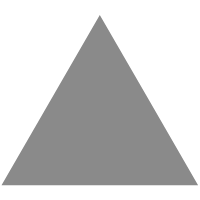
36
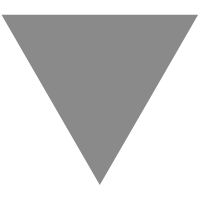
Linux System Programming by Robert Love – Reading Notes
source link: https://www.tuicool.com/articles/naUVBff
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Chapter 1 - Introduction
- processes inherit the UID and GID
- permission octal values: r=4, w=2, x=1. Order is user, group, everyone else
- functions normally just return a -1 to indicate an error
-
more details to be found in
extern int errno
in<errno.h>
-
to print
void perror(const char *str)
in<stdio.h>
- example:
if (close (fd) == −1) perror ("close");
Chapter 2 - File I/O
- Opening files:
fd = open(<path>, flags)
- create:
fd = open (file, O_WRONLY | O_CREAT | O_TRUNC, 0664);
- is identical to
fd = creat (filename, 0644);
- fd of -1 indicates error
- reading:
#include <unistd.h> ssize_t read (int fd, void *buf, size_t len);
- A call to read() can result in many possibilitie, to read all the bytes
ssize_t ret; while (len != 0 && (ret = read (fd, buf, len)) != 0) { if (ret == −1) { if (errno == EINTR) continue; perror ("read"); break; } len -= ret; buf += ret; }
open
#include <unistd.h> ssize_t write (int fd, const void *buf, size_t count);
- call fsync(int ft) to sync writes to storage
- fdatasync(int fd) does the same thing, without updated metadata, and is faster
- use the O_SYNC flag to always sync
- closing:
#include <unistd.h> int close(int fd)`
- seeking:
#include <sys/types.h> #include <unistd.h> off_t lseek (int fd, off_t pos, int origin);
- you CAN seek past the end of a file, it will be padded with zeros
- position reads and writes avoid the race condtion associated with seeking and then reading
#define _XOPEN_SOURCE 500 #include <unistd.h> ssize_t pread (int fd, void *buf, size_t count, off_t pos); ssize_t pwrite (int fd, const void *buf, size_t count, off_t pos);
Multiplexed IO
-
poll()
is easier to use thanselect()
- both wait on a set of open file descriptors and return when any are available
- poll example
#include <stdio.h> #include <poll.h> #include <unistd.h> int main(int argc, char **argv) { struct pollfd fds[2]; fds[0].fd = STDIN_FILENO; fds[0].events = POLLIN; fds[1].fd = STDOUT_FILENO; fds[1].events = POLLOUT; int err = poll(fds, (nfds_t) 2, 0); if (err == -1) { perror("poll"); return -1; } if (fds[0].revents & POLLIN) { printf("STDIN ready\n"); } if (fds[1].revents & POLLOUT) { printf("STDOUT ready\n"); } return 0; }
- The VFS provides a unified blocks and inodes based interface to all filesystems
- The page cache holds retrieved info including readaheads
Chapter 3 - Buffered I/O
- Usually more efficient to read in multiples of 4096 or 8192 bytes because of block alignment
- User space buffered IO can increase performance even more
- Write to a buffer, which is written in a single operation
- The read requests are served from the buffer
- The end result is fewer system calls for larger amounts of data, all aligned on block boundaries.
-
Provided by
stdio
- StardardI/O routines act on file pointers, not fds
-
FILE
type defined instdio.h
FILE * fopen (const char *path, const char *mode); FILE * fdopen (int fd, const char *mode);
- modes: r, w, r+ (read+write), w+ (read, write, truncates), a+(rw in append mode)
- Closing the stream will close the file descriptor as well.
int fclose (FILE *stream); int fcloseall (void); // Linux specific
- reading
// read a char int fgetc (FILE *stream); // put it back int ungetc (int c, FILE *stream); // read a line // reads one char less than size and puts a
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK