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } } |
send2.jsp
<%@ page contentType= "text/html;charset=UTF-8" language= "java" %> <html> <head> <title>转发和重定向传代数据练习</title> </head> <body> <% //1、接收重定向传代的数据 String name1 = (String)application.getAttribute( "name" ); out.println( "重定向传代的数据:" +name1); %> </body> </html> |
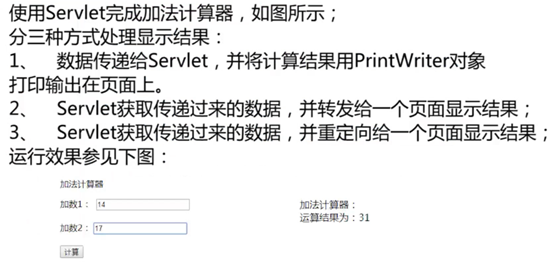
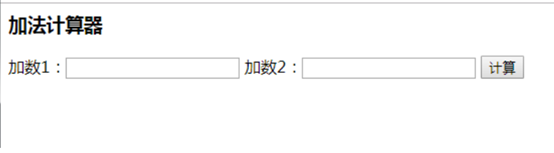
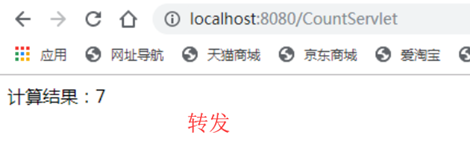
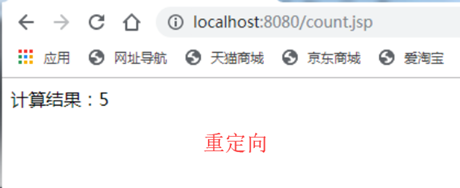
index.jsp
<%@ page contentType= "text/html;charset=UTF-8" language= "java" %> <html> <head> <title>Title</title> </head> <body> <form action= "CountServlet" method= "post" > <h3>加法计算器</h3> 加数 1 :<input type= "number" name= "one" > 加数 2 :<input type= "number" name= "two" > <input type= "submit" value= "计算" > </form> </body> </html> |
count.jsp
<%@ page contentType= "text/html;charset=UTF-8" language= "java" %> <html> <head> <title>Title</title> </head> <body> 计算结果:<%=request.getAttribute( "count" )%> <!--计算结果:<%=application.getAttribute( "count" )%>--> </body> </html> |
Servlet
package servlet; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet ( "/CountServlet" ) public class CountServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String one=request.getParameter( "one" ); int o=Integer.parseInt(one); //强制转换,将String类型的数据转换成int类型 String two=request.getParameter( "two" ); int t=Integer.parseInt(two); //强制转换,将String类型的数据转换成int类型 System.out.println(one+ " " +two); int c=o+t; String co=String.valueOf(c); //将int类型的数据转换成String类型 //转发,可以携带数据 request.setAttribute( "count" ,co); request.getRequestDispatcher( "count.jsp" ).forward(request,response); //用于存放数据 // ServletContext s=this.getServletContext(); // s.setAttribute("count",co); //重定向只能依靠ServletContext获取数据 // response.sendRedirect("count.jsp"); System.out.println(co); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request,response); } } |
Servlet分享到此结束,想了解更多的朋友可以看之前总结过的博客《Servlet》,和《JSP》 ,更多精彩等你来学习。
*****************************************************************************************************
我的博客园地址:https://www.cnblogs.com/zyx110/
本文已独家授权给脚本之家(jb51net)公众号独家发布
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK